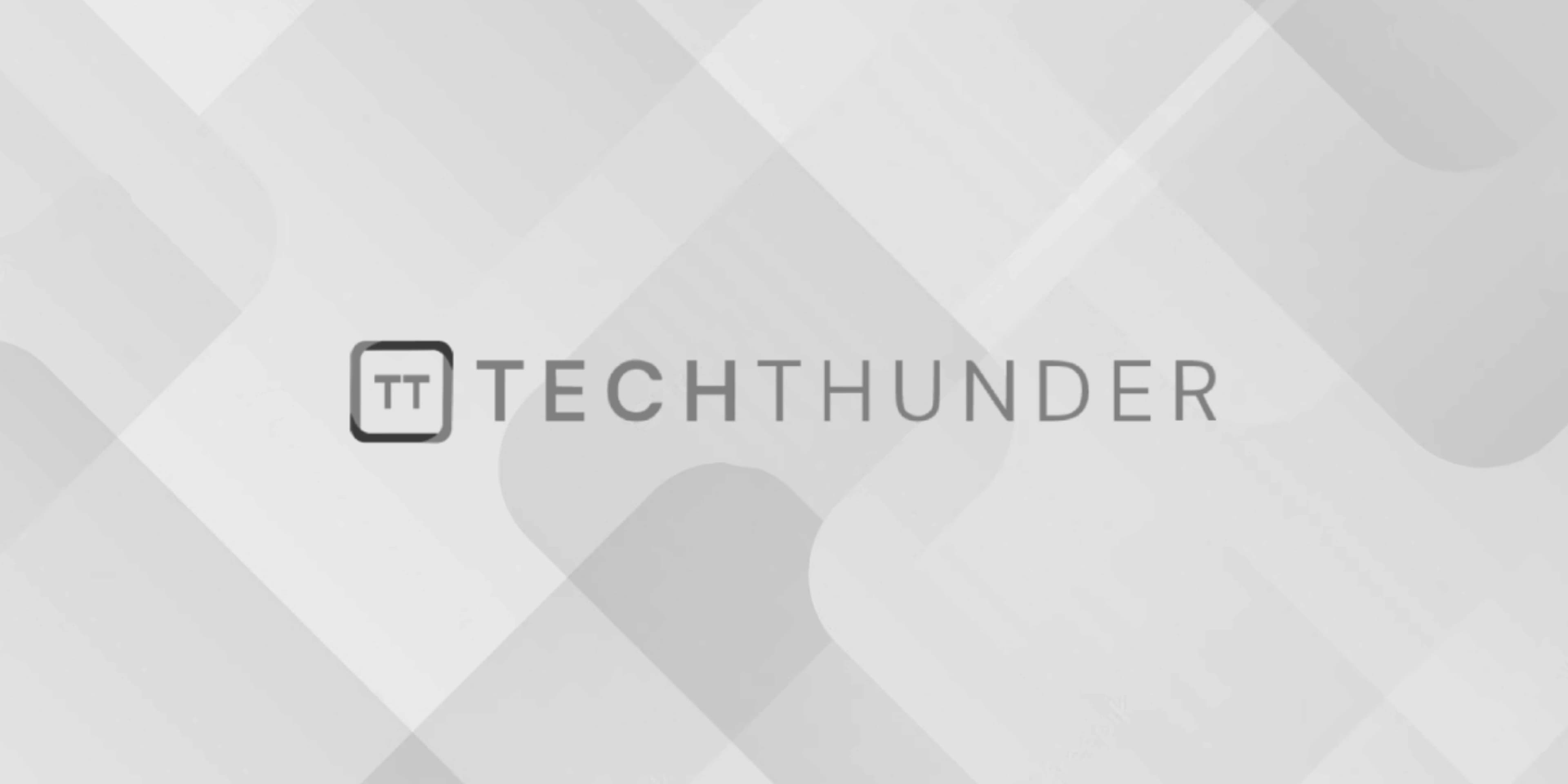
226 views
Remove Duplicate Elements from an Array in C
To remove duplicate elements from an array in C, you can follow these steps:
- Create a new array to store the unique elements.
- Iterate through the original array, and for each element, check if it has already been added to the new array.
- If the element is not in the new array, add it.
- After processing all elements, the new array will contain only unique elements.
Here’s an example C program to remove duplicates from an array:
C
#include <stdio.h>
int main() {
int originalArray[] = {1, 2, 2, 3, 4, 4, 5, 6, 6, 7};
int n = sizeof(originalArray) / sizeof(originalArray[0]);
int uniqueArray[n]; // Assuming the unique array won't have more elements than the original
int uniqueCount = 0; // Number of unique elements found so far
// Iterate through the original array
for (int i = 0; i < n; i++) {
int isDuplicate = 0;
// Check if the current element is already in the unique array
for (int j = 0; j < uniqueCount; j++) {
if (originalArray[i] == uniqueArray[j]) {
isDuplicate = 1;
break; // No need to check further
}
}
// If it's not a duplicate, add it to the unique array
if (!isDuplicate) {
uniqueArray[uniqueCount] = originalArray[i];
uniqueCount++;
}
}
// Print the unique elements
printf("Unique elements in the array: ");
for (int i = 0; i < uniqueCount; i++) {
printf("%d ", uniqueArray[i]);
}
printf("\n");
return 0;
}
In this program, we use two loops to process the original array. The outer loop iterates through all elements of the original array, while the inner loop checks whether the current element is already in the unique array. If it’s not in the unique array, we add it to the unique array and increment uniqueCount
. This way, we ensure that the unique array contains only unique elements.
After processing the array, we print the unique elements.