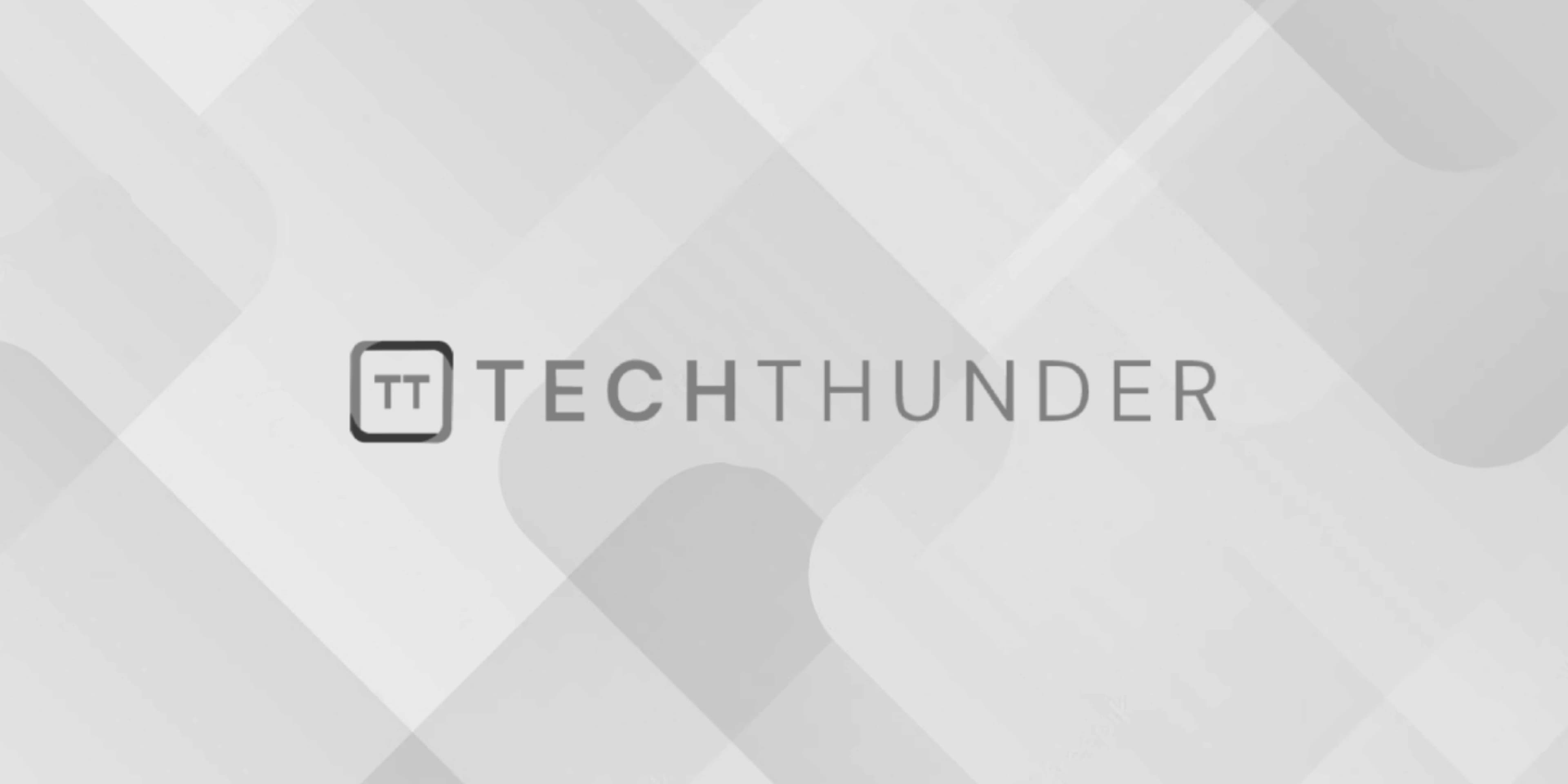
176 views
Variables in C
The variables are used to store and manipulate data within a program. Each variable has a specific data type that determines the type of data it can hold, such as integers, floating-point numbers, characters, and more. Here are the key aspects of variables in C:
Variable Declaration:
- To declare a variable, you need to specify its data type and a unique identifier (name) for the variable.
- The general syntax for declaring a variable is:
data_type variable_name;
- For example:
C
int age; // Integer variable
double salary; // Double-precision floating-point variable
char grade; // Character variable
Variable Initialization:
- You can also initialize a variable at the time of declaration by providing an initial value.
- For example:
C
int count = 0; // Initialize an integer variable
double pi = 3.14159265; // Initialize a double variable
char initial = 'A'; // Initialize a character variable
Data Types:
- C provides a variety of data types to suit different types of data. Common data types include:
int
: For integers (whole numbers).double
orfloat
: For floating-point numbers (real numbers with a decimal point).char
: For single characters.bool
: For Boolean values (true or false).long
,long long
: For larger integers.
- You can also create user-defined data types using
struct
andenum
.
Scope:
- Variables in C have scope, which defines where in the code the variable is accessible.
- A variable declared within a block (such as within a function) is typically local to that block.
- Variables declared outside of any block are typically global and can be accessed from anywhere in the program.
Lifetime:
- The lifetime of a variable refers to the duration it exists in memory.
- Local variables have a limited lifetime and are created when the block containing them is entered and destroyed when the block is exited.
- Global variables exist for the entire duration of the program.
Constants:
- In addition to variables, C allows you to define constants using the
const
keyword. Constants have fixed values that cannot be changed during program execution. - For example:
C
const double PI = 3.14159265;
Naming Conventions:
- Follow naming conventions when naming variables. It’s common to use meaningful and descriptive names that reflect the purpose of the variable.
- Variables should start with a letter or an underscore and can include letters, digits, and underscores.
- Avoid using C keywords as variable names.
Here’s an example of declaring, initializing, and using variables in C:
C
#include <stdio.h>
int main() {
int age = 30; // Declare and initialize an integer variable
double height = 175.5; // Declare and initialize a double variable
char grade = 'A'; // Declare and initialize a character variable
printf("Age: %d\n", age);
printf("Height: %.2lf\n", height); // %.2lf specifies two decimal places for a double
printf("Grade: %c\n", grade);
return 0;
}
Variables are fundamental to programming in C, and they allow you to work with and manipulate data in your programs. Understanding data types, scope, and variable naming conventions is crucial for writing correct and readable C code.