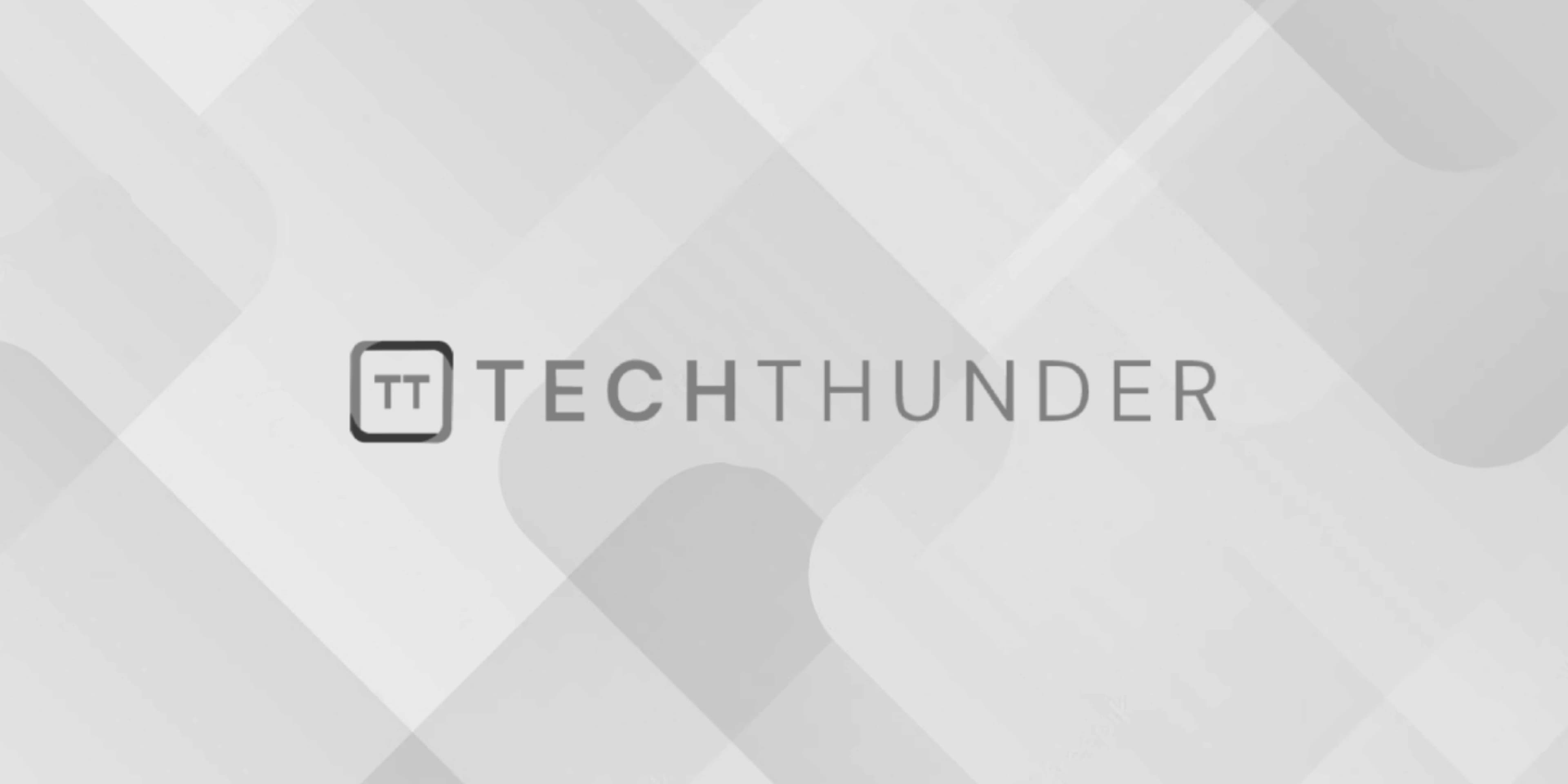
299 views
Structure of C program
A typical structure of a C program consists of various parts and elements. While C is a flexible language that doesn’t impose a strict structure, there are common components that most C programs include. Here is a basic structure of a C program:
C
// Preprocessor Directives (Headers)
#include <stdio.h>
// Additional header files, if needed
// Global Constants and Macros
#define MAX_VALUE 100
// Function Prototypes
void function1(int arg1);
int function2(int arg1, char arg2);
// Global Variables
int globalVar1;
float globalVar2;
// Main Function
int main() {
// Variable Declarations and Local Constants
int localVar1;
const double PI = 3.14159265359;
// Statements and Program Logic
printf("Hello, World!\n");
// Function Calls
function1(42);
int result = function2(10, 'A');
// Control Flow (if, switch, loops, etc.)
if (result > 0) {
printf("Result is positive.\n");
} else {
printf("Result is non-positive.\n");
}
// Return Statement (Optional)
return 0;
}
// Function Definitions
void function1(int arg1) {
// Function body
printf("Function 1 called with argument: %d\n", arg1);
}
int function2(int arg1, char arg2) {
// Function body
printf("Function 2 called with arguments: %d, %c\n", arg1, arg2);
return arg1 * 2;
}
Here’s a breakdown of the components of a typical C program:
- Preprocessor Directives (Headers): These are included at the beginning of the program to provide information to the compiler. Common headers like
<stdio.h>
are used to include standard libraries. - Global Constants and Macros: You can define global constants or macros using
#define
. Macros are typically used for conditional compilation. - Function Prototypes: These declare the functions that will be defined later in the program. It tells the compiler about the function’s name, return type, and parameter types.
- Global Variables: These are variables declared outside of any function and are accessible throughout the program.
- Main Function: The
main()
function is the entry point of a C program. It is where program execution begins. It contains declarations, statements, function calls, and control flow for your program. - Variable Declarations and Local Constants: These are declarations of local variables that are specific to the
main()
function or other functions. Constants declared usingconst
can also be defined here. - Statements and Program Logic: These are the actual program statements and logic that perform operations and calculations.
- Function Calls: Functions are called within the
main()
function or other functions to perform specific tasks or calculations. - Control Flow: Control structures like
if
,else
,switch
, and loops (e.g.,for
,while
) are used to control the flow of program execution. - Return Statement (Optional): The
main()
function typically ends with areturn
statement to indicate the program’s exit status. - Function Definitions: These are the implementations of functions declared earlier. Function definitions provide the actual code for the functions.
While this structure represents a basic C program, keep in mind that programs can vary widely in complexity and organization. Larger programs may use multiple source files, header files, and a more modular structure for better organization and maintainability.