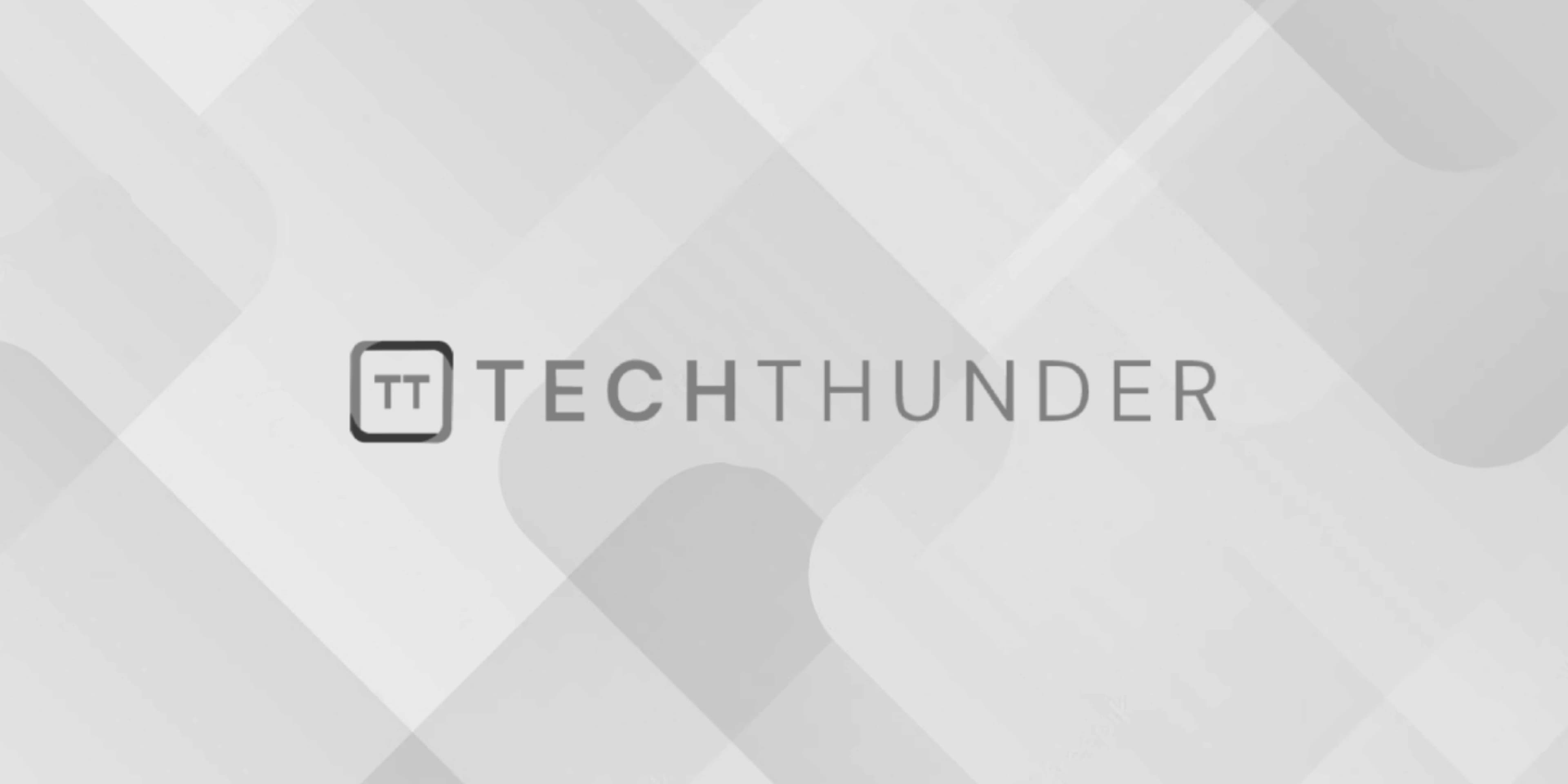
194 views
Add 2 Strings in C
You can concatenate (combine) two strings by using the standard library function strcat()
from the <string.h>
header. Here’s how you can add two strings together:
#include <stdio.h>
#include <string.h>
int main() {
char str1[50] = "Hello, ";
char str2[] = "world!";
strcat(str1, str2); // Concatenate str2 to str1
printf("Concatenated String: %s\n", str1);
return 0;
}
In this example:
- We include the
<stdio.h>
and<string.h>
headers for input/output and string manipulation functions, respectively. - We declare two character arrays (
str1
andstr2
) to hold the two strings you want to concatenate.str1
is initialized with the string “Hello, “. - We use the
strcat()
function to concatenatestr2
to the end ofstr1
. Thestrcat()
function takes the destination string (where the result will be stored) as its first argument and the source string (the string to be added) as its second argument. - Finally, we print the concatenated string, which will be “Hello, world!” in this case.
Keep in mind that when using strcat()
, it assumes that there is enough space in the destination string to hold the concatenated result. If there isn’t enough space, it can lead to buffer overflow and undefined behavior. Always ensure that the destination string has enough capacity to hold the combined result.