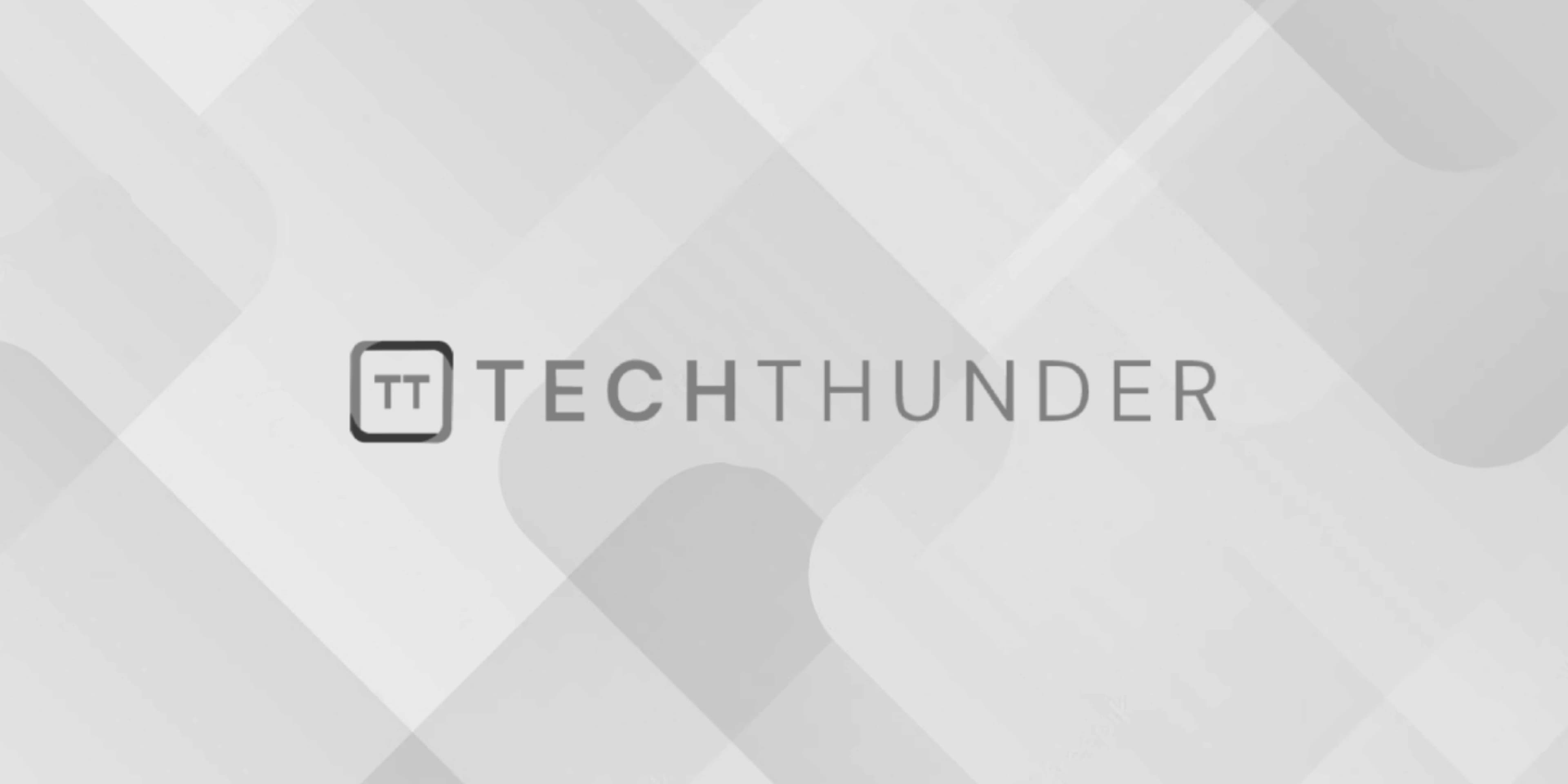
176 views
C program to compare the two strings
To compare two strings in C, you can use the strcmp
function from the standard library. The strcmp
function compares two strings character by character and returns an integer value indicating their relationship. Here’s a C program to compare two strings:
C
#include <stdio.h>
#include <string.h>
int main() {
char str1[100], str2[100];
printf("Enter the first string: ");
scanf("%s", str1);
printf("Enter the second string: ");
scanf("%s", str2);
// Compare the two strings using strcmp
int result = strcmp(str1, str2);
if (result == 0) {
printf("The two strings are equal.\n");
} else if (result < 0) {
printf("The first string is lexicographically less than the second string.\n");
} else {
printf("The first string is lexicographically greater than the second string.\n");
}
return 0;
}
In this program:
- We declare two character arrays
str1
andstr2
to store the input strings. - We use
printf
andscanf
to input both strings from the user. - We compare the two strings using
strcmp
. Thestrcmp
function returns 0 if the strings are equal, a negative value if the first string is lexicographically less than the second, and a positive value if the first string is lexicographically greater than the second. - We check the result of
strcmp
and print an appropriate message to indicate the relationship between the two strings.
Compile and run the program, and it will compare the two input strings and display the result of the comparison.