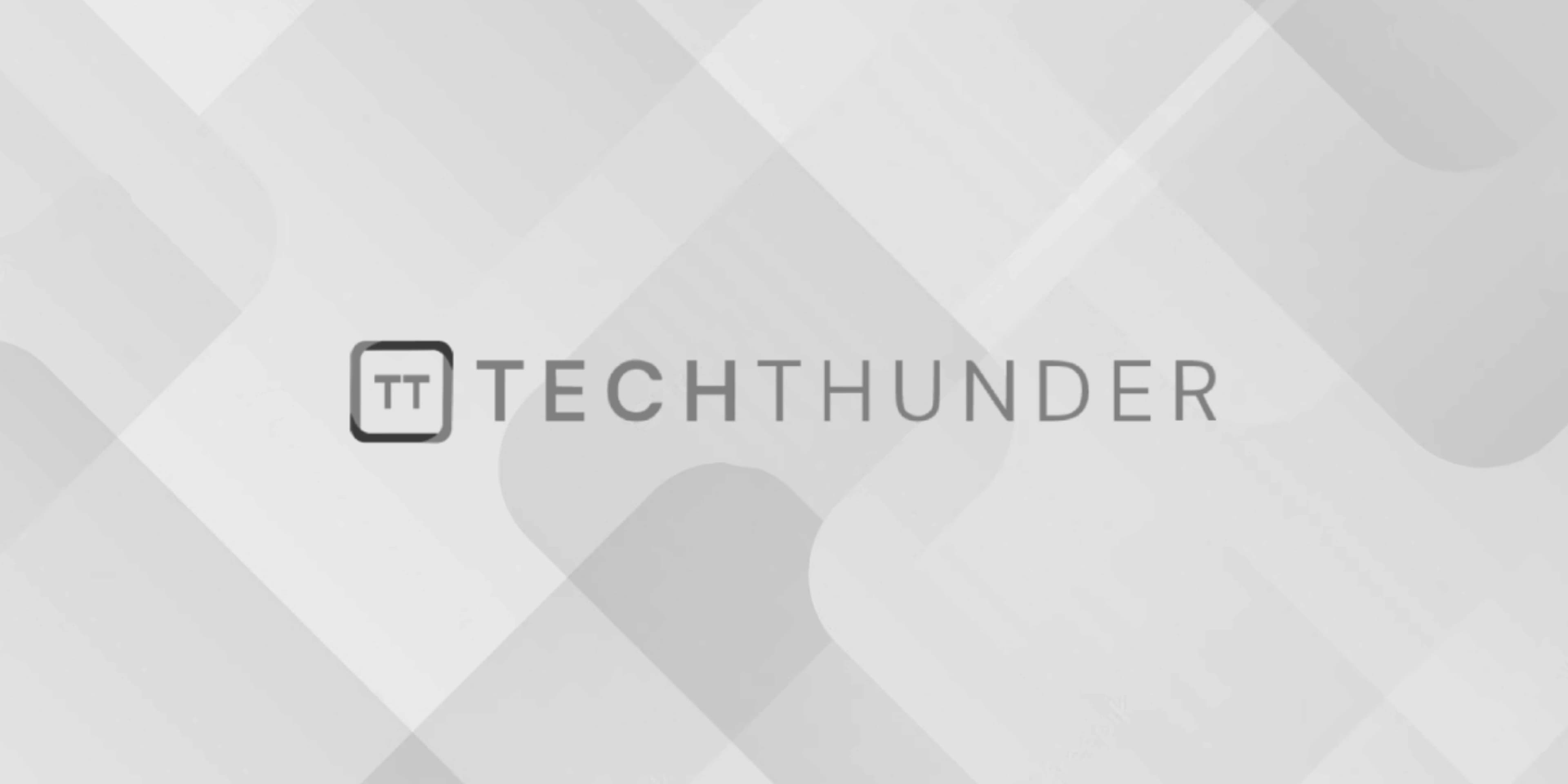
C Type Casting
Type casting in C is the process of converting a value from one data type to another. It allows you to change the data type of a value temporarily or permanently, depending on your program’s needs. Type casting is useful in situations where you want to perform operations or assignments involving variables of different data types.
There are two types of type casting in C:
- Implicit Type Casting (Type Coercion):
- Implicit type casting, also known as type coercion, occurs automatically when the compiler converts one data type to another without requiring explicit instructions from the programmer.
- It typically occurs in situations where a “smaller” data type is promoted to a “larger” data type to avoid data loss or to facilitate arithmetic operations.
- For example, when adding an
int
and adouble
, theint
is implicitly converted to adouble
before the addition.
int integerNumber = 5;
double doubleNumber = 3.14;
double result = integerNumber + doubleNumber; // Implicit type casting
- Explicit Type Casting (Type Conversion):
- Explicit type casting, also known as type conversion, requires the programmer to explicitly specify the desired type conversion using casting operators.
- It allows you to change the data type of a value intentionally.
- The casting operator
(type)
is used to specify the target data type.
double pi = 3.14159265359;
int truncatedPi = (int)pi; // Explicit type casting to int
In this example, the explicit type casting operation converts the double
value pi
to an int
, resulting in the truncated value.
Common casting operators used in explicit type casting include:
(int)
for integer casting(double)
for double casting(float)
for float casting(char)
for character casting
It’s important to note that explicit type casting may result in data loss or unexpected behavior if the new data type cannot fully represent the original value. Care should be taken when using explicit type casting to ensure that the conversion is valid and appropriate for the given situation.
Here’s an example of explicit type casting to convert a double
to an int
:
double pi = 3.14159265359;
int truncatedPi = (int)pi; // Explicit type casting to int
In this case, the double
value pi
is explicitly cast to an int
, resulting in truncatedPi
having the value 3
.