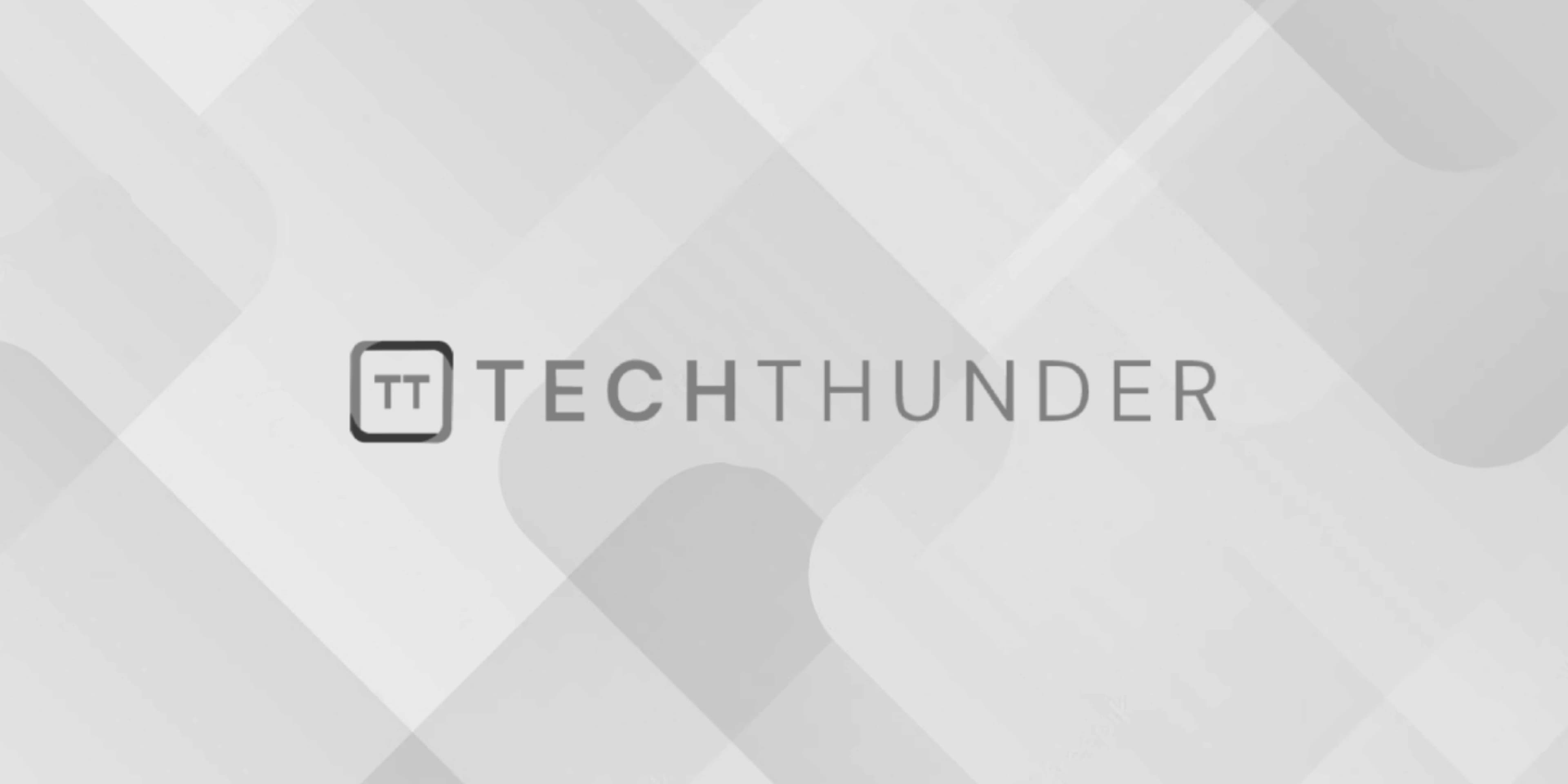
186 views
Add Two Matrices in C
To add two matrices in C, both matrices should have the same number of rows and columns. You can perform matrix addition element-wise by iterating through both matrices and adding corresponding elements together. Here’s a C program to add two matrices:
C
#include <stdio.h>
int main() {
int rows, cols;
// Input the dimensions of the matrices
printf("Enter the number of rows: ");
scanf("%d", &rows);
printf("Enter the number of columns: ");
scanf("%d", &cols);
// Initialize the matrices
int matrix1[rows][cols];
int matrix2[rows][cols];
int result[rows][cols];
// Input elements of the first matrix
printf("Enter elements of the first matrix:\n");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
scanf("%d", &matrix1[i][j]);
}
}
// Input elements of the second matrix
printf("Enter elements of the second matrix:\n");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
scanf("%d", &matrix2[i][j]);
}
}
// Perform matrix addition
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result[i][j] = matrix1[i][j] + matrix2[i][j];
}
}
// Display the result matrix
printf("Result of matrix addition:\n");
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%d\t", result[i][j]);
}
printf("\n");
}
return 0;
}
In this program, you first input the dimensions (number of rows and columns) of the matrices. Then, you initialize the matrices and read the elements for both matrices. After that, you perform matrix addition element-wise and store the result in the result
matrix. Finally, you display the result matrix.
Make sure to enter the elements of both matrices carefully, ensuring that they have the same dimensions for addition to be valid.