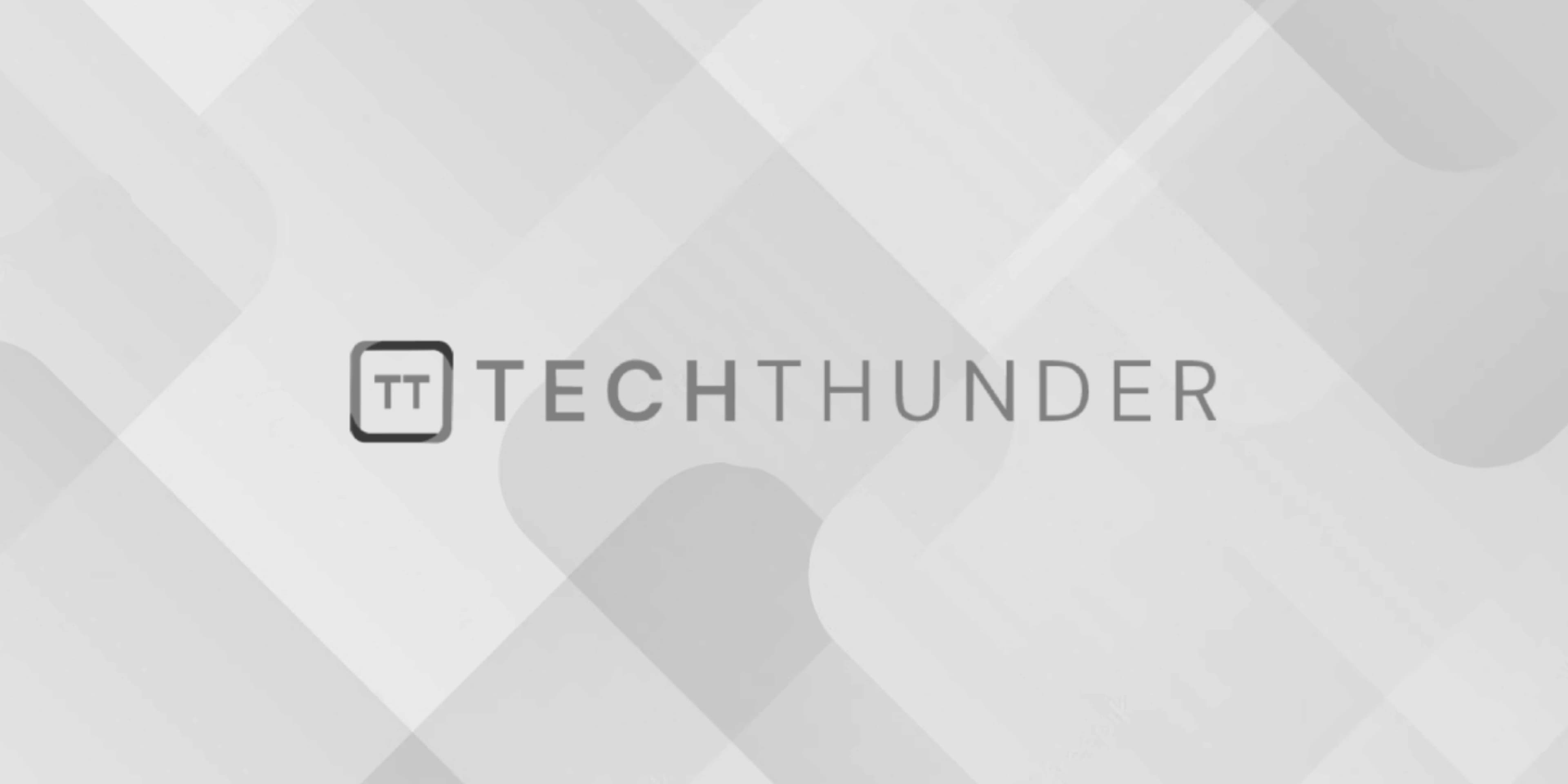
abs() function in C
The abs()
function is used to calculate the absolute value of an integer. It’s a part of the C Standard Library and is declared in the stdlib.h
header file.
The syntax of the abs()
function is as follows:
int abs(int x);
x
: The integer value for which you want to calculate the absolute value.
The abs()
function returns the absolute value of the integer passed as an argument. The absolute value of a number is its distance from zero on the number line, and it is always a non-negative value.
Here’s an example of how to use the abs()
function:
#include <stdio.h>
#include <stdlib.h>
int main() {
int num1 = -5;
int num2 = 10;
int abs_num1 = abs(num1);
int abs_num2 = abs(num2);
printf("Absolute value of %d is %d\n", num1, abs_num1);
printf("Absolute value of %d is %d\n", num2, abs_num2);
return 0;
}
In this example, we have two integers, num1
and num2
. We use the abs()
function to calculate their absolute values and then print the results. The output of this program will be:
Absolute value of -5 is 5
Absolute value of 10 is 10
Keep in mind that the abs()
function works only with integers (int
). If you need to calculate the absolute value of a floating-point number (e.g., float
or double
), you can use the fabs()
function from the math.h
header, which is specifically designed for floating-point numbers.