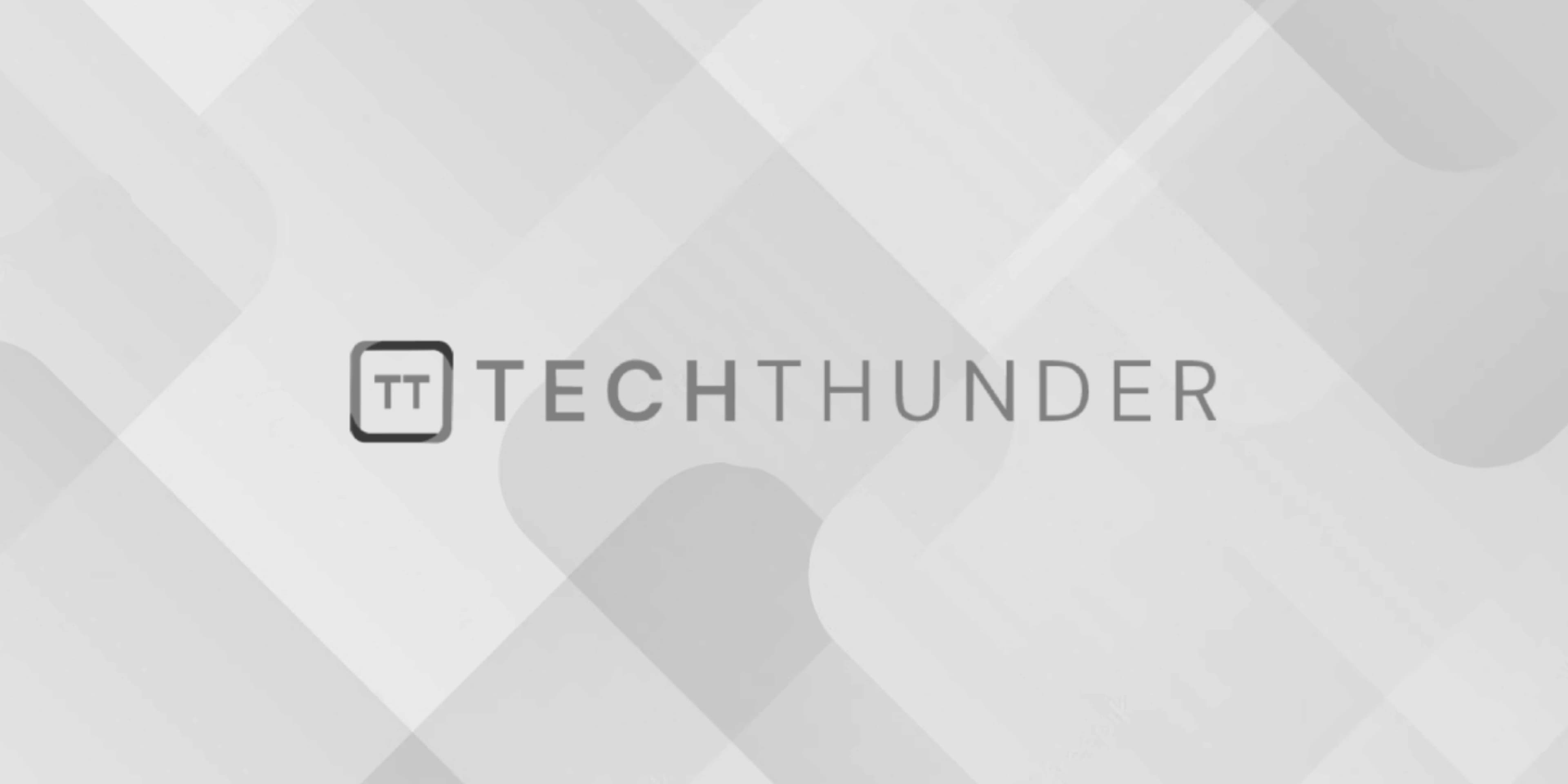
577 views
Table Program in C
A table program in C typically involves generating and displaying a multiplication or any other kind of table. Here’s a simple example of a multiplication table program in C that generates and displays a multiplication table for a given number:
C
#include <stdio.h>
int main() {
int number, i;
// Input the number for which you want to generate the multiplication table
printf("Enter an integer: ");
scanf("%d", &number);
// Display the multiplication table for the input number
printf("Multiplication Table for %d:\n", number);
for (i = 1; i <= 10; i++) {
printf("%d x %d = %d\n", number, i, number * i);
}
return 0;
}
In this program:
- We prompt the user to input an integer for which they want to generate the multiplication table.
- We use a
for
loop to iterate from 1 to 10 (you can adjust the range as needed). - Inside the loop, we calculate and display the product of the input number and the loop variable to generate the multiplication table.
Here’s how the program works:
Plaintext
Enter an integer: 5
Multiplication Table for 5:
5 x 1 = 5
5 x 2 = 10
5 x 3 = 15
5 x 4 = 20
5 x 5 = 25
5 x 6 = 30
5 x 7 = 35
5 x 8 = 40
5 x 9 = 45
5 x 10 = 50
You can modify this program to generate tables for different operations (e.g., addition, subtraction, division) or for different numbers and ranges as needed.