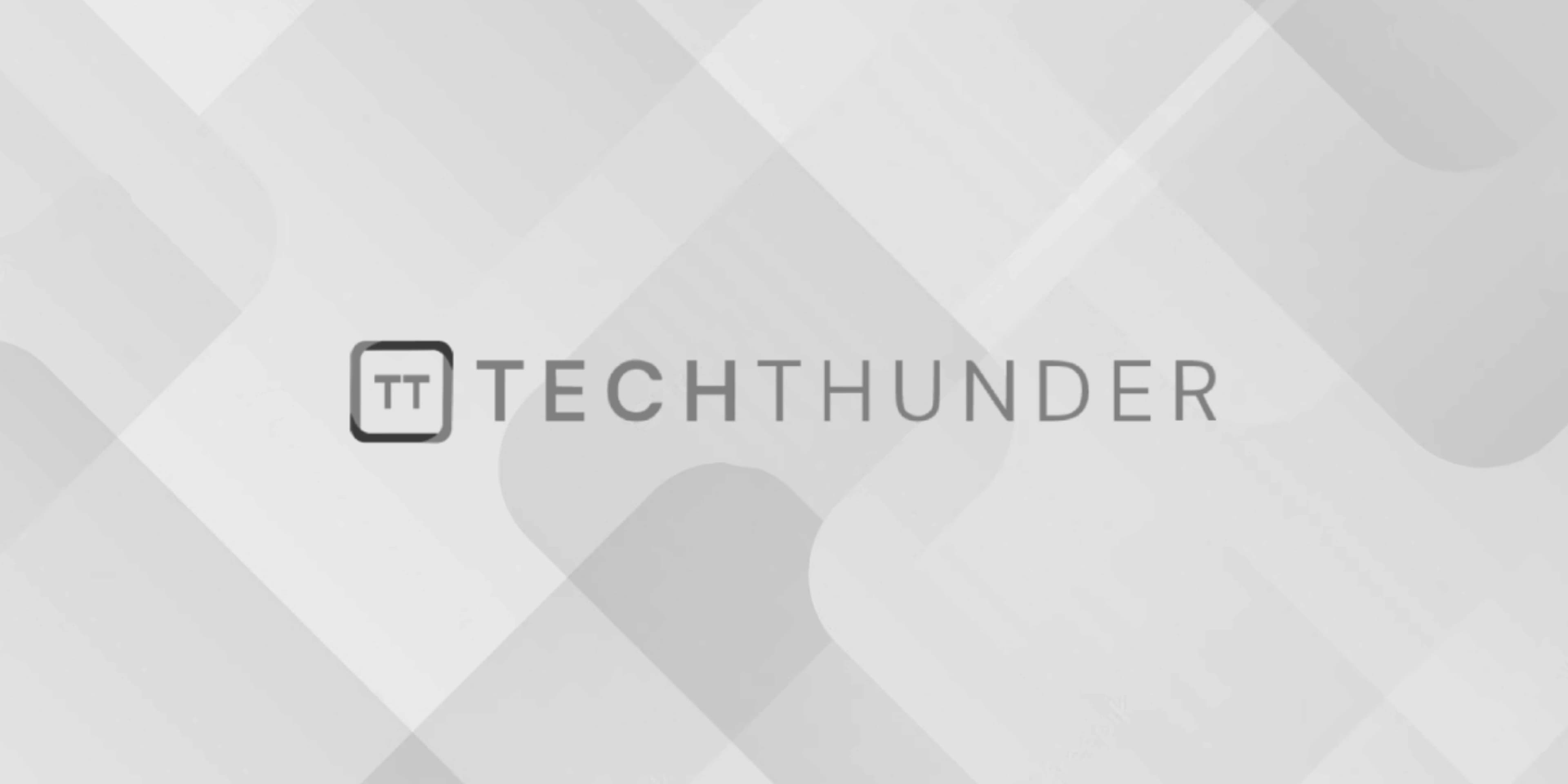
Types of Recursion in C
The recursion is a technique where a function calls itself either directly or indirectly. There are two main types of recursion: direct (or single) recursion and indirect (or mutual) recursion.
- Direct Recursion:
- Direct recursion occurs when a function calls itself directly from within its own body.
- In a directly recursive function, there is typically a base case or termination condition that prevents the recursion from continuing indefinitely. Without a base case, the recursion can lead to a stack overflow and crash the program.
- Direct recursion is the most common type of recursion. Example of direct recursion:
#include <stdio.h>
void countDown(int n) {
if (n <= 0) {
return; // Base case: Stop recursion
}
printf("%d ", n);
countDown(n - 1); // Recursive call with a smaller value
}
int main() {
countDown(5);
return 0;
}
In this example, the countDown
function calls itself directly with a smaller value until it reaches the base case (when n
becomes less than or equal to 0), at which point the recursion stops.
- Indirect Recursion (Mutual Recursion):
- Indirect recursion occurs when two or more functions call each other in a circular manner, forming a cycle of function calls.
- Each function in the cycle calls another function, which eventually leads back to the initial function, creating a loop.
- Like direct recursion, indirect recursion also requires a base case or termination condition to prevent infinite recursion. Example of indirect recursion:
#include <stdio.h>
// Function prototypes
void function1(int n);
void function2(int n);
void function1(int n) {
if (n <= 0) {
return; // Base case: Stop recursion
}
printf("%d ", n);
function2(n - 1); // Call function2
}
void function2(int n) {
if (n <= 0) {
return; // Base case: Stop recursion
}
printf("%d ", n);
function1(n - 1); // Call function1
}
int main() {
function1(5);
return 0;
}
In this example, function1
and function2
call each other in a circular manner until the base case is reached in one of them.
Recursion is a powerful programming technique, but it should be used carefully. Recursive functions should have well-defined base cases to avoid infinite recursion, and the depth of recursion should be reasonable to prevent stack overflow errors.