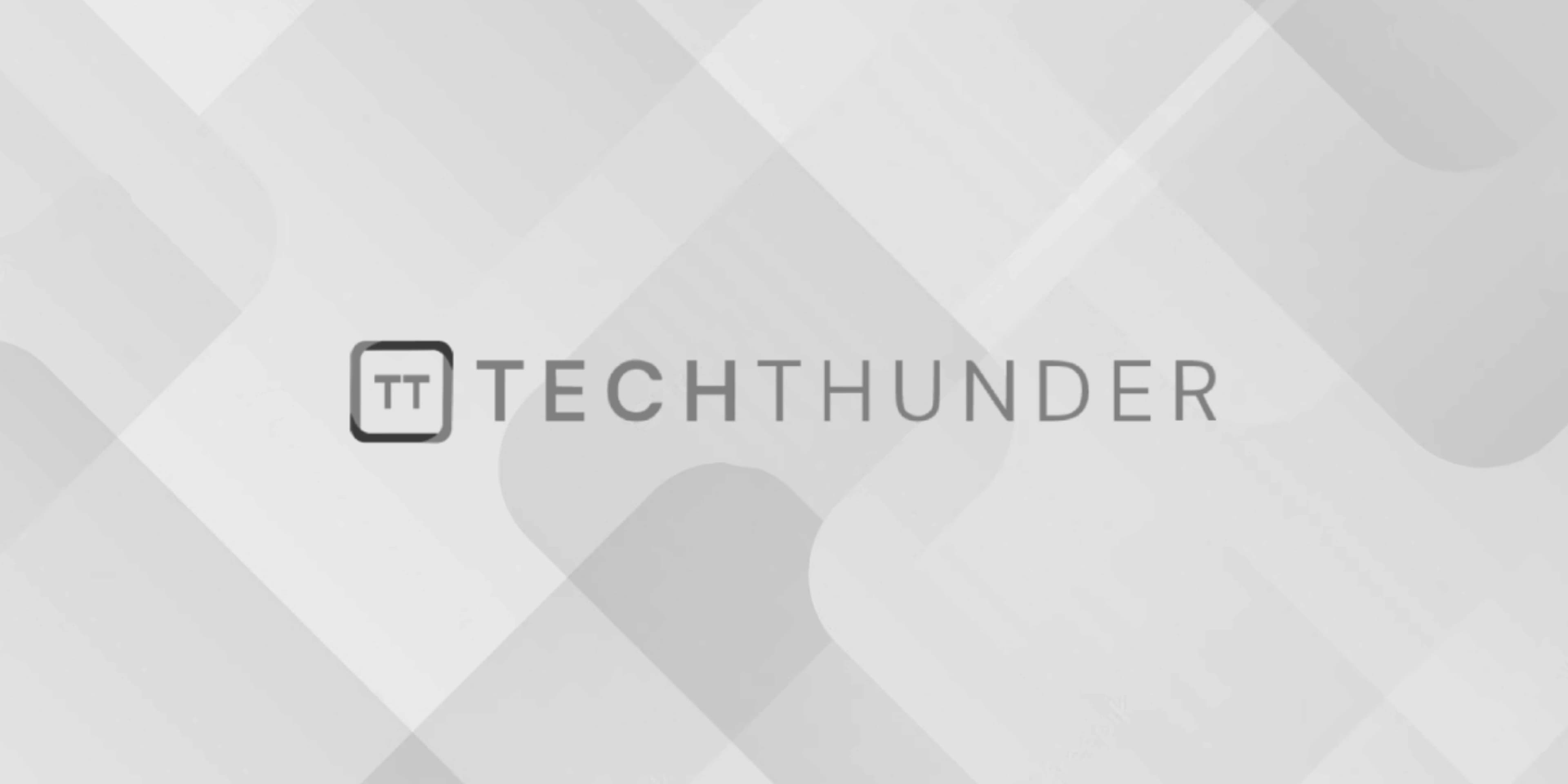
C Preprocessor
The C preprocessor is a tool that runs before the actual compilation of a C program. It processes the source code before it’s compiled and performs various tasks like macro substitution, conditional compilation, and inclusion of header files. The preprocessor is a part of the C compiler and is typically invoked automatically when you compile a C program.
Here are some of the most commonly used preprocessor directives and their purposes:
Include Files (#include
)
#include
is used to include the contents of other files into your source code.- Commonly used to include standard library headers or your own header files.
- Example:
#include <stdio.h>
Macro Definitions (#define
)
#define
is used to create macros, which are symbolic names representing expressions or code fragments.- Macros are often used for constants or to create reusable code snippets.
- Example:
#define PI 3.14159
Conditional Compilation (#ifdef
, #ifndef
, #if
, #else
, #elif
, #endif
)
- Conditional directives allow you to compile or exclude code based on certain conditions.
- Useful for creating platform-specific code or enabling/disabling features.
- Example:
c #ifdef DEBUG printf("Debug mode is enabled.\n"); #endif
File Inclusion Guards
- These are used to prevent multiple inclusions of the same header file.
- Commonly used to avoid issues with redefinition of symbols.
- Example:
c #ifndef MY_HEADER_H #define MY_HEADER_H // Header contents go here #endif
Stringize Operator (#
) and Token Pasting Operator (##
)
#
is used to convert a token into a string.##
is used for token pasting, where you can concatenate tokens.- Example:
c #define STRINGIZE(x) #x #define CONCAT(x, y) x ## y
Pragma Directives (#pragma
)
#pragma
is used for compiler-specific instructions.- Allows you to control various aspects of compilation.
- Example:
#pragma warning(disable: 1234)
Line Control (#line
)
#line
is used to control the line numbers reported by the compiler during error messages.- It can be useful for debugging or generating code from other sources.
Assertions (#assert
)
#assert
is used to conditionally test a condition at compile-time and generate an error if it’s false.
The preprocessor directives are processed in a single pass before the code is compiled. They are identified by the #
symbol at the beginning of a line. The preprocessor’s primary goal is to prepare the source code for compilation by replacing macros, including header files, and handling conditional compilation based on the directives you provide.
For example, when you use #include
, the preprocessor replaces that directive with the content of the included file. Similarly, when you use #define
, it defines a macro that will be substituted throughout the code.
Remember that the C preprocessor does not understand C syntax; it operates on text substitution and basic conditional logic.