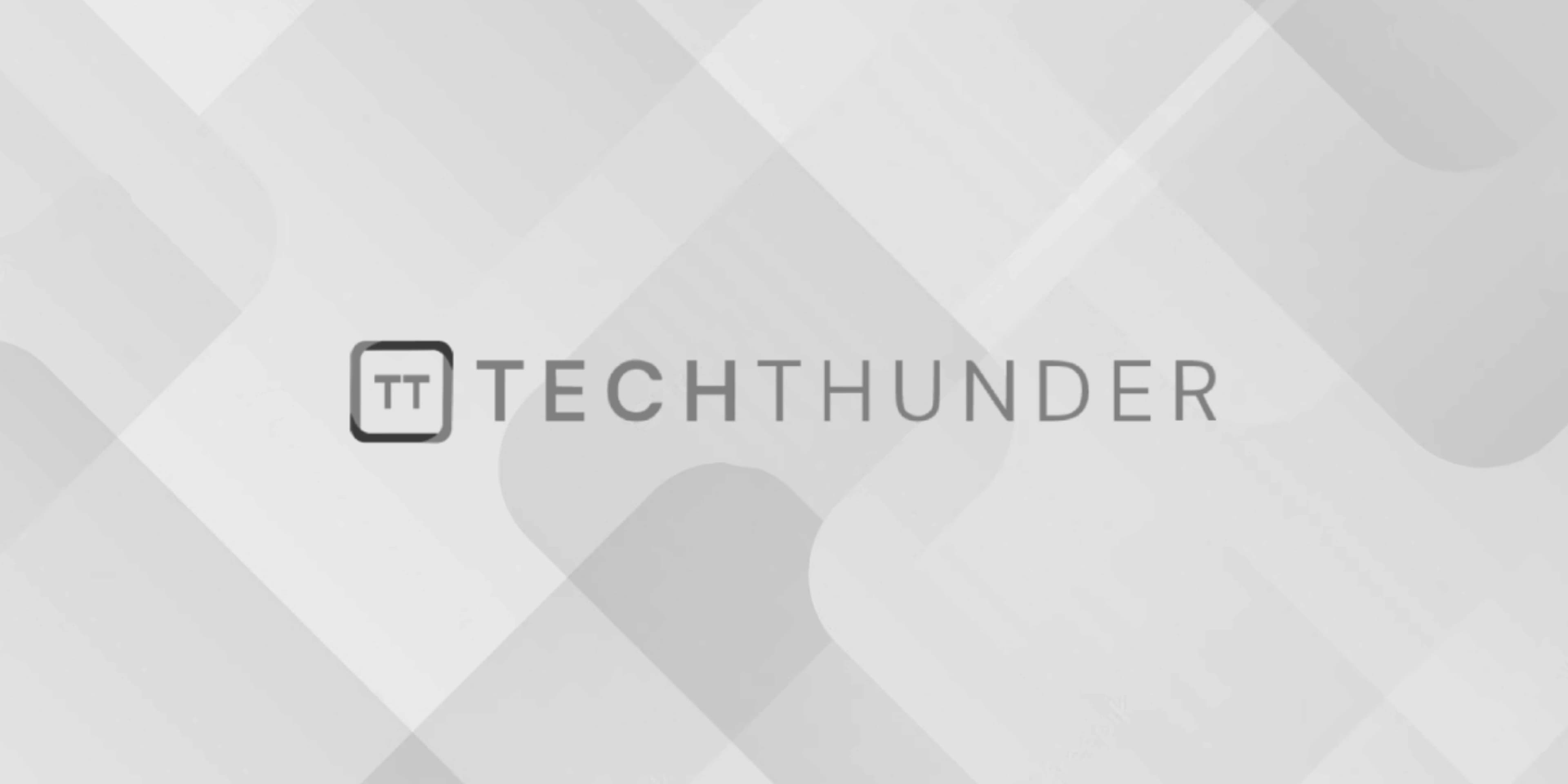
175 views
islower() in C
The islower()
function in C is part of the C Standard Library, and it is used to check if a given character is a lowercase letter in the ASCII character set. It takes an integer argument (typically representing a character) and returns an integer value, typically 1 (true) if the character is a lowercase letter and 0 (false) otherwise.
Here is the prototype of the islower()
function:
C
int islower(int c);
c
: An integer representing a character.
Here’s an example of how to use islower()
:
C
#include <stdio.h>
#include <ctype.h>
int main() {
char character = 'a';
if (islower(character)) {
printf("%c is a lowercase letter.\n", character);
} else {
printf("%c is not a lowercase letter.\n", character);
}
return 0;
}
In this example:
- We include the
<ctype.h>
header, which contains theislower()
function. - We check if the character
'a'
is a lowercase letter usingislower()
. Since'a'
is indeed a lowercase letter, the program prints that it is.
You can use the islower()
function to perform character-level checks in your C programs, especially when dealing with input validation or character classification.