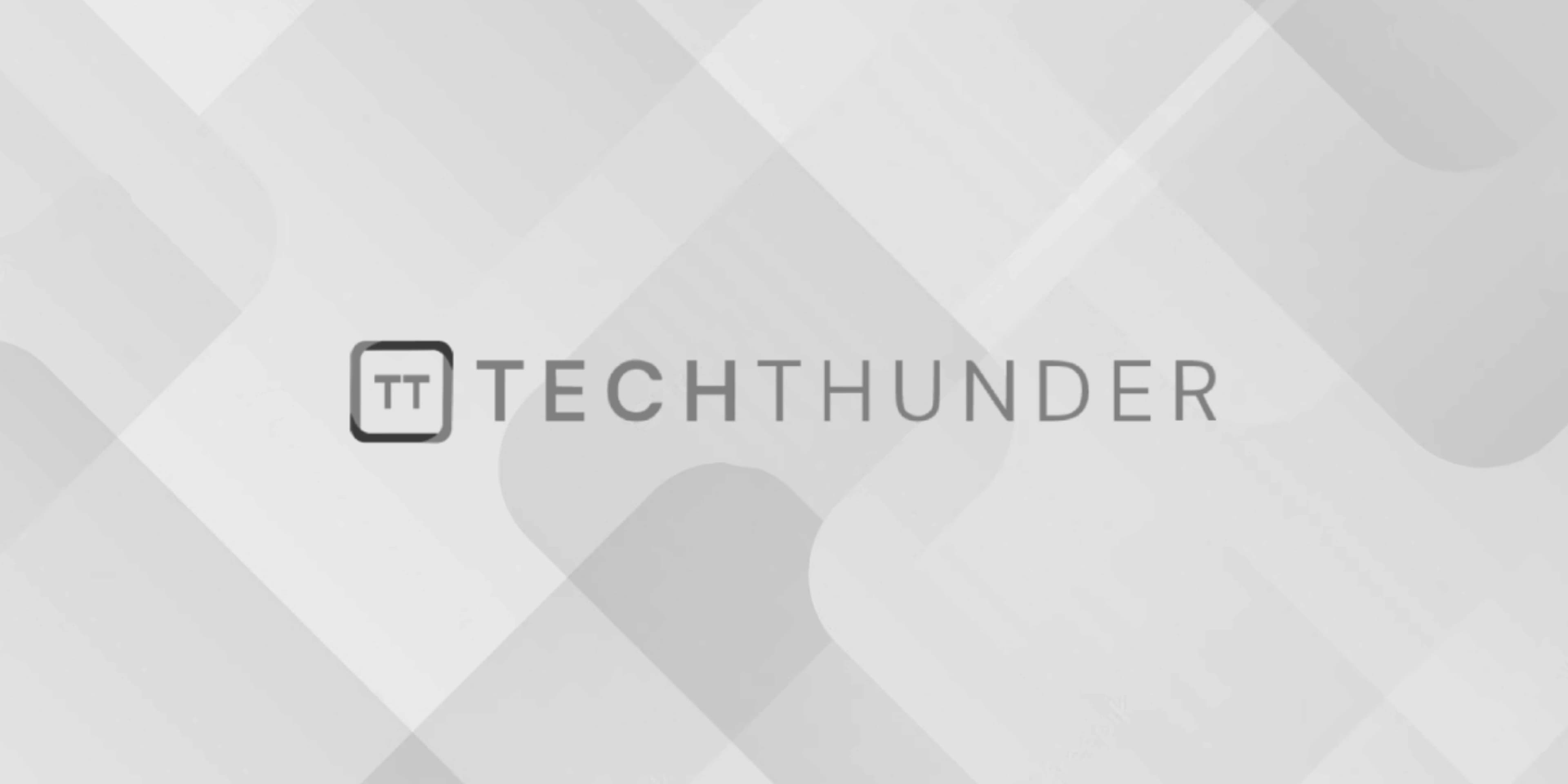
177 views
Sum of first N natural numbers in C
You can calculate the sum of the first N natural numbers (positive integers) in C using a simple loop. The sum of the first N natural numbers is given by the formula:
C
Sum = 1 + 2 + 3 + ... + N = N * (N + 1) / 2
Here’s a C program to calculate the sum of the first N natural numbers:
C
#include <stdio.h>
int main() {
int N;
long long sum = 0; // Use a long long to handle larger values
printf("Enter a positive integer N: ");
scanf("%d", &N);
if (N < 1) {
printf("N must be a positive integer.\n");
} else {
// Calculate the sum using the formula
sum = (long long)N * (N + 1) / 2;
printf("Sum of the first %d natural numbers is %lld\n", N, sum);
}
return 0;
}
In this program:
- We prompt the user to enter a positive integer N.
- We check if N is less than 1 (i.e., not a positive integer) and provide an error message if it’s not.
- If N is a positive integer, we calculate the sum using the formula
sum = (long long)N * (N + 1) / 2
and then display the result.
Note that we use a long long
data type for the sum
variable to handle larger values of N without overflowing.
Compile and run the program, and it will calculate and display the sum of the first N natural numbers based on the user’s input.