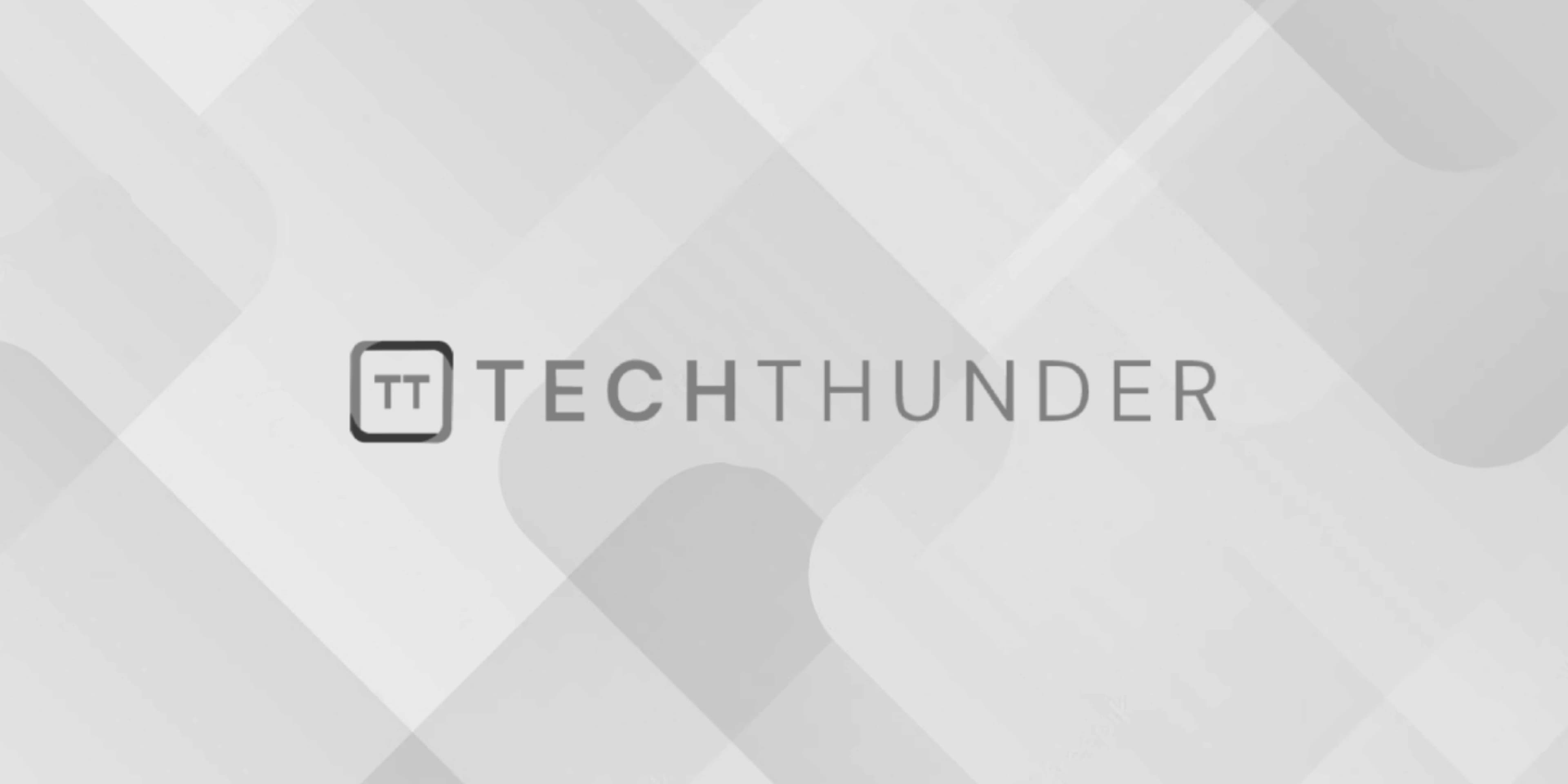
How to Add Matrix in C
Triple Data Encryption Standard (Triple DES or 3DES) is a symmetric-key block cipher algorithm that applies the Data Encryption Standard (DES) algorithm three times to each data block. It provides increased security compared to DES due to its use of multiple encryption passes. Here’s a simplified example of how to use Triple DES encryption and decryption in C using the OpenSSL library:
Make sure you have OpenSSL installed on your system. If not, you can typically install it using your system’s package manager.
#include <stdio.h>
#include <string.h>
#include <openssl/des.h>
// Function to perform Triple DES encryption
void des3_encrypt(const unsigned char *plaintext, unsigned char *ciphertext, DES_key_schedule ks1, DES_key_schedule ks2, DES_key_schedule ks3) {
DES_ecb3_encrypt(plaintext, ciphertext, &ks1, &ks2, &ks3, DES_ENCRYPT);
}
// Function to perform Triple DES decryption
void des3_decrypt(const unsigned char *ciphertext, unsigned char *plaintext, DES_key_schedule ks1, DES_key_schedule ks2, DES_key_schedule ks3) {
DES_ecb3_encrypt(ciphertext, plaintext, &ks1, &ks2, &ks3, DES_DECRYPT);
}
int main() {
DES_cblock key1, key2, key3;
DES_key_schedule ks1, ks2, ks3;
unsigned char plaintext[] = "Hello123"; // 8 bytes
unsigned char ciphertext[8];
unsigned char decryptedtext[8];
// Initialize the keys (each key is 8 bytes)
memset(key1, 0, sizeof(DES_cblock));
memset(key2, 0, sizeof(DES_cblock));
memset(key3, 0, sizeof(DES_cblock));
// Set your keys here (e.g., using random bytes or your own keys)
// Replace these zeros with your actual keys
// Example:
// strncpy((char *)key1, "mykey123", 8);
// strncpy((char *)key2, "yourkey1", 8);
// strncpy((char *)key3, "secret12", 8);
// Generate key schedules
DES_set_key_unchecked(&key1, &ks1);
DES_set_key_unchecked(&key2, &ks2);
DES_set_key_unchecked(&key3, &ks3);
// Encrypt the plaintext
des3_encrypt(plaintext, ciphertext, ks1, ks2, ks3);
// Decrypt the ciphertext
des3_decrypt(ciphertext, decryptedtext, ks1, ks2, ks3);
printf("Plaintext: %s\n", plaintext);
printf("Ciphertext: ");
for (int i = 0; i < 8; i++) {
printf("%02x ", ciphertext[i]);
}
printf("\n");
printf("Decrypted text: %s\n", decryptedtext);
return 0;
}
In this example:
- We include the necessary OpenSSL headers for DES encryption.
- We initialize the keys
key1
,key2
, andkey3
, and set them to your desired values (replace the zeros with your actual keys). - We generate key schedules
ks1
,ks2
, andks3
usingDES_set_key_unchecked
. - We encrypt the
plaintext
usingdes3_encrypt
and decrypt theciphertext
usingdes3_decrypt
. - Finally, we print the plaintext, ciphertext in hexadecimal format, and the decrypted text.
Compile this program with OpenSSL’s development libraries and run it to see Triple DES encryption and decryption in action. Ensure that you handle keys securely and adjust the key management part according to your security requirements.