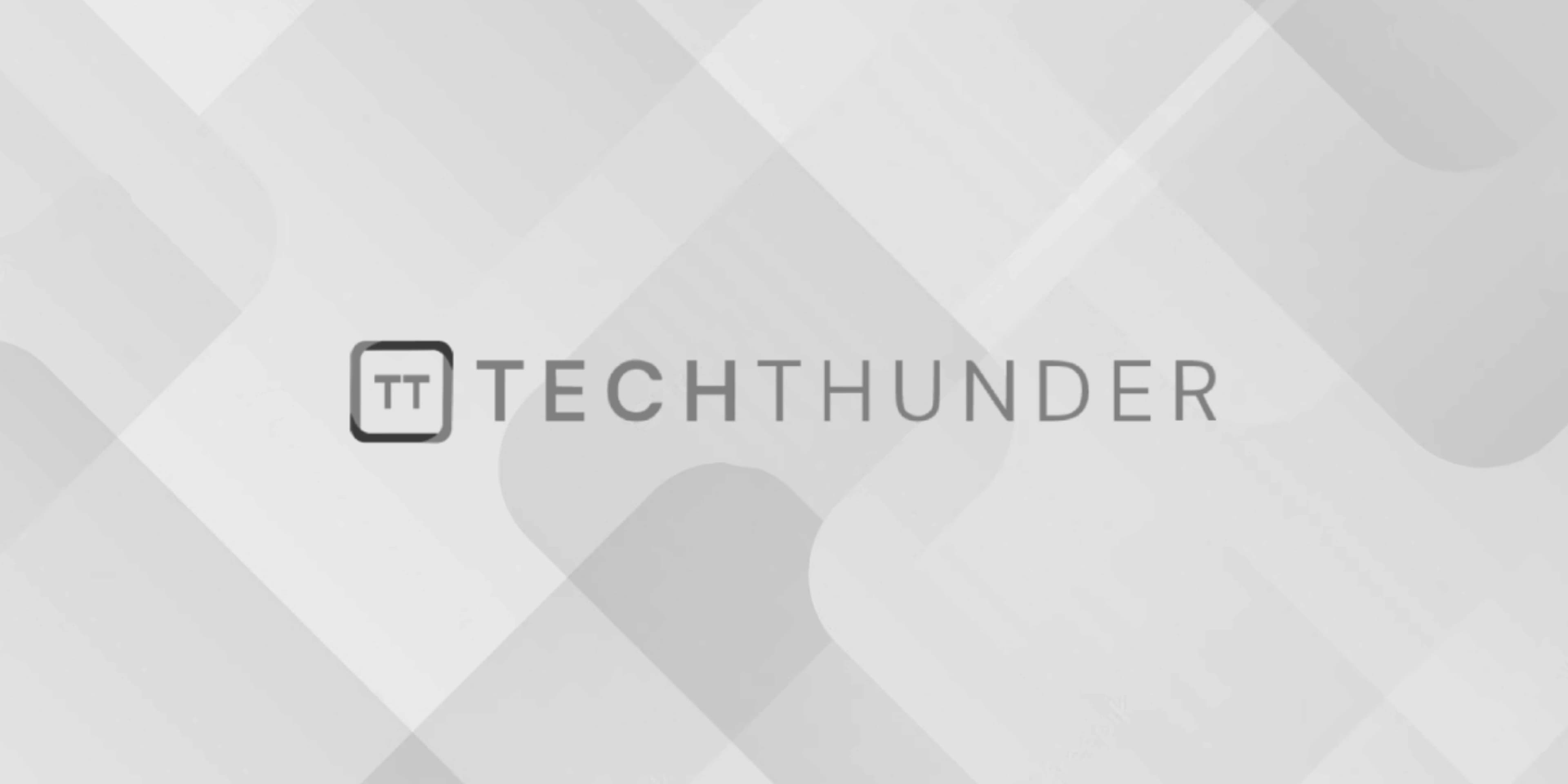
259 views
Bitwise Operator in C
The bitwise operators are used to manipulate individual bits within integer data types. These operators perform bit-level operations, which can be useful for tasks such as setting or clearing specific bits, extracting or inserting values into bit fields, and more. C provides several bitwise operators, including:
- Bitwise AND (
&
):
- The bitwise AND operator
&
compares each pair of corresponding bits from two integers and returns a new integer with bits set to 1 only if both corresponding bits are 1. - Example:
a & b
performs bitwise AND betweena
andb
.
- Bitwise OR (
|
):
- The bitwise OR operator
|
compares each pair of corresponding bits from two integers and returns a new integer with bits set to 1 if at least one corresponding bit is 1. - Example:
a | b
performs bitwise OR betweena
andb
.
- Bitwise XOR (
^
):
- The bitwise XOR (exclusive OR) operator
^
compares each pair of corresponding bits from two integers and returns a new integer with bits set to 1 if the corresponding bits are different (one is 0, and the other is 1). - Example:
a ^ b
performs bitwise XOR betweena
andb
.
- Bitwise NOT (
~
):
- The bitwise NOT operator
~
is a unary operator that inverts each bit in the operand. It turns 0s into 1s and 1s into 0s. - Example:
~a
inverts all the bits ina
.
- Left Shift (
<<
):
- The left shift operator
<<
shifts the bits of an integer to the left by a specified number of positions. It effectively multiplies the integer by 2 raised to the power of the shift amount. - Example:
a << n
shifts the bits ofa
to the left byn
positions.
- Right Shift (
>>
):
- The right shift operator
>>
shifts the bits of an integer to the right by a specified number of positions. It effectively divides the integer by 2 raised to the power of the shift amount. - Example:
a >> n
shifts the bits ofa
to the right byn
positions.
Here’s a simple example that demonstrates the use of some bitwise operators:
C
#include <stdio.h>
int main() {
int a = 5; // Binary representation: 0101
int b = 3; // Binary representation: 0011
// Bitwise AND
int result_and = a & b; // result_and = 0001 (binary) = 1 (decimal)
// Bitwise OR
int result_or = a | b; // result_or = 0111 (binary) = 7 (decimal)
// Bitwise XOR
int result_xor = a ^ b; // result_xor = 0110 (binary) = 6 (decimal)
// Bitwise NOT
int result_not_a = ~a; // result_not_a = 11111010 (binary) = -6 (decimal)
// Left Shift
int result_left_shift = a << 2; // result_left_shift = 10100 (binary) = 20 (decimal)
// Right Shift
int result_right_shift = a >> 1; // result_right_shift = 0010 (binary) = 2 (decimal)
printf("a & b = %d\n", result_and);
printf("a | b = %d\n", result_or);
printf("a ^ b = %d\n", result_xor);
printf("~a = %d\n", result_not_a);
printf("a << 2 = %d\n", result_left_shift);
printf("a >> 1 = %d\n", result_right_shift);
return 0;
}
This program demonstrates various bitwise operations on integers a
and b
and prints the results. Understanding bitwise operators is important for low-level manipulation of data and dealing with binary representations of values.