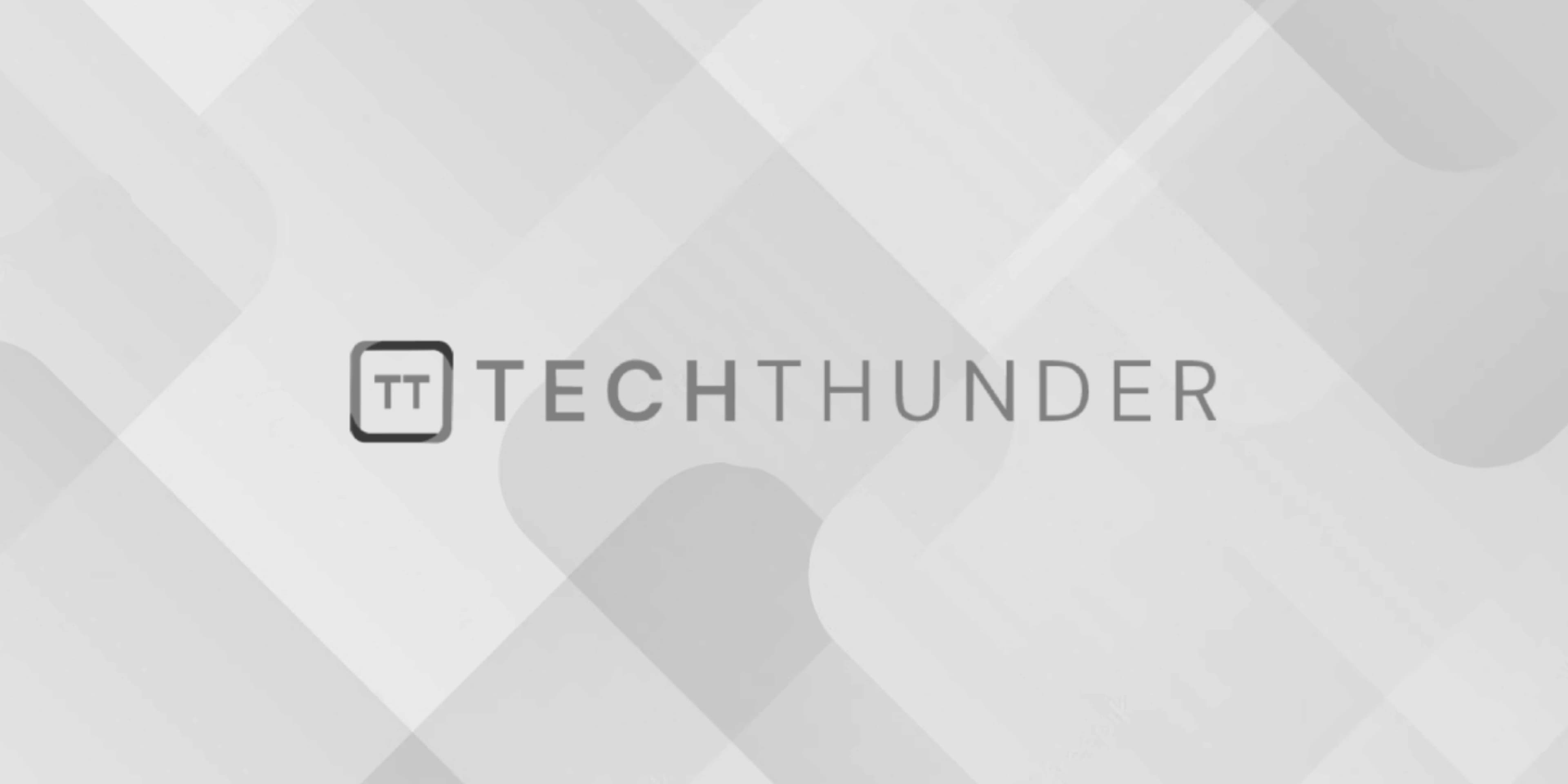
Structure Pointer in C
You can use structure pointers to work with structures (structs) more efficiently and flexibly. Structure pointers allow you to manipulate the members of a structure using pointers, which can be particularly useful when working with dynamic memory allocation and data structures.
Here’s how you can declare, create, and use structure pointers in C:
- Defining a Structure: First, you define a structure that represents the data you want to work with. For example:
struct Student {
char name[50];
int roll_number;
float GPA;
};
- Declaring a Structure Pointer: You can declare a pointer to a structure using the structure’s name followed by an asterisk (*). For example:
struct Student *studentPtr;
- Allocating Memory Dynamically: To allocate memory for a structure dynamically (usually on the heap), you can use functions like
malloc
orcalloc
. For example:
studentPtr = (struct Student *)malloc(sizeof(struct Student));
This allocates memory for a single struct Student
and assigns the pointer studentPtr
to the allocated memory.
- Accessing Structure Members using Pointers: You can access the members of the structure using the
->
operator with the structure pointer. For example:
strcpy(studentPtr->name, "John");
studentPtr->roll_number = 101;
studentPtr->GPA = 3.75;
This code sets the values of the structure members using the pointer studentPtr
.
- Deallocating Memory: Don’t forget to deallocate the memory when you’re done with it to prevent memory leaks. You can use the
free
function:
free(studentPtr);
Here’s a complete example that demonstrates the usage of structure pointers:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
char name[50];
int roll_number;
float GPA;
};
int main() {
struct Student *studentPtr;
// Allocate memory for a student
studentPtr = (struct Student *)malloc(sizeof(struct Student));
// Check if memory allocation was successful
if (studentPtr == NULL) {
printf("Memory allocation failed.\n");
return 1;
}
// Initialize the structure members
strcpy(studentPtr->name, "John");
studentPtr->roll_number = 101;
studentPtr->GPA = 3.75;
// Access and print the structure members
printf("Name: %s\n", studentPtr->name);
printf("Roll Number: %d\n", studentPtr->roll_number);
printf("GPA: %.2f\n", studentPtr->GPA);
// Deallocate the memory
free(studentPtr);
return 0;
}
This example demonstrates the use of a structure pointer to allocate memory dynamically for a struct Student
, initialize its members, access and print those members, and finally, deallocate the memory to avoid memory leaks.