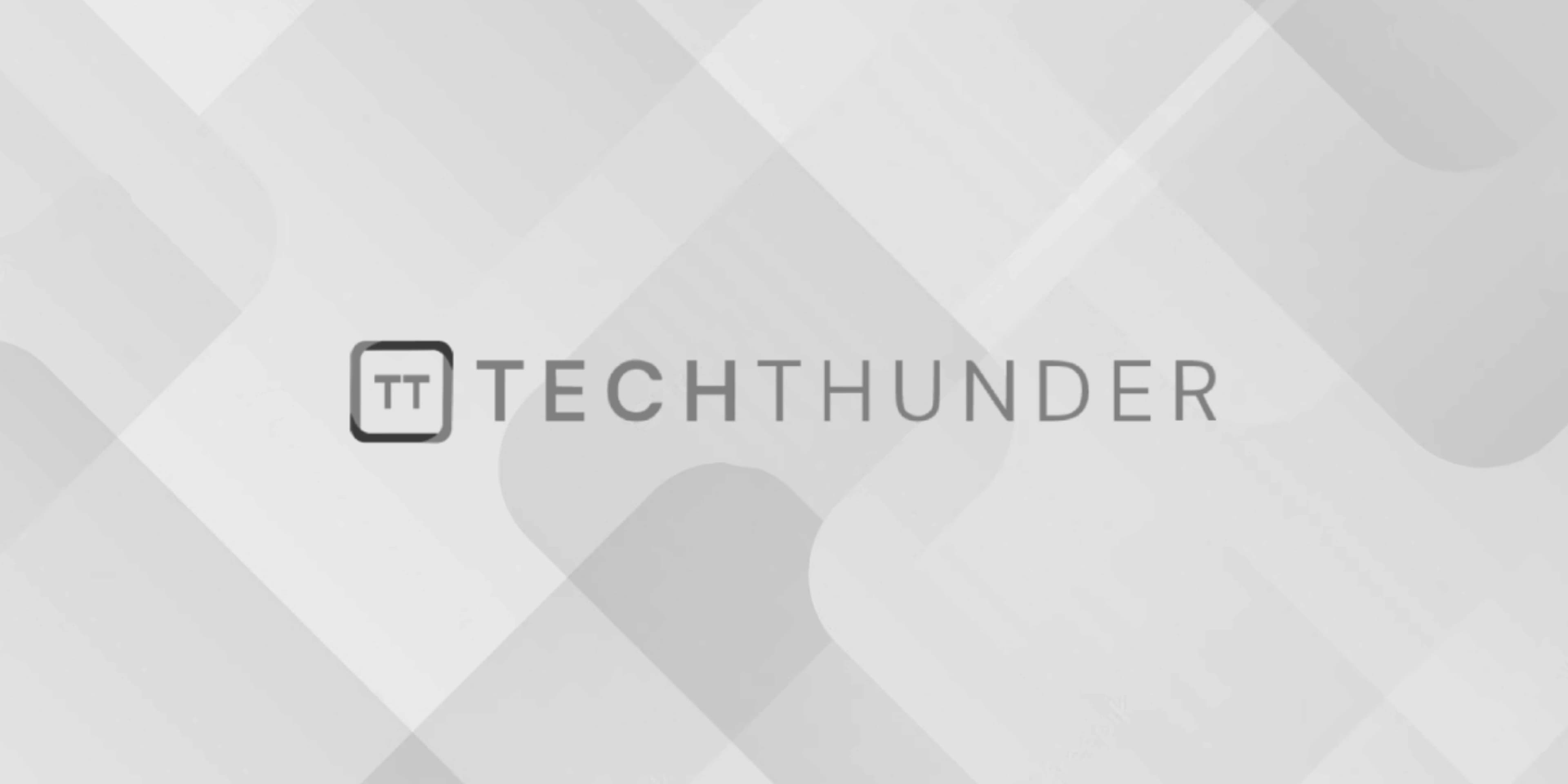
Customer Billing System in C
Creating a complete customer billing system in C is a complex task that involves multiple components and features. To give you a starting point, I’ll provide a simplified example of a console-based customer billing system in C. This example will cover basic functionalities like adding customers, calculating bills, and displaying customer information.
Please note that this is a minimalistic example, and a real-world billing system would require more extensive features, error handling, and potentially a database for data storage.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_CUSTOMERS 100
// Customer structure
typedef struct {
char name[50];
double balance;
} Customer;
// Function to add a new customer
void addCustomer(Customer customers[], int *numCustomers) {
if (*numCustomers >= MAX_CUSTOMERS) {
printf("Customer database is full. Cannot add more customers.\n");
return;
}
printf("Enter customer name: ");
scanf("%s", customers[*numCustomers].name);
printf("Enter initial balance: ");
scanf("%lf", &customers[*numCustomers].balance);
(*numCustomers)++;
printf("Customer added successfully.\n");
}
// Function to calculate the bill for a customer
void calculateBill(Customer customers[], int numCustomers) {
char customerName[50];
double amount;
printf("Enter customer name: ");
scanf("%s", customerName);
for (int i = 0; i < numCustomers; i++) {
if (strcmp(customers[i].name, customerName) == 0) {
printf("Enter the bill amount: ");
scanf("%lf", &amount);
if (amount > customers[i].balance) {
printf("Insufficient balance. Bill not paid.\n");
} else {
customers[i].balance -= amount;
printf("Bill paid successfully. Updated balance: %.2lf\n", customers[i].balance);
}
return;
}
}
printf("Customer not found.\n");
}
// Function to display customer information
void displayCustomers(Customer customers[], int numCustomers) {
printf("Customer List:\n");
for (int i = 0; i < numCustomers; i++) {
printf("Name: %s, Balance: %.2lf\n", customers[i].name, customers[i].balance);
}
}
int main() {
Customer customers[MAX_CUSTOMERS];
int numCustomers = 0;
int choice;
while (1) {
printf("\nCustomer Billing System\n");
printf("1. Add Customer\n");
printf("2. Calculate Bill\n");
printf("3. Display Customers\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addCustomer(customers, &numCustomers);
break;
case 2:
calculateBill(customers, numCustomers);
break;
case 3:
displayCustomers(customers, numCustomers);
break;
case 4:
exit(0);
default:
printf("Invalid choice. Please try again.\n");
}
}
return 0;
}
In this simplified example:
- Customers are stored in an array of
Customer
structures. - You can add customers, calculate bills, and display customer information through a menu-driven console interface.
- The system handles basic input validation and error checking.
Please note that this example does not include error handling for invalid inputs or edge cases. In a real-world application, you would need to implement more robust error handling and possibly use a data storage solution like files or a database to persist customer data.