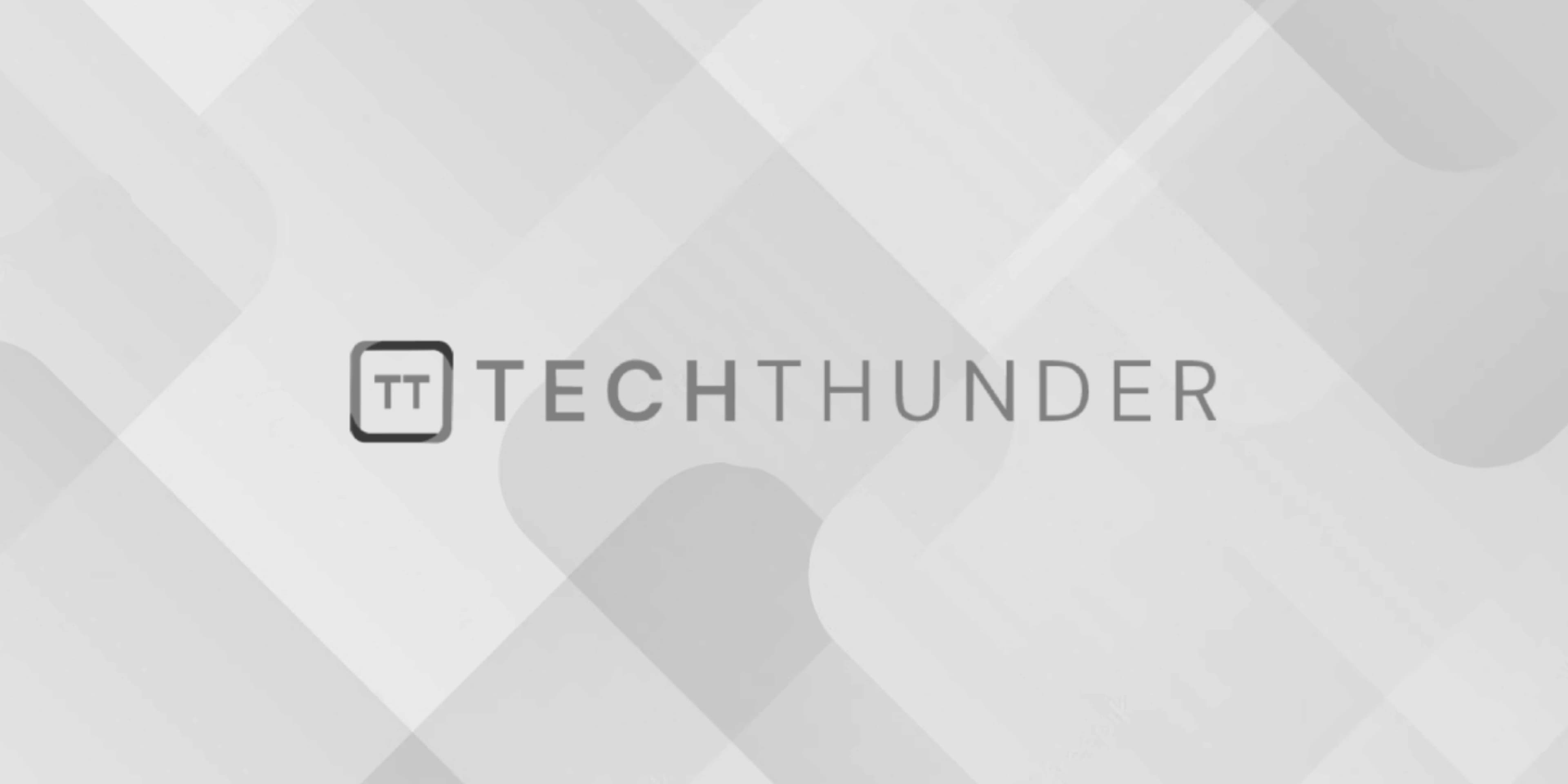
405 views
Square Numbers List in C
The simple C program that generates a list of square numbers within a specified range:
C
#include <stdio.h>
#include <math.h>
int main() {
int lower, upper;
printf("Enter the lower and upper bounds: ");
scanf("%d %d", &lower, &upper);
printf("Square numbers between %d and %d:\n", lower, upper);
for (int num = lower; num <= upper; ++num) {
int sqrtNum = (int)sqrt(num);
if (sqrtNum * sqrtNum == num) {
printf("%d ", num);
}
}
printf("\n");
return 0;
}
In this program, we calculate the square root of each number in the specified range and check if its square is equal to the original number. If it is, then the number is a perfect square. The sqrt
function from the math.h
library is used to calculate the square root.
This basic implementation should work for relatively small ranges of numbers. If you’re working with larger ranges, you might want to consider more efficient algorithms for identifying square numbers. As always, ensure that you validate user input to make sure it’s within an appropriate range.