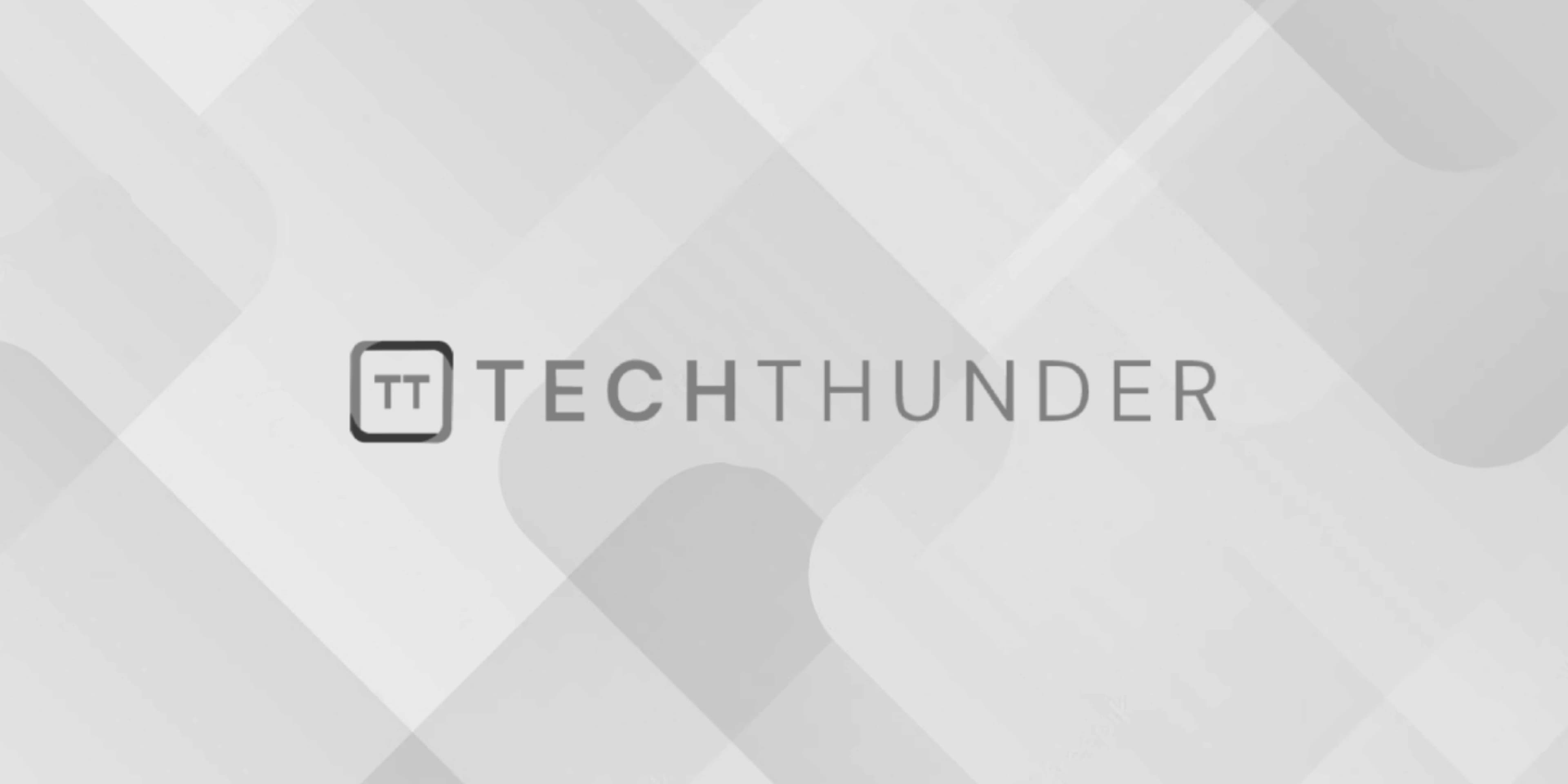
Cricket Score Sheet in C
Creating a cricket score sheet program in C can be a complex task due to the various aspects of cricket scoring, including runs, wickets, overs, and more. Below, I’ll provide a simplified example of how you can create a basic cricket score sheet in C to keep track of runs scored and wickets taken by a team. Please note that this is a minimalistic example and does not cover all aspects of cricket scoring.
#include <stdio.h>
int main() {
int runs = 0;
int wickets = 0;
int overs = 0;
int balls = 0;
printf("Cricket Score Sheet\n");
while (1) {
printf("Current Score: %d/%d\n", runs, wickets);
printf("Overs: %d.%d\n", overs, balls);
printf("Enter runs scored in this ball (0-6, -1 for wicket, -2 to exit): ");
int score;
scanf("%d", &score);
if (score == -1) {
wickets++;
} else if (score == -2) {
break;
} else if (score >= 0 && score <= 6) {
runs += score;
balls++;
if (balls == 6) {
overs++;
balls = 0;
}
} else {
printf("Invalid input. Please enter a valid score.\n");
}
}
printf("Final Score: %d/%d\n", runs, wickets);
printf("Overs: %d.%d\n", overs, balls);
return 0;
}
In this basic cricket score sheet program:
- The program initializes variables to keep track of runs, wickets, overs, and balls.
- It enters an infinite loop to accept input for each ball bowled.
- The user can input runs scored in each ball (0-6), mark a wicket (-1), or exit the program (-2).
- Runs are added to the total, and if a wicket is marked, the wickets count is incremented.
- Overs are incremented after every 6 balls.
- Invalid input is handled with a message.
- The loop exits when -2 is entered, and the final score and overs are displayed.
Please note that this is a basic example for educational purposes and does not cover all the complexities of a real cricket score sheet, such as recording individual batsman statistics, bowler details, fielding positions, and more. Real cricket score sheets are typically more comprehensive and structured.