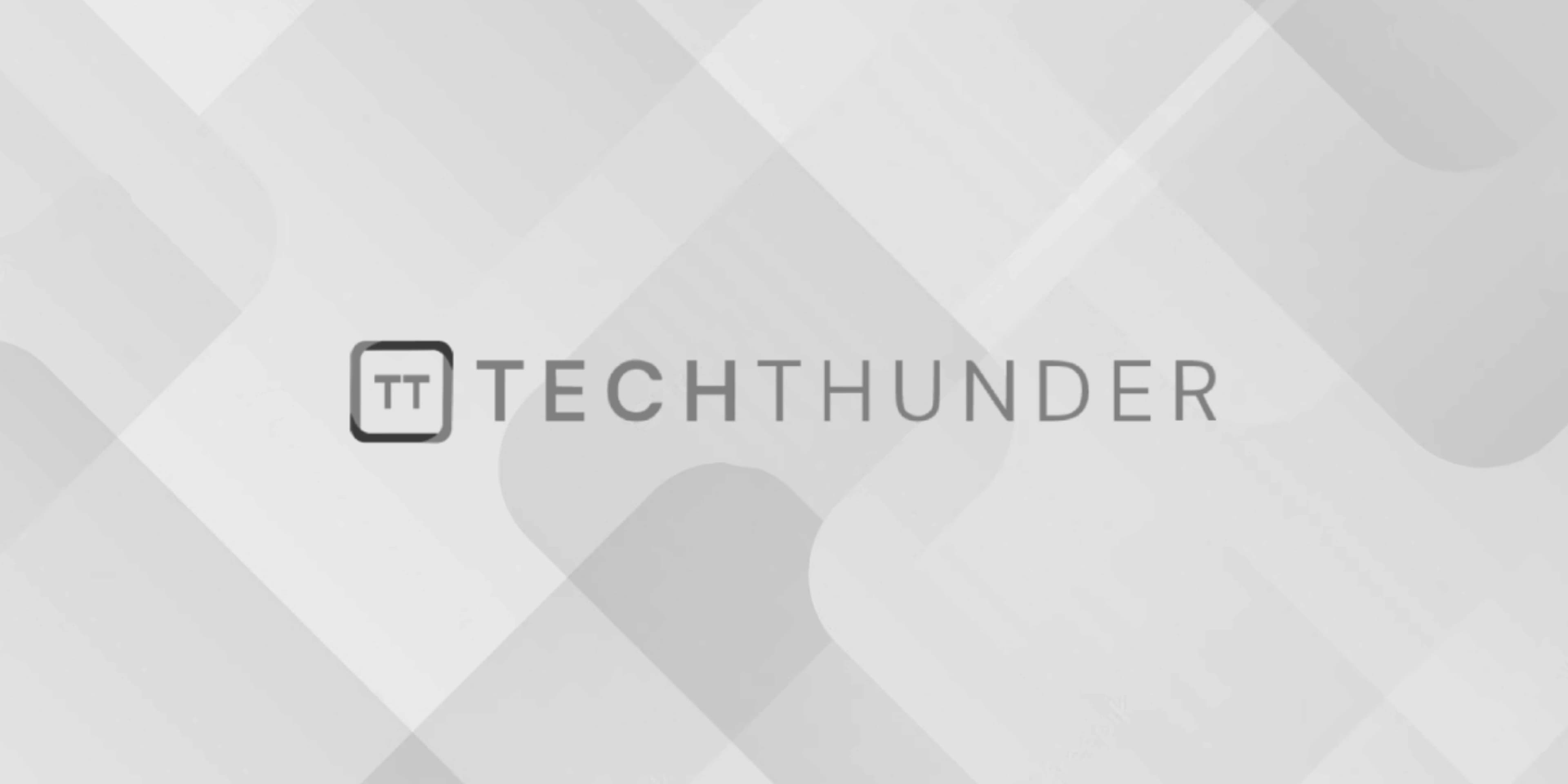
Power Function in C
There is no built-in power()
function like you might find in some other programming languages. Instead, you can use the pow()
function, which is part of the C Standard Library and is declared in the <math.h>
header. The pow()
function is used to calculate the power of a number.
Here’s the syntax of the pow()
function:
double pow(double base, double exponent);
base
: The base value.exponent
: The exponent value.
The pow()
function returns the result of raising the base
to the power of exponent
as a double
value.
Here’s an example of how to use the pow()
function:
#include <stdio.h>
#include <math.h>
int main() {
double base = 2.0;
double exponent = 3.0;
double result;
// Calculate 2^3
result = pow(base, exponent);
printf("%lf ^ %lf = %lf\n", base, exponent, result);
return 0;
}
In this example, we calculate and print the result of 2^3 using the pow()
function. The result will be 8.000000
.
Make sure to include the <math.h>
header and link against the math library (using the -lm
flag when compiling) when using the pow()
function. Additionally, be aware that the pow()
function returns a double
value, so you may need to cast the result to a different data type if needed.