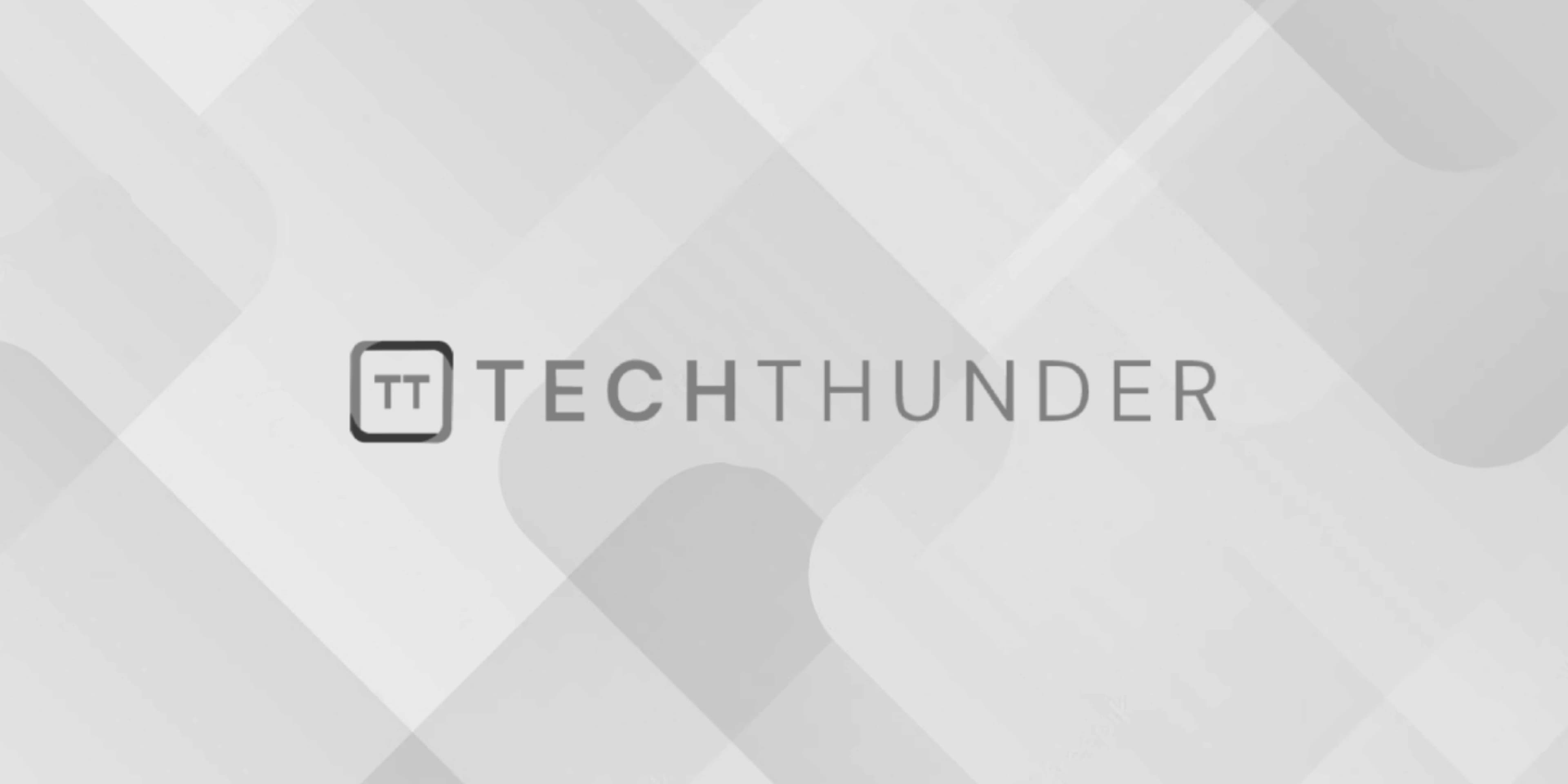
202 views
Command Line Arguments in C
Command line arguments in C allow you to pass information to your C program when you run it from the command line. These arguments are passed as strings and can be used to customize the behavior of your program. Here’s how you can work with command line arguments in C:
C
#include <stdio.h>
int main(int argc, char *argv[]) {
// argc is the count of command line arguments (including the program name)
// argv is an array of strings containing the arguments
// Check if there are at least two arguments (including the program name)
if (argc < 3) {
printf("Usage: %s <arg1> <arg2>\n", argv[0]);
return 1; // Exit with an error code
}
// Access and print the command line arguments
printf("Program name: %s\n", argv[0]);
printf("First argument: %s\n", argv[1]);
printf("Second argument: %s\n", argv[2]);
return 0; // Exit with a success code
}
In this example:
- The
main
function takes two parameters:argc
andargv
.argc
(argument count) is an integer that represents the number of command line arguments, including the program name itself.argv
(argument vector) is an array of strings, where each element is a command line argument. - We first check if there are at least three arguments (including the program name) by comparing
argc
with 3. If not, we print a usage message and return an error code (1) to indicate that the program was used incorrectly. - We access and print the program name using
argv[0]
and the first and second arguments usingargv[1]
andargv[2]
, respectively. - Finally, we return 0 to indicate successful execution or a non-zero value to indicate an error.
To compile and run this program, you can use a command like this:
Bash
gcc program.c -o program
./program arg1 arg2
Replace program.c
with the name of your C source file and program
with the desired output executable name. arg1
and arg2
can be replaced with any arguments you want to pass to the program.