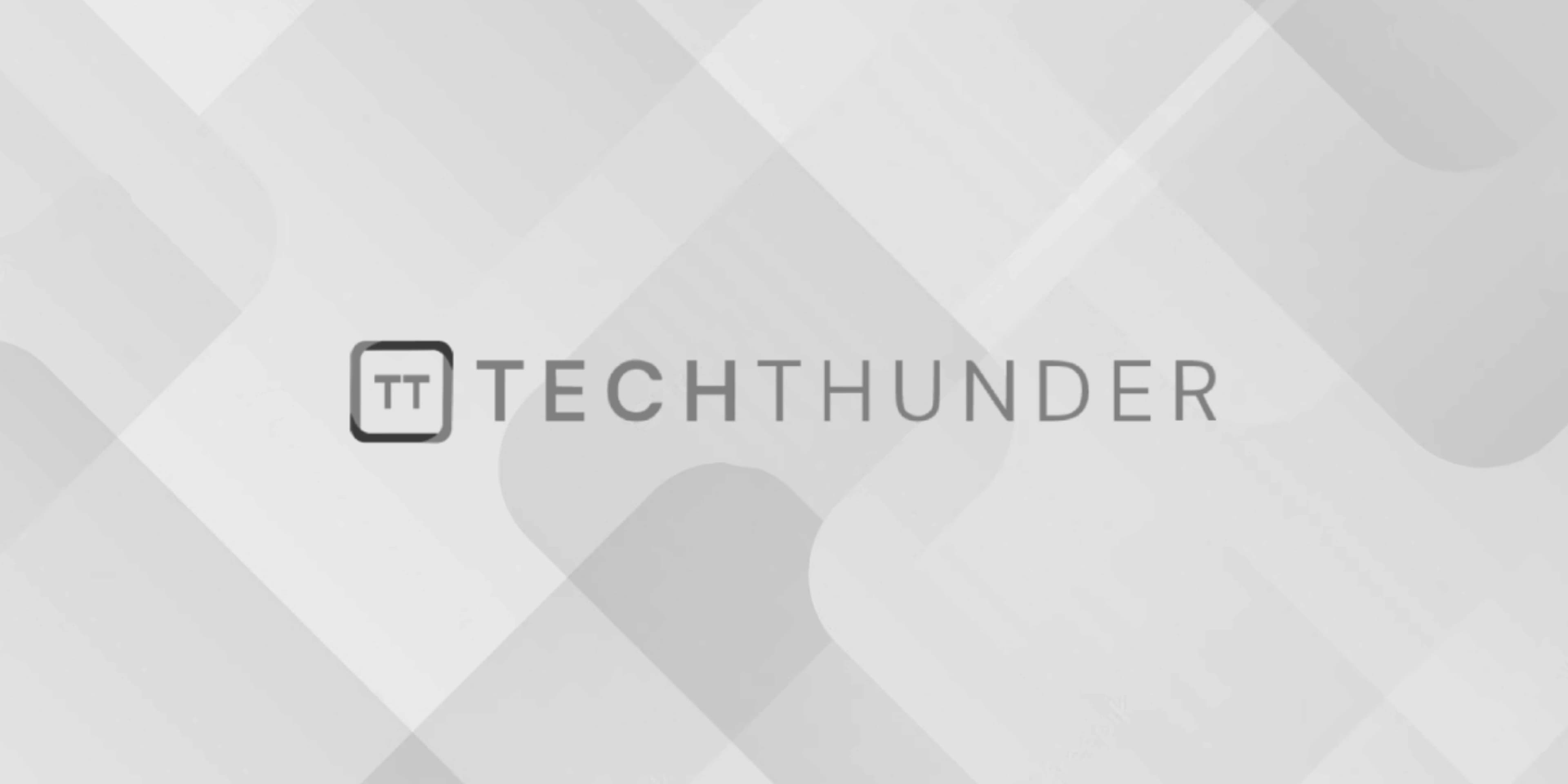
C strcpy()
The strcpy()
is a standard library function that is used to copy a source string to a destination string. It’s part of the <string.h>
header and is commonly used for string manipulation in C programs. strcpy()
is null-terminated, which means it copies characters from the source string until it reaches the null character (‘\0’), indicating the end of the string.
Here’s the syntax of strcpy()
:
char *strcpy(char *destination, const char *source);
destination
: A pointer to the destination string where the source string will be copied.source
: A pointer to the source string to be copied.
The strcpy()
function copies characters from the source
string to the destination
string until it encounters the null character in the source
string. It then adds a null character at the end of the destination
string to ensure it is null-terminated.
Here’s an example of using strcpy()
:
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!";
char destination[20]; // Make sure the destination array has enough space
strcpy(destination, source);
printf("Source string: %s\n", source);
printf("Copied string: %s\n", destination);
return 0;
}
In this example, we declare a source string (source
) and a destination string (destination
). We then use strcpy()
to copy the content of the source
string to the destination
string. After the copy operation, both strings are null-terminated.
It’s important to ensure that the destination
string has enough space to accommodate the copied characters from the source
string, including the null character. Failing to allocate enough space can lead to buffer overflow issues.
Additionally, you should be cautious when working with user-input or untrusted data, as strcpy()
does not perform bounds checking and can result in buffer overflows if the source string is longer than the destination buffer. Consider using safer functions like strncpy()
or strcpy_s()
(if available) when working with strings of unknown or potentially large lengths to prevent buffer overflows.