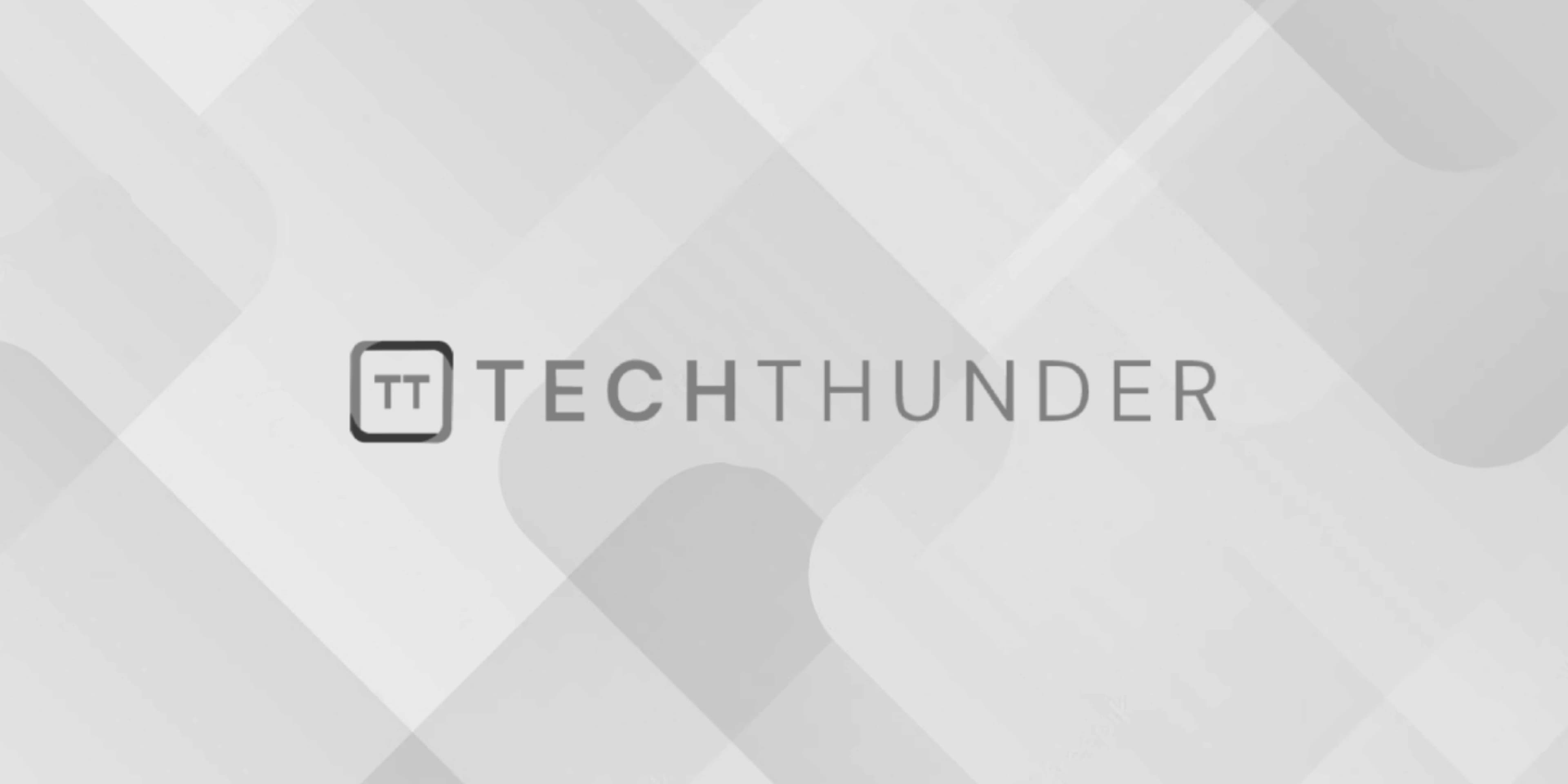
Call Value & Reference in C
The function arguments can be passed by value or by reference, depending on how you declare and use the function parameters. Understanding the difference between these two methods is crucial for proper variable manipulation in C.
- Call by Value:
- When you pass an argument to a function by value, a copy of the argument’s value is created and used within the function. Any changes made to the parameter inside the function do not affect the original variable outside the function.
- This method is commonly used for simple data types like integers, characters, and floating-point numbers. Example of call by value:
#include <stdio.h>
// Function to increment an integer by value
void incrementByValue(int num) {
num += 1;
}
int main() {
int x = 5;
incrementByValue(x); // Call by value
printf("Value of x: %d\n", x); // Output: Value of x: 5
return 0;
}
In this example, x
remains unchanged after the function call because num
inside the incrementByValue
function is a copy of x
.
- Call by Reference (Using Pointers):
- When you pass an argument to a function by reference, you pass the memory address (pointer) of the variable. Inside the function, you can manipulate the original variable using this pointer. Changes made to the parameter inside the function affect the original variable.
- This method is commonly used for complex data types like arrays and structs. Example of call by reference using pointers:
#include <stdio.h>
// Function to increment an integer by reference using a pointer
void incrementByReference(int *num) {
(*num) += 1; // Increment the value pointed to by 'num'
}
int main() {
int x = 5;
incrementByReference(&x); // Call by reference using a pointer
printf("Value of x: %d\n", x); // Output: Value of x: 6
return 0;
}
In this example, x
is incremented inside the incrementByReference
function because it receives a pointer to x
.
- Call by Reference (Using Reference Variables):
- C does not have native support for call by reference using reference variables like some other programming languages (e.g., C++). However, you can achieve a similar effect by using pointers, as shown in the previous example.
- Returning Values by Reference (Using Pointers):
- While C does not support direct call by reference for variables, you can return values by reference using pointers. A function can return a pointer to a variable or data structure, allowing you to modify the original variable outside the function. Example of returning a value by reference using a pointer:
#include <stdio.h>
// Function to create and return an integer by reference using a pointer
int *createAndReturnReference() {
int *num = (int *)malloc(sizeof(int)); // Allocate memory for an integer
*num = 42; // Set the value
return num; // Return a pointer to the integer
}
int main() {
int *xPtr = createAndReturnReference();
printf("Value of x: %d\n", *xPtr); // Output: Value of x: 42
free(xPtr); // Free the dynamically allocated memory
return 0;
}
In this example, createAndReturnReference
dynamically allocates an integer, sets its value, and returns a pointer to it.
While C does not have native call by reference like some other languages, you can use pointers to achieve similar functionality when you need to modify variables within functions. However, you should be cautious when using pointers to ensure proper memory management and avoid potential issues like memory leaks or undefined behavior.