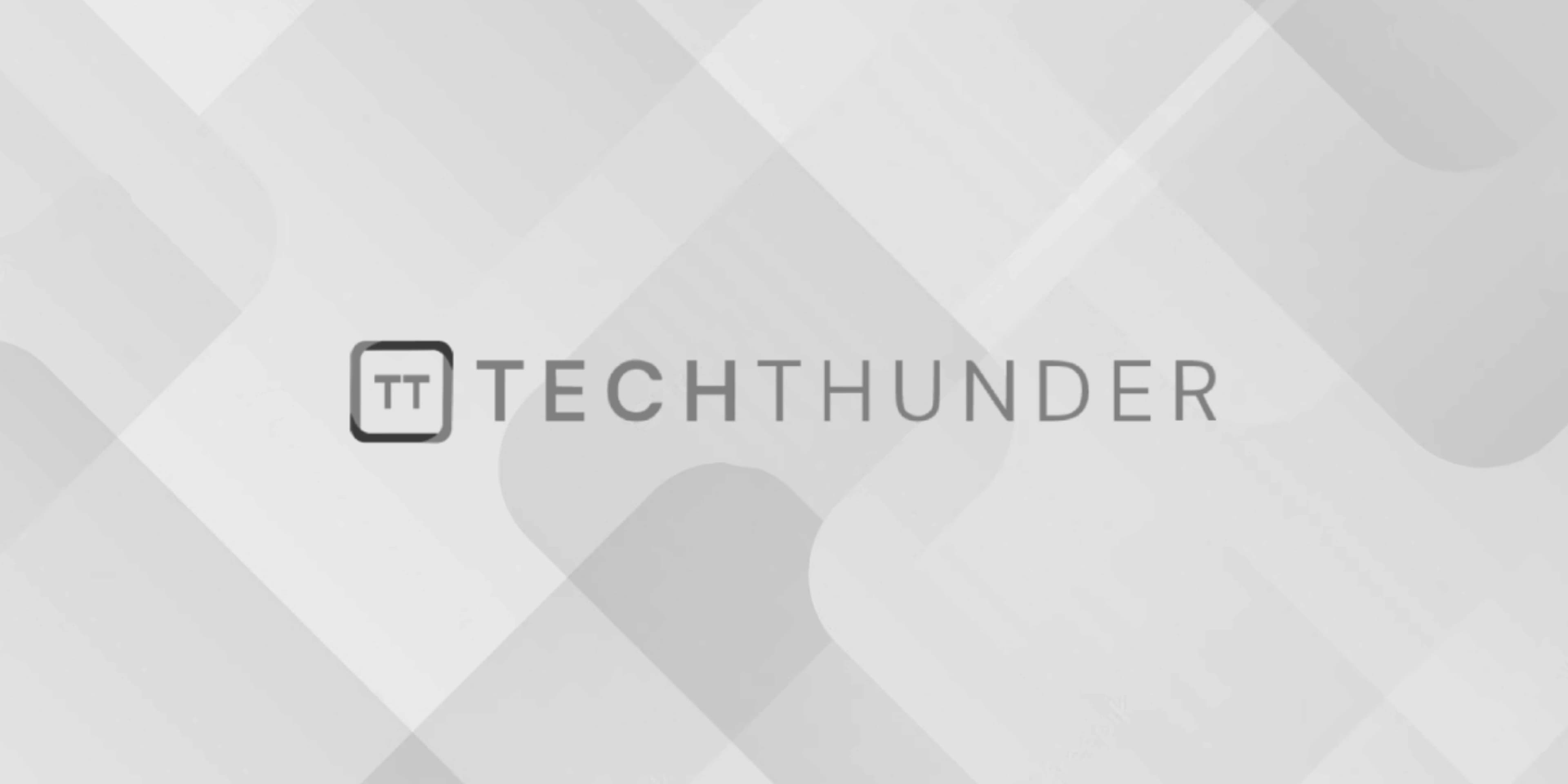
Floor() Function in C
The floor()
function is part of the math library (math.h
). It is used to round a floating-point number down to the nearest integer value that is less than or equal to the original number. The floor()
function returns a double
value.
Here’s the prototype of the floor()
function:
#include <math.h>
double floor(double x);
x
: The floating-point number that you want to round down.
The floor()
function effectively truncates the fractional part of the floating-point number, leaving only the integer part. It always rounds towards negative infinity.
Here’s an example of how to use the floor()
function:
#include <stdio.h>
#include <math.h>
int main() {
double num = 7.9;
double result = floor(num);
printf("Original number: %.2f\n", num);
printf("Floor value: %.2f\n", result);
return 0;
}
In this example, we use the floor()
function to round down the number 7.9
, and the result is 7.0
.
The floor()
function is often used in various mathematical and numerical applications where you need to round down a floating-point value to the nearest integer value.