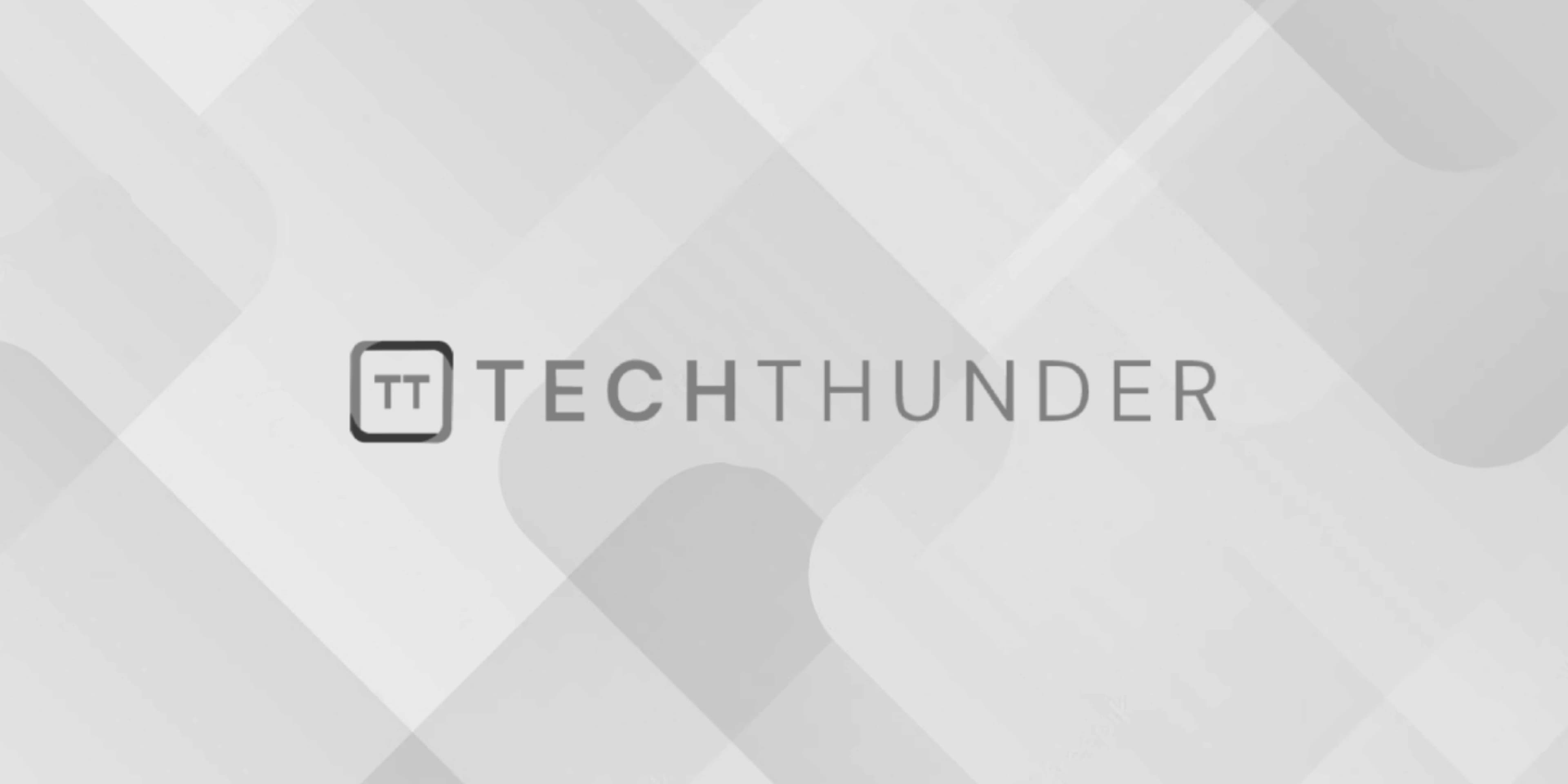
C #pragma
The #pragma
is a preprocessor directive that allows you to give special instructions to the compiler or control specific compiler behaviors. #pragma
directives are not part of the C standard itself; instead, they are compiler-specific and may vary between different compilers. However, some #pragma
directives are commonly supported by many C compilers. Below are some common uses of #pragma
directives:
- Pragma Once:
#pragma once
This directive is used to ensure that a header file is included only once during compilation. It’s a compiler-specific way to prevent multiple inclusions of the same header file and serves as an alternative to traditional inclusion guards (e.g., #ifndef
and #define
).
- Pragma Message:
#pragma message("This is a custom compile-time message.")
It instructs the compiler to display a custom message during compilation. This can be useful for adding comments or notes to your code that are only visible during compilation.
- Pragma Warning and Pragma Error:
#pragma warning(disable: warning_number)
#pragma warning(error: warning_number)
These directives allow you to control compiler warnings and errors at a fine-grained level. You can disable specific warnings or turn them into errors using these directives. The warning_number
corresponds to the specific warning you want to control.
- Pragma Pack:
#pragma pack(push, n)
#pragma pack(pop)
These directives control structure padding and alignment. You can use #pragma pack(push, n)
to set the packing alignment to n
bytes and #pragma pack(pop)
to restore the previous packing alignment. This is useful when dealing with binary file formats or interfacing with hardware.
- Pragma Comment:
#pragma comment(lib, "library_name")
This directive is used to embed comments in the object file or specify additional libraries to be linked with your program. It’s often used to specify external libraries that your program depends on.
- Pragma Linker:
#pragma comment(linker, "/SUBSYSTEM:WINDOWS")
This directive allows you to specify linker options or settings. For example, it can be used to specify the subsystem for a Windows application.
Keep in mind that the availability and behavior of #pragma
directives can vary between compilers. It’s essential to consult your compiler’s documentation to understand the specific #pragma
directives it supports and their usage. Additionally, using #pragma
directives should be done judiciously, as they may make your code less portable across different compilers.