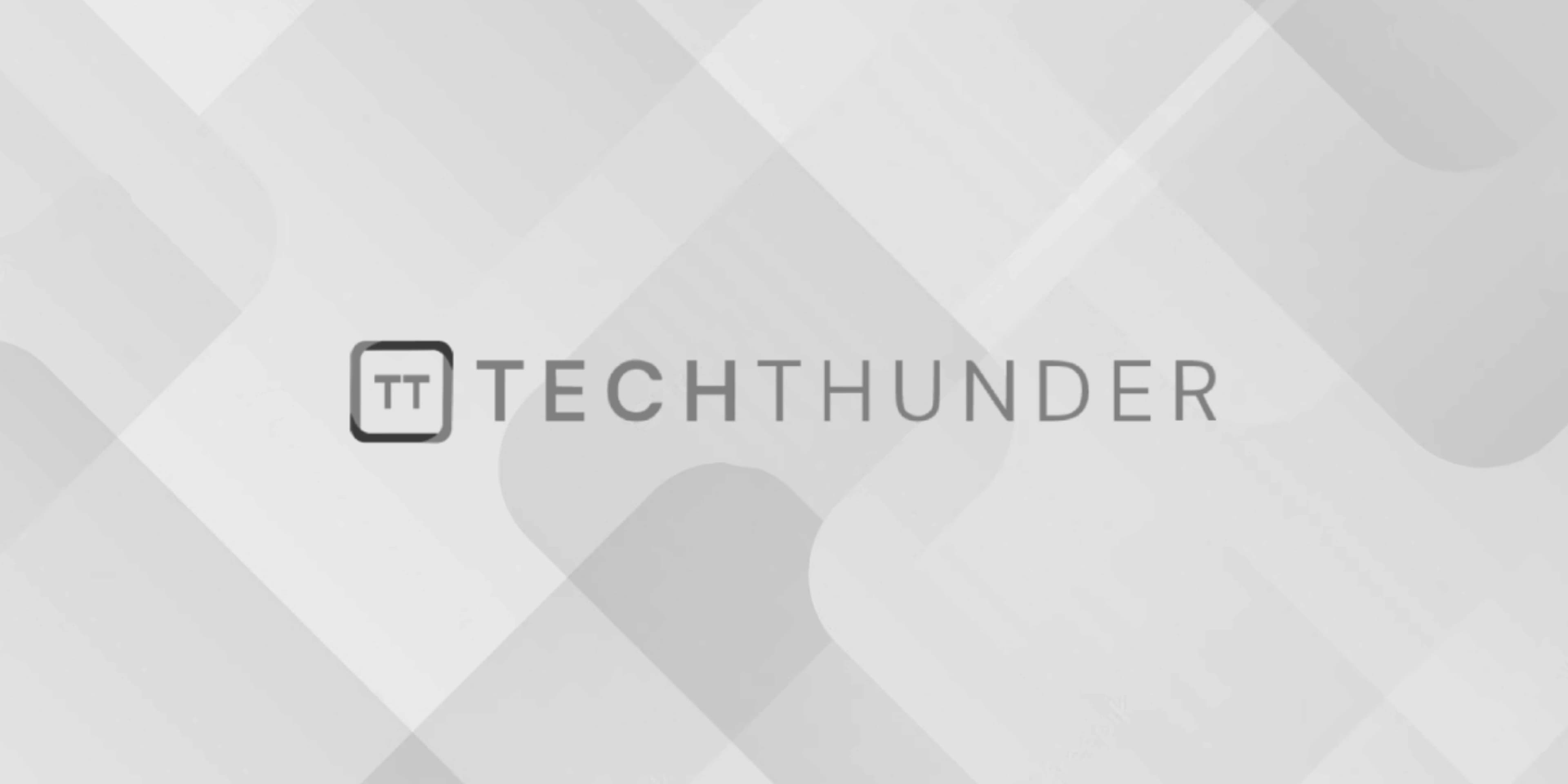
204 views
user-defined vs library function in C
The C programming, functions are essential for organizing and reusing code. Functions can be categorized into two main types: user-defined functions and library functions.
User-Defined Functions:
- Definition: User-defined functions are functions that are created by the programmer (you) to perform specific tasks within a C program.
- Declaration: You need to declare and define user-defined functions within your code. Declarations typically appear before the main function or at the beginning of your code, while the definitions contain the actual implementation of the function.
- Control: You have full control over the design, implementation, and behavior of user-defined functions. You can name them, specify their parameters, and define their functionality.
- Scope: User-defined functions are typically scoped within the file in which they are defined. However, you can make them accessible to other parts of your program by using appropriate declarations in header files.
- Examples: Here’s an example of a user-defined function:
C
#include <stdio.h>
// User-defined function to calculate the square of a number
int square(int x) {
return x * x;
}
int main() {
int result = square(5);
printf("Square of 5 is %d\n", result);
return 0;
}
Library Functions:
- Definition: Library functions are pre-written functions provided by the C Standard Library or other libraries. These functions are designed to perform common tasks and are available for use in your programs.
- Declaration: To use library functions, you need to include the appropriate header files that declare these functions. You do not need to define them because their implementations are already provided in compiled library files.
- Control: You have limited control over library functions. You can choose to use them or not, but you cannot modify their behavior or implementation.
- Scope: Library functions are available for use across different programs and are not limited to a single file. You can use them by including the corresponding header files and linking to the appropriate libraries.
- Examples: Here’s an example of using a library function to calculate the square root of a number:
C
#include <stdio.h>
#include <math.h>
int main() {
double x = 25.0;
double result = sqrt(x); // Using the sqrt() library function
printf("Square root of %lf is %lf\n", x, result);
return 0;
}
In summary, user-defined functions are functions that you create and define in your C program to perform custom tasks, while library functions are pre-existing functions provided by libraries like the C Standard Library to perform common tasks. Both types of functions are essential in C programming, and your choice of which to use depends on the specific requirements of your program.