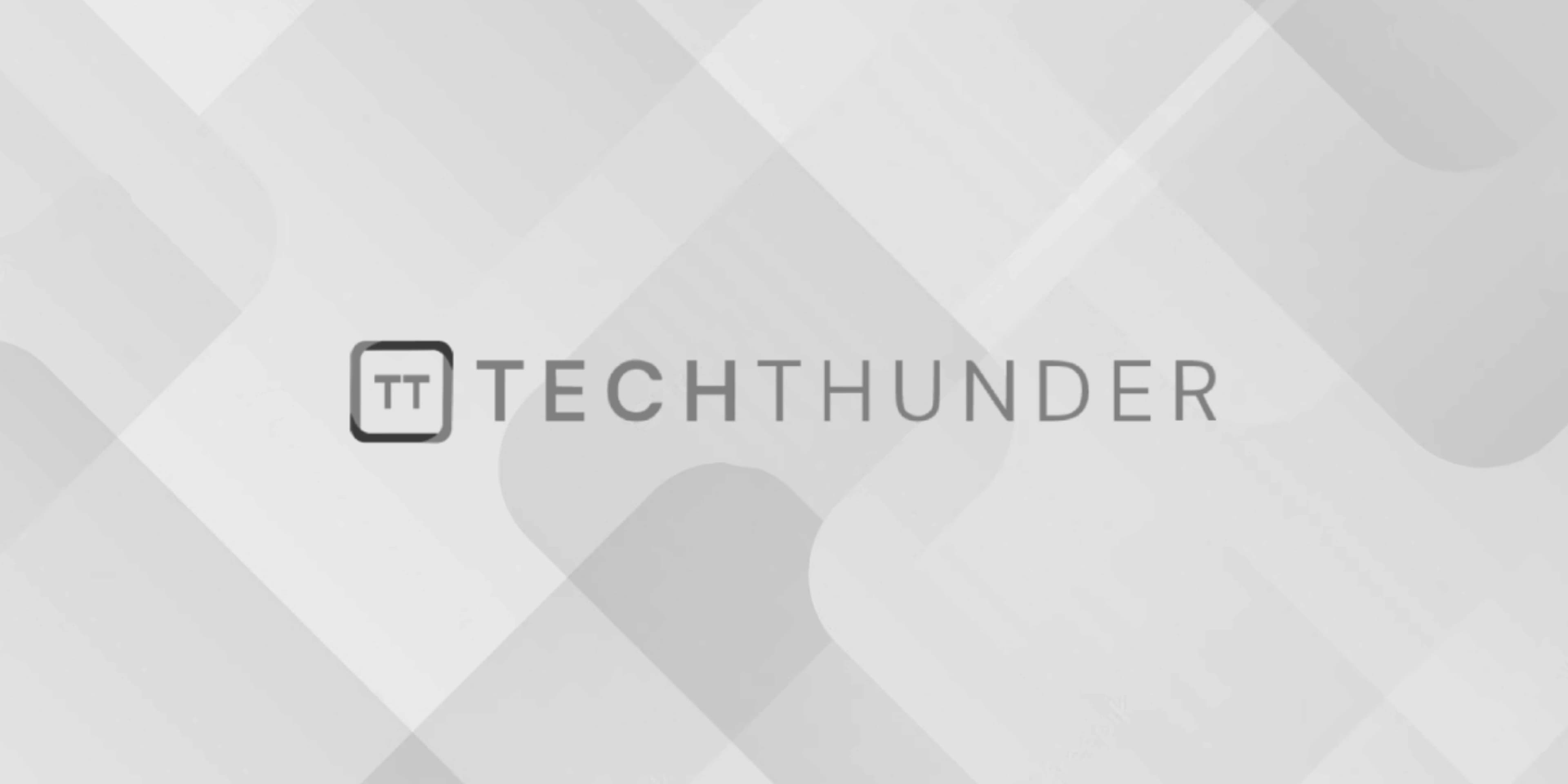
C gets() & puts()
The gets()
and puts()
are standard library functions used for reading and writing strings, respectively. However, it’s important to note that both gets()
and puts()
are considered unsafe and are generally discouraged for use in modern C programming. This is because they do not provide any mechanism to limit the input size, which can lead to buffer overflow vulnerabilities and security issues.
gets()
Function:
gets()
is used for reading a line of text from the standard input (usually the keyboard) and stores it as a string.- It reads characters until it encounters a newline character (‘\n’) or reaches the end of the input stream.
- It is considered unsafe because it does not check or limit the size of the input, which can lead to buffer overflows.
- Example:
char buffer[100];
printf("Enter a string: ");
gets(buffer); // Reads a line of text from the user
puts()
Function:
puts()
is used for writing a null-terminated string (a string with a ‘\0’ character at the end) to the standard output (usually the console).- It automatically appends a newline character (‘\n’) to the output.
- It is safer than
gets()
because it operates on null-terminated strings and does not suffer from buffer overflow issues. - Example:
char greeting[] = "Hello, world!";
puts(greeting); // Writes "Hello, world!" to the console
Note: The use of gets()
is highly discouraged because of its security risks. Instead, you should use fgets()
for reading input, which allows you to specify the maximum number of characters to read, thus preventing buffer overflows.
Here’s an example of using fgets()
and puts()
together to safely read and display a string:
#include <stdio.h>
int main() {
char buffer[100];
printf("Enter a string: ");
if (fgets(buffer, sizeof(buffer), stdin) != NULL) {
// Remove the trailing newline character if present
size_t len = strlen(buffer);
if (len > 0 && buffer[len - 1] == '\n') {
buffer[len - 1] = '\0';
}
puts("You entered:");
puts(buffer);
} else {
printf("Error reading input.\n");
}
return 0;
}
In this example, fgets()
is used to safely read a line of text from the user, and puts()
is used to display the input. The code also removes the trailing newline character added by fgets()
for better formatting.