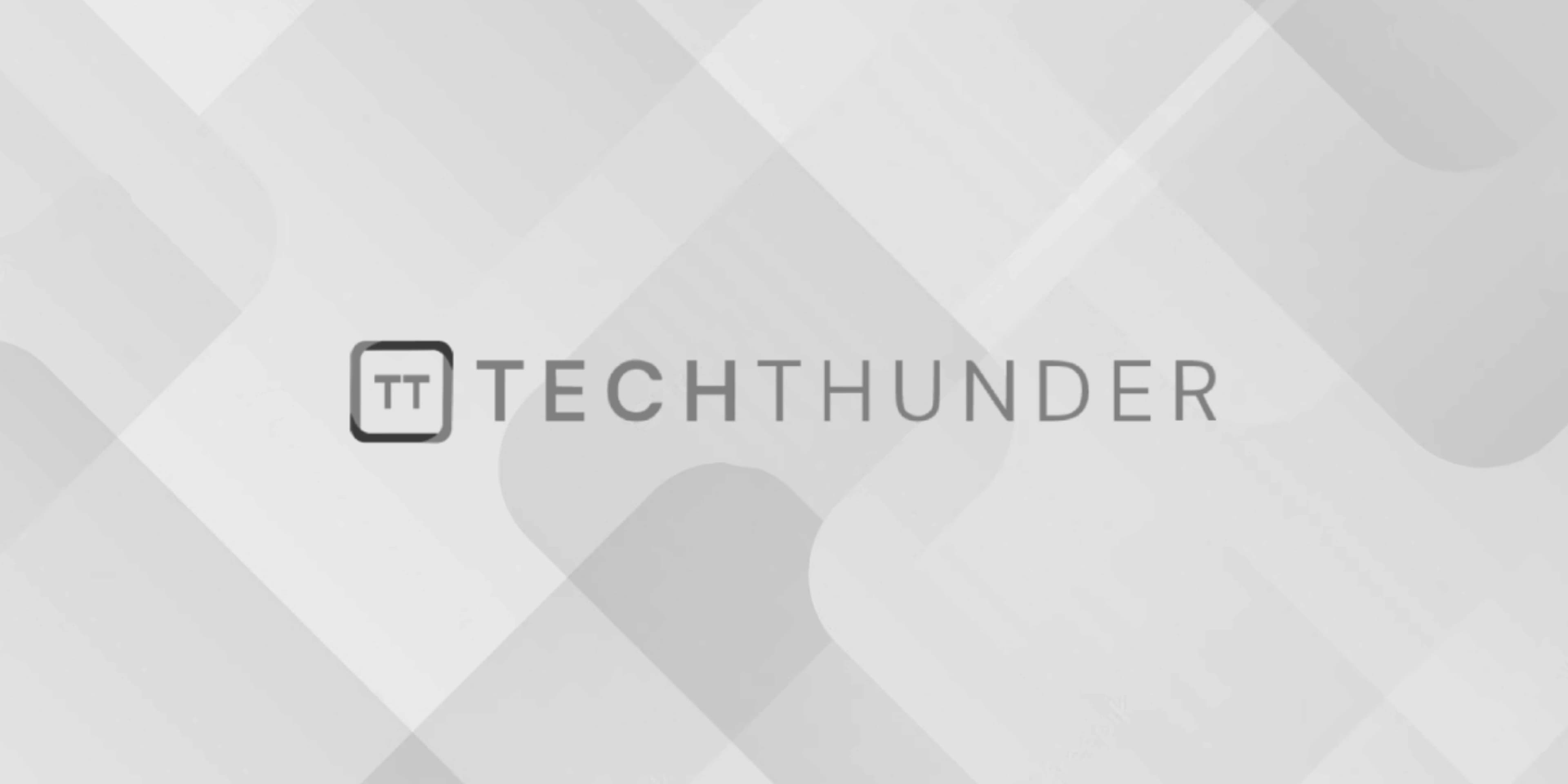
const Pointer in C
The const
pointer is a pointer that points to a constant value or a value that cannot be modified through that pointer. The const
qualifier can be used in different ways to specify what is constant: either the pointer itself or the value it points to. Here are some common uses of const
pointers in C:
- Constant Pointer to Non-Constant Data:
When you declare a constant pointer to non-constant data, it means that the pointer itself is constant, but you can modify the data it points to.
int x = 10;
int y = 20;
int *const ptr = &x; // Constant pointer to a non-constant integer
*ptr = 30; // Allowed, modifies the value pointed to by ptr
ptr = &y; // Not allowed, cannot change where ptr points
- Non-Constant Pointer to Constant Data:
When you declare a non-constant pointer to constant data, it means that the pointer can be modified to point elsewhere, but you cannot modify the value it points to.
const int x = 10;
const int y = 20;
const int *ptr1 = &x; // Non-constant pointer to a constant integer
int const *ptr2 = &y; // Equivalent declaration
*ptr1 = 30; // Not allowed, cannot modify the constant data
ptr1 = &y; // Allowed, can change where ptr1 points
- Constant Pointer to Constant Data:
When you declare a constant pointer to constant data, it means that both the pointer and the data it points to are constant, and neither can be modified.
const int x = 10;
const int y = 20;
const int *const ptr = &x; // Constant pointer to a constant integer
*ptr = 30; // Not allowed, cannot modify the constant data
ptr = &y; // Not allowed, cannot change where ptr points
The use of const
pointers helps in writing code that is more robust, self-documenting, and less error-prone. It provides a way to express your intent about whether you intend to modify the data, the pointer itself, or both. Additionally, it allows the compiler to catch certain types of errors at compile-time, preventing accidental modification of constant data or constant pointers.
When working with functions that accept pointers as arguments, you can also use const
to indicate whether the function will modify the data pointed to by the argument. For example:
void printValue(const int *ptr) {
printf("Value: %d\n", *ptr);
}
In this function, ptr
is declared as a pointer to a constant integer, indicating that the function will not modify the value pointed to by ptr
.