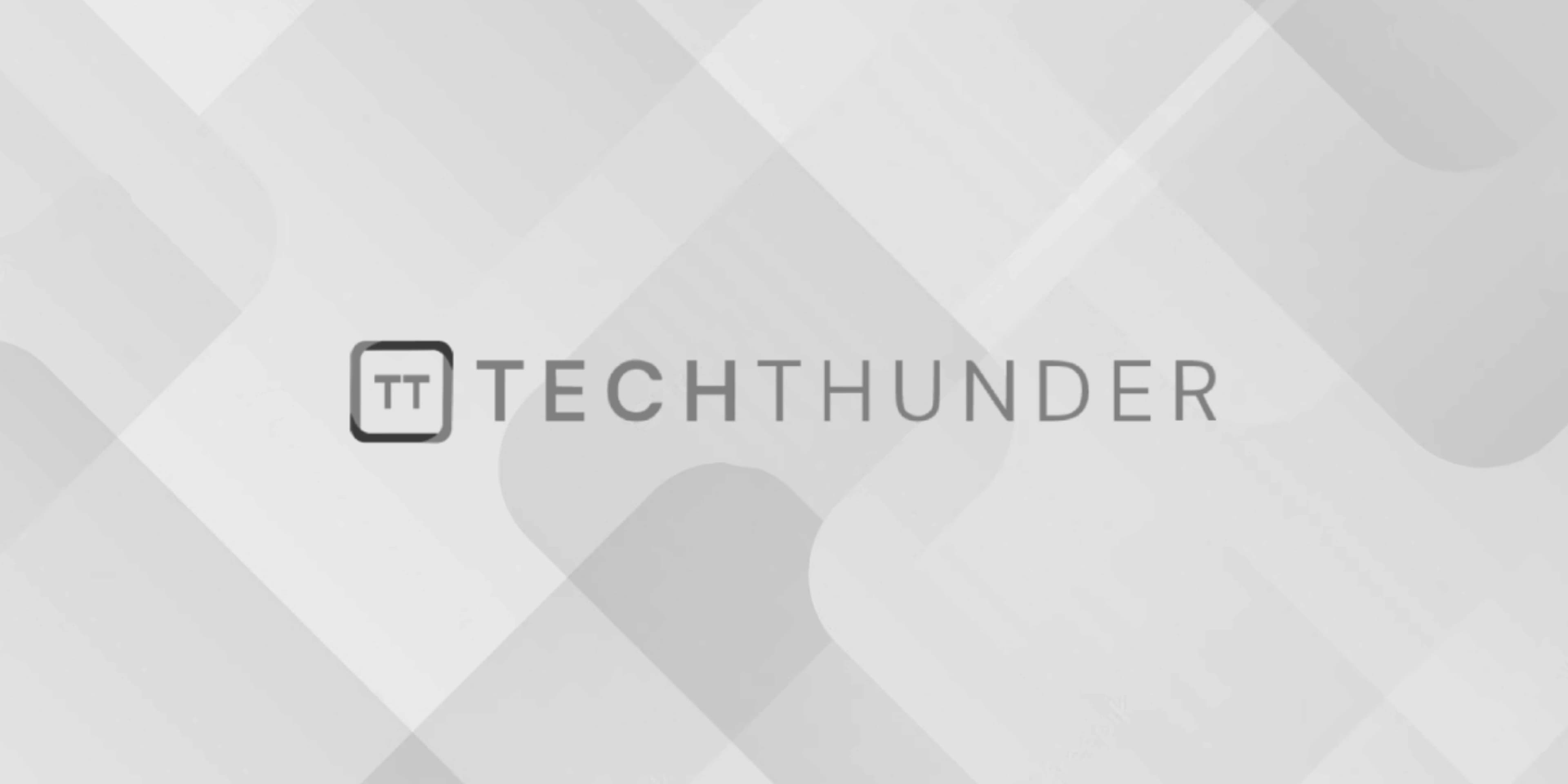
C continue
The continue
statement is used within loops to force the immediate termination of the current iteration (or “cycle”) of the loop and move on to the next iteration. When continue
is encountered, the remaining code within the current iteration of the loop is skipped, and the loop’s condition is re-evaluated to determine whether to continue with the next iteration.
The syntax of the continue
statement is straightforward:
continue;
Key points to understand about continue
:
- Usage in Loops:
- The
continue
statement is primarily used within loops, such asfor
,while
, anddo-while
loops. - When
continue
is encountered, the program jumps to the loop’s increment or update section (e.g., the increment part of afor
loop) and then re-evaluates the loop’s condition.
- Skip Current Iteration:
continue
is used to skip the remaining code within the current iteration of the loop and proceed to the next iteration.- It does not exit the loop entirely; instead, it moves to the next iteration.
- Example with
for
Loop:
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue; // Skip iteration when i equals 3
}
printf("%d ", i);
}
In this example, when i
equals 3, the continue
statement is executed, skipping the printf
statement, and the loop proceeds to the next iteration. The output will be 1 2 4 5
.
- Example with
while
Loop:
int i = 1;
while (i <= 5) {
if (i == 3) {
i++;
continue; // Skip iteration when i equals 3
}
printf("%d ", i);
i++;
}
This while
loop accomplishes the same task as the for
loop in the previous example, but it uses the continue
statement in a different loop construct.
- Avoiding Infinite Loops:
- Be cautious when using
continue
to avoid creating infinite loops unintentionally. Ensure that the loop’s condition eventually allows the loop to terminate.
- Common Use Cases:
- Common use cases for
continue
include skipping specific iterations based on certain conditions, filtering out undesired elements in a loop, or handling exceptional cases.
The continue
statement is a useful tool for controlling the flow of execution within loops and can help you skip specific iterations when necessary. However, it should be used judiciously to maintain the correctness of your program logic.