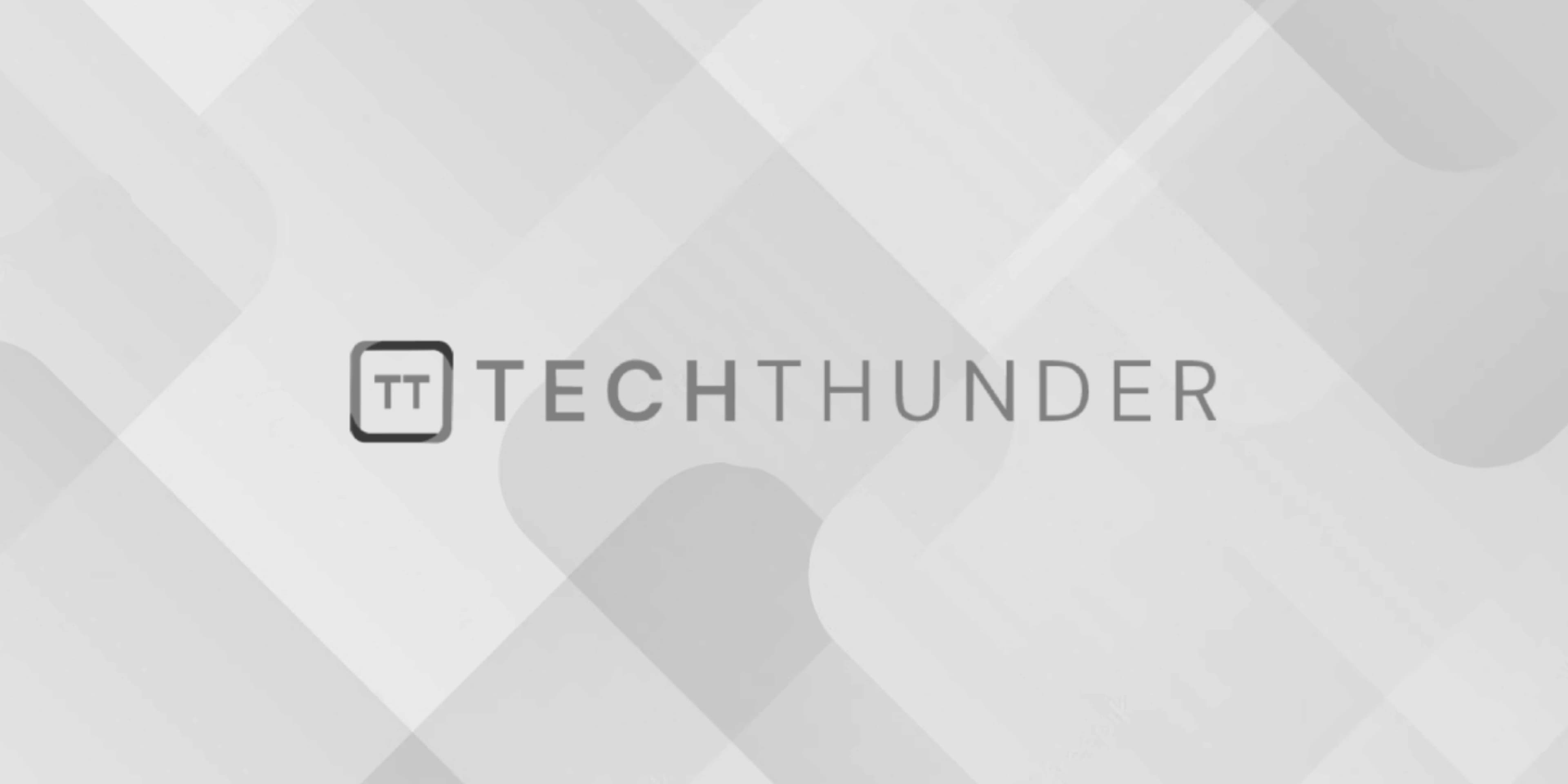
233 views
Tricky Programs in C
The tricky programs in C often involve clever or unconventional uses of C language features. Here are some examples:
- Swapping Without a Temporary Variable:
This program swaps two integers without using a temporary variable using XOR.
C
#include <stdio.h>
int main() {
int a = 5, b = 10;
printf("Before Swap: a = %d, b = %d\n", a, b);
a = a ^ b;
b = a ^ b;
a = a ^ b;
printf("After Swap: a = %d, b = %d\n", a, b);
return 0;
}
- Print “Hello, World!” Without Semicolon:
This program prints “Hello, World!” without using a semicolon in the code.
C
#include <stdio.h>
int main() {
if (printf("Hello, World!\n")) {}
return 0;
}
- Reversing a String Recursively:
This program reverses a string using recursion.
C
#include <stdio.h>
void reverseString(char *str) {
if (*str) {
reverseString(str + 1);
putchar(*str);
}
}
int main() {
char str[] = "Hello";
reverseString(str);
return 0;
}
- Printing Triangle Patterns:
This program prints various triangle patterns using nested loops.
C
#include <stdio.h>
int main() {
int n = 5;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++) {
printf("* ");
}
printf("\n");
}
return 0;
}
- Fibonacci Series Without Recursion:
This program prints the Fibonacci series without using recursion.
C
#include <stdio.h>
int main() {
int n = 10, first = 0, second = 1, next;
printf("Fibonacci Series: ");
for (int i = 0; i < n; i++) {
if (i <= 1) next = i;
else {
next = first + second;
first = second;
second = next;
}
printf("%d ", next);
}
return 0;
}
These tricky programs demonstrate creative ways to use C language features and solve problems in unconventional ways. They are often used to test your understanding of C or to explore interesting programming techniques.