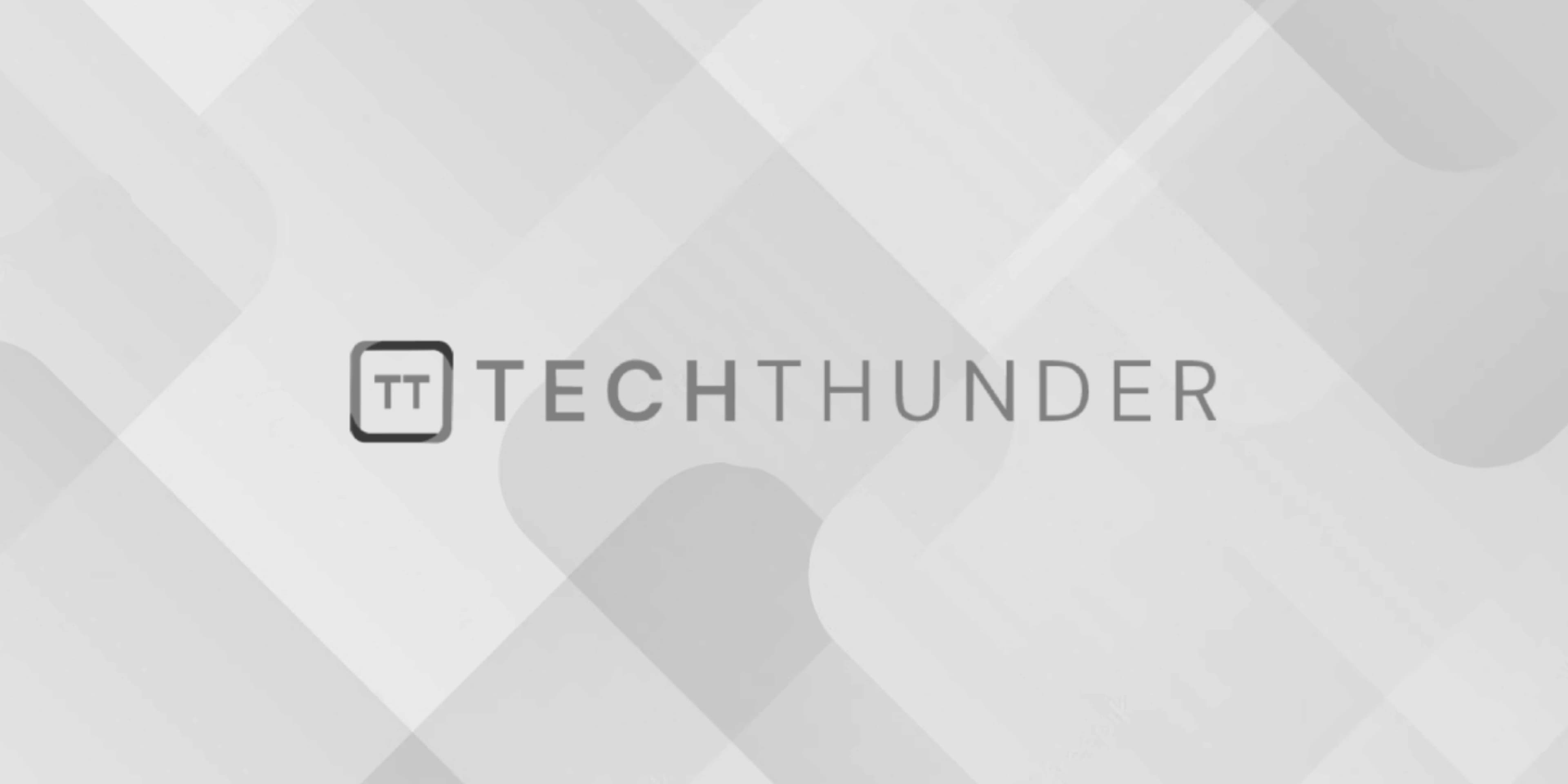
2s complement in C
The two’s complement representation is a common way to represent signed integers. It is used to represent both positive and negative integers using a fixed number of bits. Here’s how two’s complement works:
- Positive Numbers:
- Positive numbers in two’s complement representation are represented just like in regular binary notation.
- The most significant bit (the leftmost bit) is 0 to indicate a positive number. For example, the decimal number +5 can be represented in 8-bit two’s complement as
00000101
.
- Negative Numbers:
- Negative numbers in two’s complement representation are obtained by taking the two’s complement of the absolute value of the number.
- To calculate the two’s complement, invert (flip) all the bits and then add 1 to the result. For example, to represent the decimal number -5 in 8-bit two’s complement:
- Start with the binary representation of +5:
00000101
- Invert all the bits:
11111010
- Add 1 to the result:
11111011
So, -5 in 8-bit two’s complement is represented as11111011
.
- Range of Representable Numbers:
- In an N-bit two’s complement representation, you can represent numbers in the range from -2^(N-1) to 2^(N-1)-1.
- For example, in an 8-bit representation, you can represent numbers from -128 to 127.
Here’s a C program that demonstrates the conversion between decimal and two’s complement representations:
#include <stdio.h>
int main() {
int decimal_number = -5;
unsigned int twos_complement = (unsigned int)decimal_number; // Convert to unsigned for bitwise operations
printf("Decimal: %d\n", decimal_number);
printf("Binary (unsigned): %08x\n", twos_complement); // Print in hexadecimal format
// Calculate the two's complement representation
unsigned int two_comp = ~twos_complement + 1;
printf("Two's Complement: %08x\n", two_comp); // Print in hexadecimal format
return 0;
}
This program converts the decimal number -5 to its two’s complement representation. Note that the conversion involves bitwise operations and type casting to ensure that the bits are correctly interpreted. The result is printed both in binary (in hexadecimal format) and as a decimal number.
Understanding two’s complement is essential when working with signed integers in C, especially when performing bitwise operations or when dealing with low-level memory manipulation.