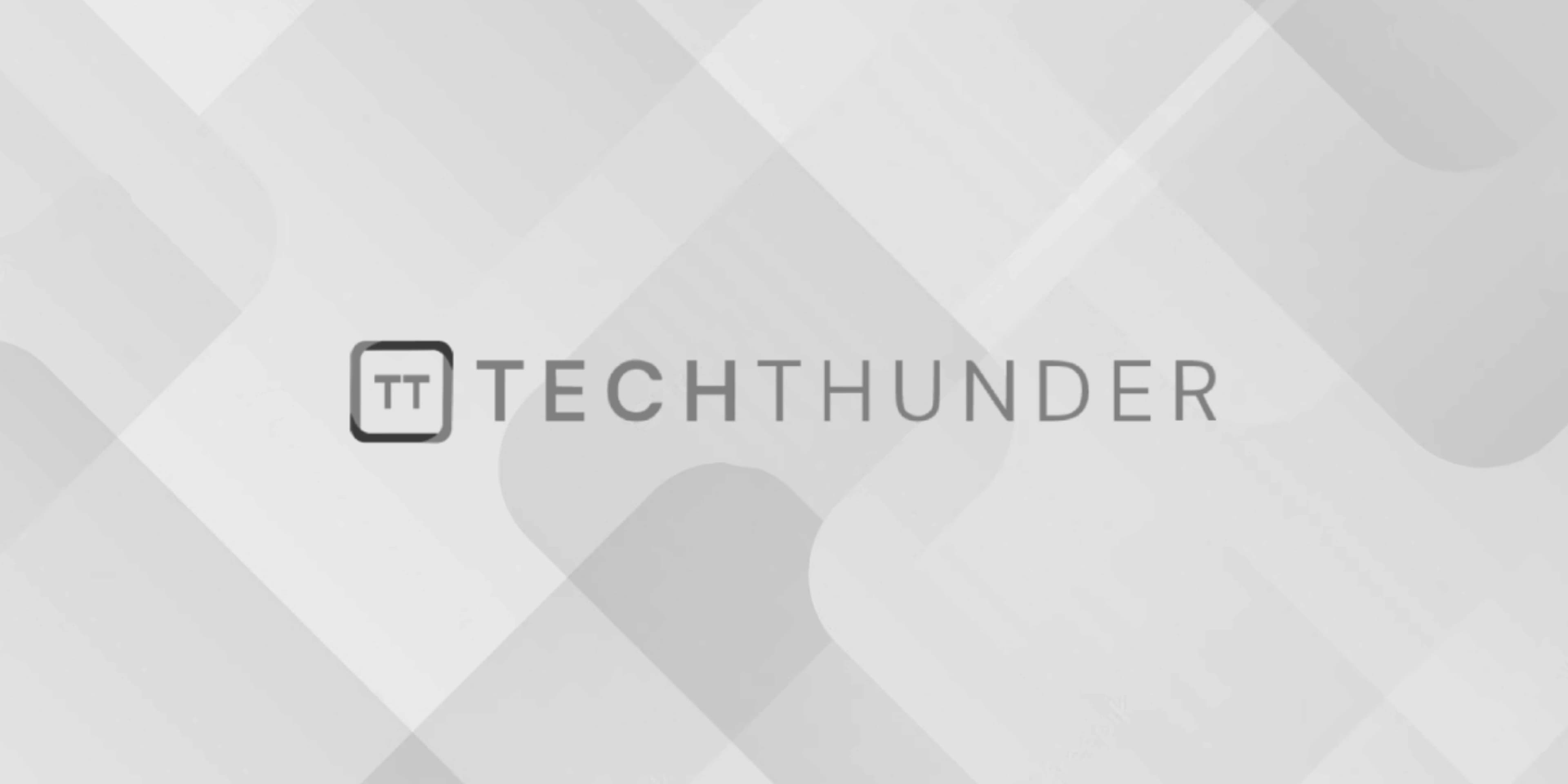
Infinite Loop in C
The infinite loop in C is a loop that continues to execute indefinitely, without ever terminating on its own. Infinite loops can be intentional or unintentional and are typically used in specific situations where continuous execution is required. Here are some common ways to create infinite loops in C:
- Using
while
Loop:
You can create an infinite loop using awhile
loop with a condition that always evaluates to true.
while (1) {
// Loop body
}
In this case, the condition 1
is always true, so the loop continues indefinitely.
- Using
for
Loop:
Similarly, you can create an infinite loop using afor
loop with a condition that is always true.
for (;;) {
// Loop body
}
The absence of a condition in the for
loop header makes it an infinite loop.
- Using
do-while
Loop:
You can create an infinite loop using ado-while
loop by placing the loop condition at the end, ensuring that the loop body always executes at least once.
do {
// Loop body
} while (1);
The condition 1
ensures that the loop continues to execute after the first iteration.
- Using a Conditional Statement:
You can also create an infinite loop by placing a conditional statement that always evaluates to true.
if (1) {
// Loop body
}
In this case, the if
statement’s condition is always true, causing the loop body to execute.
- Using
goto
Statement:
While it’s generally discouraged to use thegoto
statement, you can create an infinite loop using it.
start:
// Loop body
goto start;
The goto
statement jumps back to the start
label, creating an infinite loop.
It’s important to note that infinite loops can be useful in certain situations, such as when creating server programs that continually listen for incoming connections, implementing real-time systems, or running background tasks. However, they should be used judiciously and with caution, as they can lead to programs that are difficult to control and may consume excessive CPU resources.
In most cases, it’s a good practice to include a way to exit or break out of an infinite loop when a specific condition is met to avoid unintended behavior or program lockup.