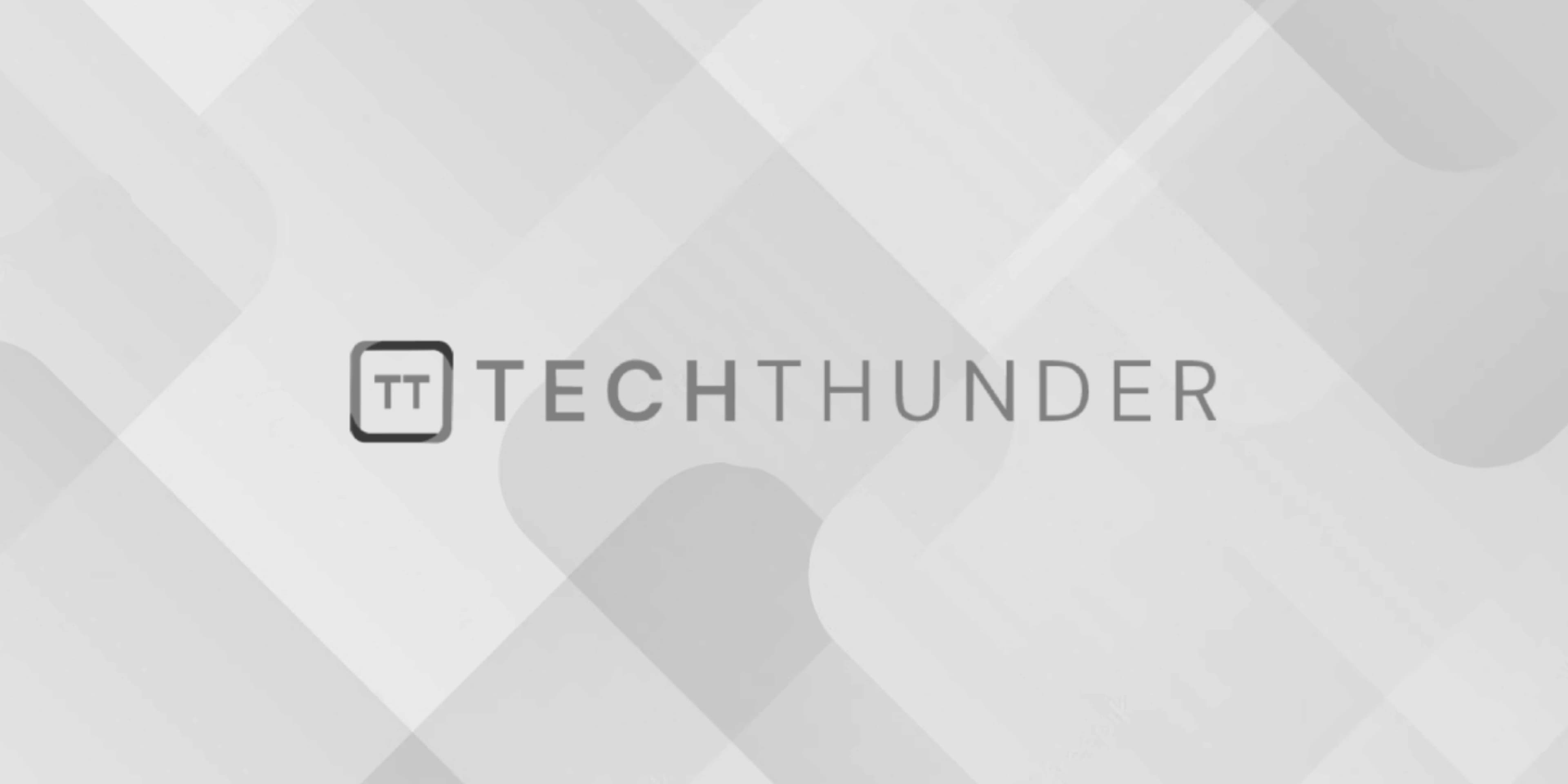
132 views
Function pointer as argument in C
You can pass function pointers as arguments to functions, allowing you to create more flexible and extensible code. This technique is often used to implement callbacks or to allow functions to operate on various types of data and behaviors. Here’s how you can pass function pointers as arguments in C:
C
#include <stdio.h>
// Define a function pointer type for a binary operation
typedef int (*BinaryOperation)(int, int);
// Function that takes a binary operation function pointer as an argument
int applyOperation(int a, int b, BinaryOperation operation) {
return operation(a, b);
}
// Example binary operations
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
int multiply(int a, int b) {
return a * b;
}
int main() {
int result;
// Pass different operation functions to applyOperation
result = applyOperation(5, 3, add);
printf("5 + 3 = %d\n", result);
result = applyOperation(5, 3, subtract);
printf("5 - 3 = %d\n", result);
result = applyOperation(5, 3, multiply);
printf("5 * 3 = %d\n", result);
return 0;
}
In this example:
- We define a function pointer type
BinaryOperation
that represents functions taking two integers and returning an integer. - The
applyOperation
function takes two integers (a
andb
) and a function pointer (operation
) as arguments. It applies the specified binary operation to the two integers and returns the result. - We define three binary operation functions (
add
,subtract
, andmultiply
) that match theBinaryOperation
function pointer type. - In the
main()
function, we pass different operation functions toapplyOperation
to demonstrate how it can be used to apply various operations dynamically.
Function pointers as arguments are a powerful way to make your code more flexible and modular. They allow you to change the behavior of a function at runtime, which can be useful in situations like callbacks, sorting functions, and other scenarios where the behavior of a function needs to be determined dynamically.