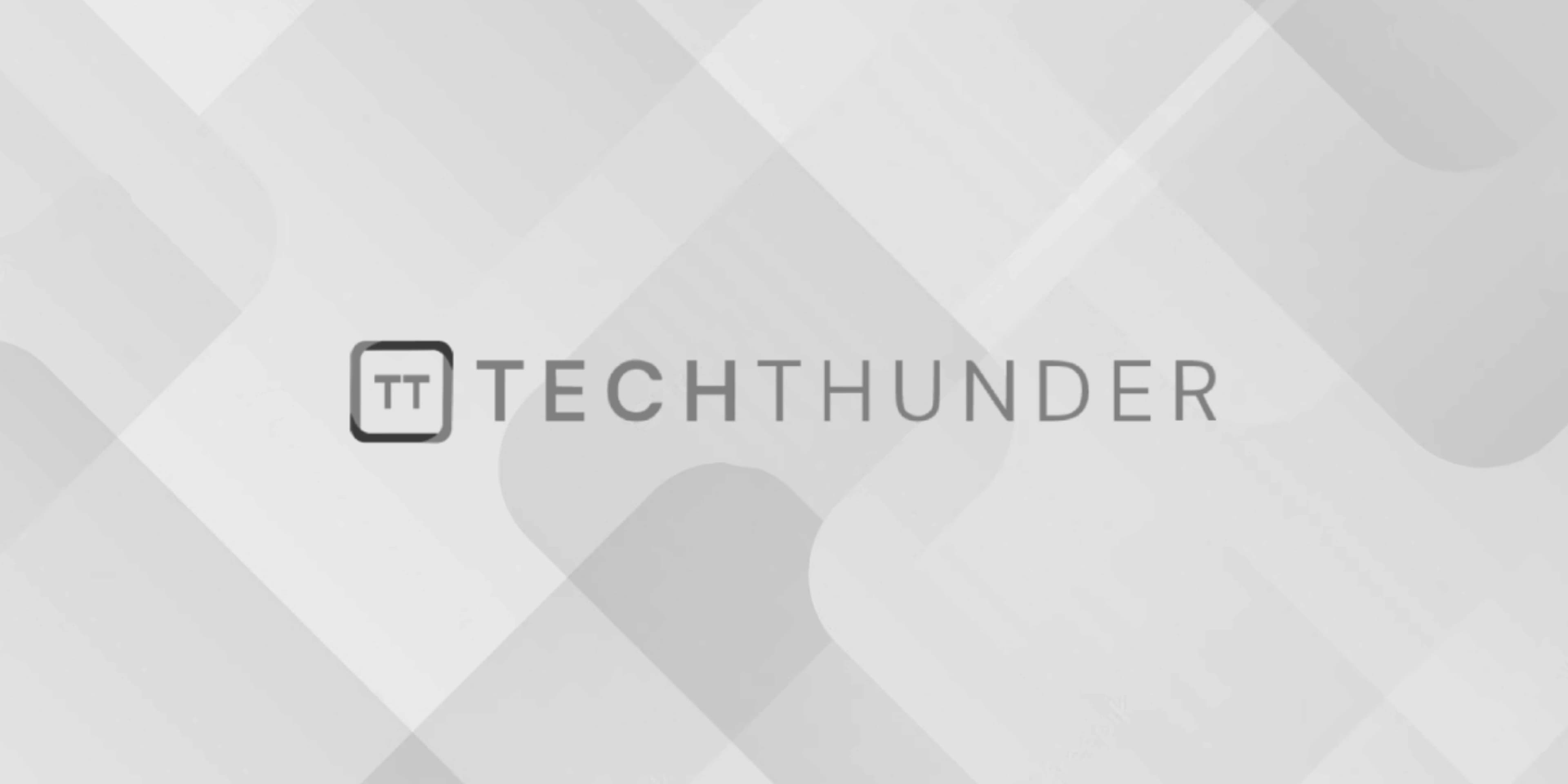
195 views
C Functions Test
The C function is a reusable block of code that performs a specific task. Functions are used to break down a program into smaller, manageable parts, making the code more organized, modular, and easier to maintain. Functions allow you to structure your program logically and promote code reuse. Here are some questions of varying difficulty:
- Function Basics:
- What is a function in C?
- Explain the difference between a function declaration and a function definition.
- What is the purpose of the
return
statement in a function? - How is a function called in C?
- Function Parameters:
- What are function parameters, and why are they used?
- Explain the difference between call by value and call by reference in C.
- Write a C function that swaps the values of two integer variables using call by reference.
- Function Prototypes:
- What is a function prototype, and why is it used?
- How can you declare a function prototype for a function defined in a different source file?
- Explain the role of function prototypes in preventing errors in your C program.
- Recursion:
- What is recursion in the context of functions?
- Write a recursive function to calculate the factorial of a non-negative integer.
- What are the base cases in a recursive function, and why are they important?
- Function Pointers:
- What is a function pointer in C?
- Write a C program that uses a function pointer to perform arithmetic operations (addition, subtraction, multiplication, division) on two integers.
- How can function pointers be useful in creating flexible and extensible code?
- Standard Library Functions:
- Provide examples of standard library functions in C for:
- Input/output (e.g.,
printf
,scanf
) - String manipulation (e.g.,
strlen
,strcpy
) - Mathematical operations (e.g.,
sqrt
,rand
)
- Input/output (e.g.,
- Variable Scope:
- Explain the concept of variable scope in C.
- What are local variables, and where are they accessible?
- What are global variables, and how do they differ from local variables?
- Static Variables:
- Describe the characteristics of static variables in C.
- How does the lifetime of a static variable differ from that of an automatic (local) variable?
- Provide an example of when you would use a static variable.
- Function Overloading (Not Supported in C):
- Explain the concept of function overloading.
- Why is function overloading not supported in C, and how is it different from languages like C++?
- Error Handling:
- How can you handle errors or exceptional cases in a C program using functions?
- Describe the use of error codes or return values to indicate success or failure in functions.
Feel free to use these questions for your test or quiz on C functions. You can also adapt or modify them as needed to suit your specific requirements and the level of your audience.