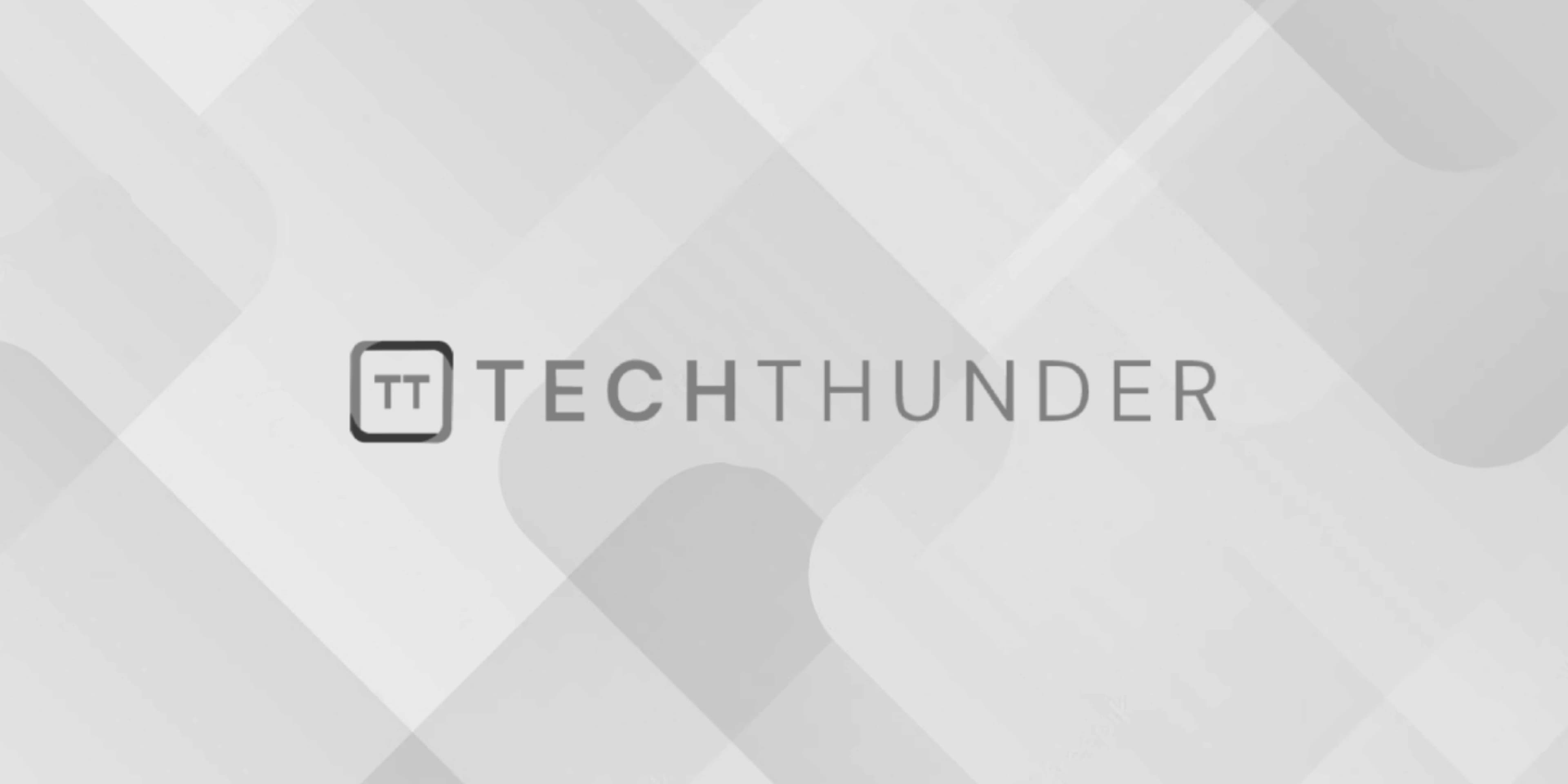
212 views
C Program to Convert Infix to Postfix
Converting an infix expression to postfix notation can be accomplished using a stack data structure to maintain the order of operators. Here’s a C program to convert an infix expression to postfix notation:
C
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
// Define the maximum size of the expression and stack
#define MAX_EXPRESSION_SIZE 100
#define MAX_STACK_SIZE 100
// Stack data structure
struct Stack {
int top;
char items[MAX_STACK_SIZE];
};
// Function to initialize an empty stack
void initialize(struct Stack *stack) {
stack->top = -1;
}
// Function to check if the stack is empty
bool isEmpty(struct Stack *stack) {
return stack->top == -1;
}
// Function to check the precedence of an operator
int precedence(char operator) {
switch (operator) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
default:
return 0;
}
}
// Function to push an item onto the stack
void push(struct Stack *stack, char item) {
if (stack->top == MAX_STACK_SIZE - 1) {
printf("Stack Overflow\n");
exit(1);
}
stack->items[++stack->top] = item;
}
// Function to pop an item from the stack
char pop(struct Stack *stack) {
if (isEmpty(stack)) {
printf("Stack Underflow\n");
exit(1);
}
return stack->items[stack->top--];
}
// Function to convert infix to postfix
void infixToPostfix(char infix[], char postfix[]) {
struct Stack stack;
initialize(&stack);
int i, j = 0;
for (i = 0; infix[i]; i++) {
char token = infix[i];
if (token >= '0' && token <= '9') {
postfix[j++] = token;
} else if (token == '(') {
push(&stack, token);
} else if (token == ')') {
while (!isEmpty(&stack) && stack.items[stack.top] != '(') {
postfix[j++] = pop(&stack);
}
if (!isEmpty(&stack) && stack.items[stack.top] == '(') {
pop(&stack);
}
} else {
while (!isEmpty(&stack) && precedence(token) <= precedence(stack.items[stack.top])) {
postfix[j++] = pop(&stack);
}
push(&stack, token);
}
}
while (!isEmpty(&stack)) {
postfix[j++] = pop(&stack);
}
postfix[j] = '\0';
}
int main() {
char infix[MAX_EXPRESSION_SIZE];
char postfix[MAX_EXPRESSION_SIZE];
printf("Enter an infix expression: ");
fgets(infix, sizeof(infix), stdin);
infix[strlen(infix) - 1] = '\0'; // Remove newline character
infixToPostfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
return 0;
}
This C program reads an infix expression from the user, converts it to postfix notation, and then prints the postfix expression. The program uses a stack to keep track of operators while scanning the input expression and follows the rules of operator precedence.
To use the program, compile it and run it. Enter an infix expression when prompted, and it will provide the corresponding postfix expression as output.