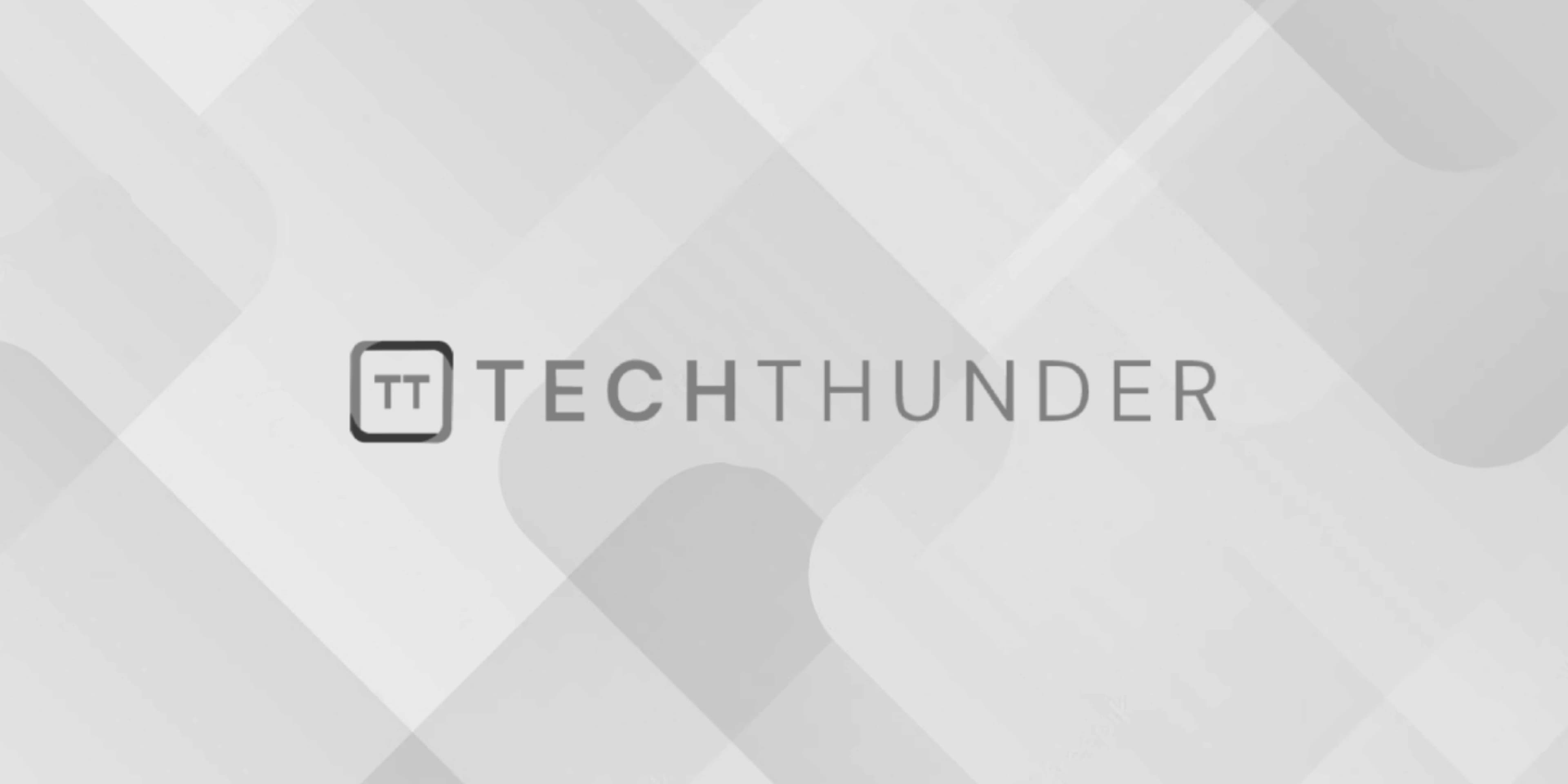
194 views
C Macros
The macros are a way to define reusable pieces of code or constants that are expanded by the preprocessor before actual compilation. Macros are typically defined using the #define
preprocessor directive and can be used to make code more readable, maintainable, and efficient.
Here are some common uses of macros in C:
Constant Macros:
- Macros are often used to define constants that are used throughout the program. These constants are replaced with their values during preprocessing, which can improve code readability.
- Example:
C
c #define PI 3.14159265359 #define MAX_LENGTH 100
Function-like Macros:
- Macros can be defined to mimic functions, taking arguments and returning values. These macros are expanded inline and can be more efficient than actual functions for simple operations.
- Example:
C
c #define SQUARE(x) ((x) * (x))
Conditional Compilation:
- Macros are commonly used to conditionally include or exclude sections of code during compilation based on preprocessor directives like
#ifdef
,#ifndef
,#if
,#elif
, and#endif
. - Example:
C
c #define DEBUG // Enable debugging #ifdef DEBUG // Debugging code here #endif
Header Guards:
- Macros are used to prevent header files from being included multiple times in a program by using header guards.
- Example in a header file (
example.h
):
C
c #ifndef EXAMPLE_H #define EXAMPLE_H // Header contents here #endif
String Concatenation:
- Macros can concatenate strings or identifiers together.
- Example:
C
c #define CONCAT(a, b) a##b int foobar = CONCAT(foo, bar);
// This creates an integer variable named "foobar."</code>
Token Pasting:
- Macros can paste tokens together to create new identifiers or symbols.
- Example:
C
c #define DECLARE_VARIABLE(type, name) type name DECLARE_VARIABLE(int, age);
// This declares an integer variable named "age."
File Inclusion:
- Macros like
#include
are used to include header files or other source files at specific locations within your code. - Example:
C
c #include <stdio.h>
It’s important to use macros judiciously and follow best practices to ensure code maintainability and avoid common pitfalls. Macros can make code more efficient but can also lead to readability and debugging challenges if overused or misused.