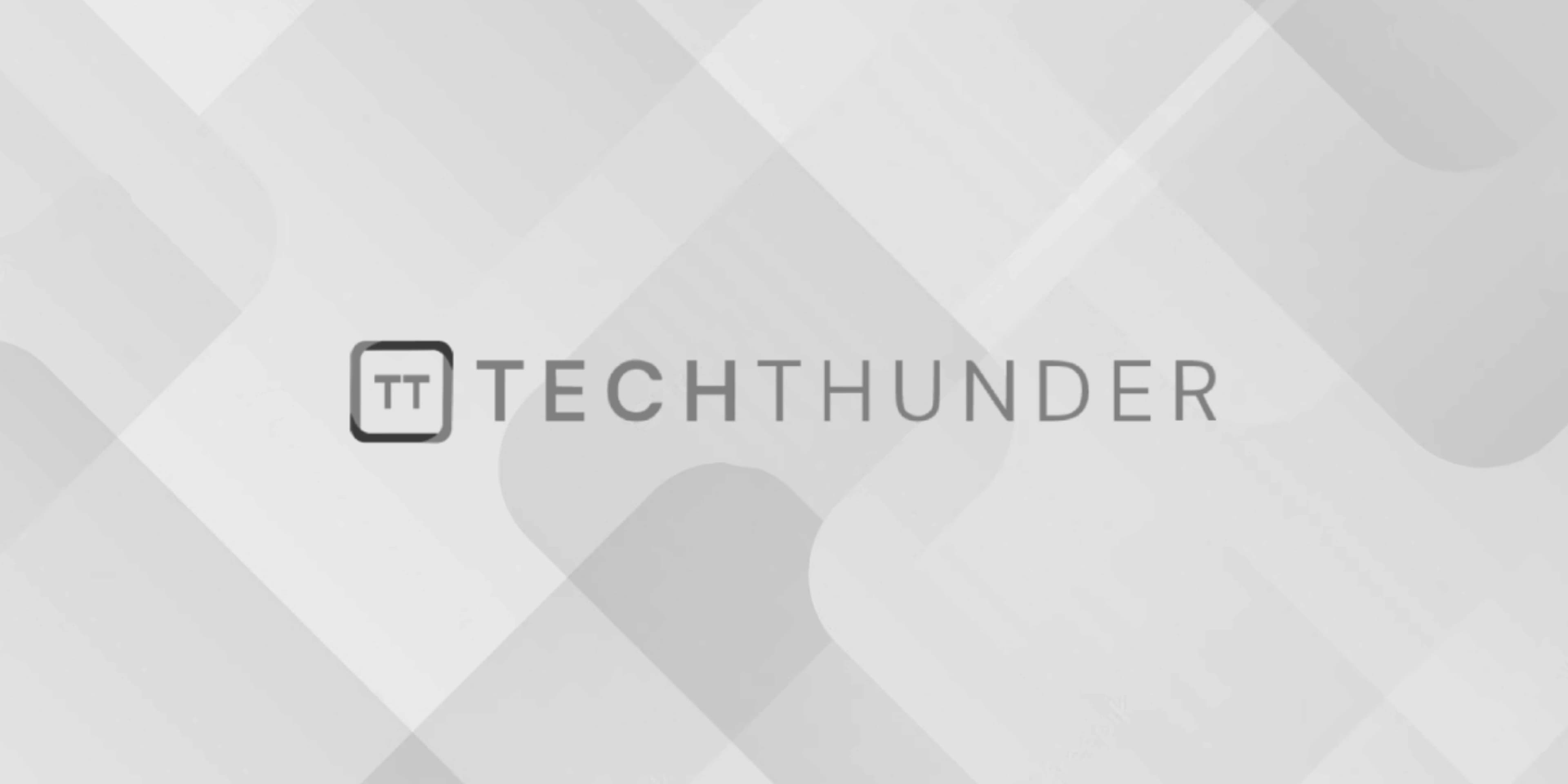
243 views
Assembly code in C
You can include assembly code using inline assembly. Inline assembly allows you to insert assembly instructions directly into your C code. However, please note that inline assembly is highly dependent on the compiler you are using, and the syntax may vary between compilers. Here’s an example of using inline assembly in C code for the x86 architecture with the GCC compiler:
C
#include <stdio.h>
int main() {
int num1 = 5, num2 = 3, result;
// Inline assembly to add num1 and num2
asm(
"add %[input1], %[input2];" // Assembly instruction
: [result] "=r" (result) // Output operand
: [input1] "r" (num1), // Input operands
[input2] "r" (num2)
);
printf("The result is: %d\n", result);
return 0;
}
In this example:
- We declare integer variables
num1
,num2
, andresult
. - We use the
asm
keyword to introduce inline assembly. Inside the inline assembly block, we write the assembly instructions to addnum1
andnum2
. - We use input and output operands specified using constraints. The
"%[input1]"
and"%[input2]"
placeholders represent the input operands, and"=r" (result)
represents the output operand. - The input and output operands are connected to C variables using the
: [result] "=r" (result)
and: [input1] "r" (num1), [input2] "r" (num2)
syntax. - Finally, we print the result.
Please note that inline assembly is compiler-specific, and the syntax may differ if you are using a different compiler or architecture. Always refer to your compiler’s documentation for specific details on inline assembly for your platform.