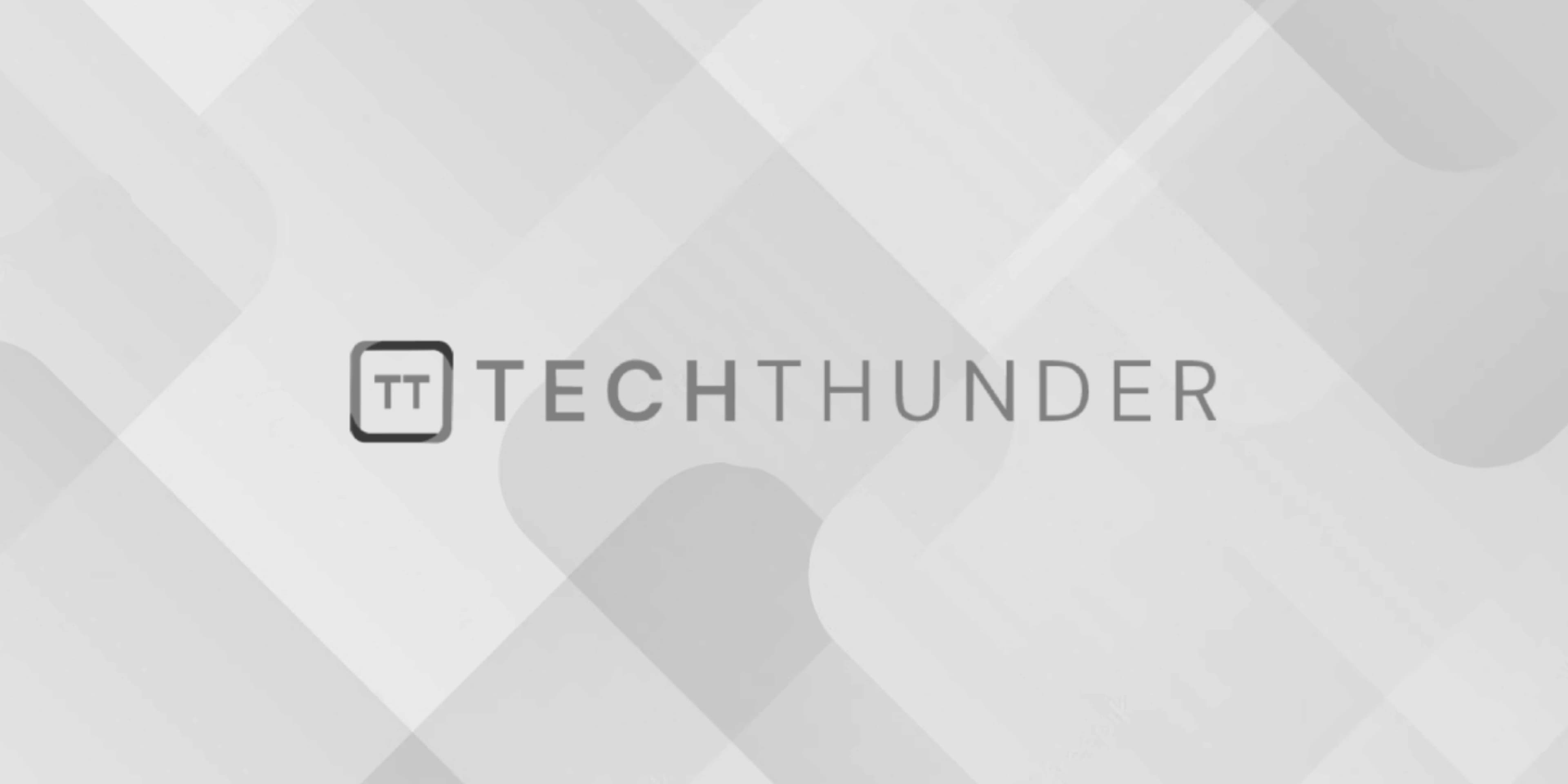
C do-while loop
The do-while
loop is a control flow structure in C that allows you to execute a block of code repeatedly as long as a specified condition remains true. Unlike the while
loop, which tests the condition before the loop body is executed, the do-while
loop tests the condition after the loop body has executed at least once. This guarantees that the loop body is executed at least once, even if the condition is initially false.
Here’s the syntax of the do-while
loop in C:
do {
// Loop body
} while (condition);
- The
do
keyword marks the beginning of the loop. - The loop body contains the code you want to execute repeatedly.
- The
while
keyword is followed by the condition that is tested after each execution of the loop body. - The loop body is enclosed in curly braces
{}
if it consists of multiple statements.
Here’s an example of using a do-while
loop to repeatedly prompt the user for a positive number until a valid input is provided:
#include <stdio.h>
int main() {
int number;
do {
printf("Enter a positive number: ");
scanf("%d", &number);
if (number <= 0) {
printf("Invalid input. Please enter a positive number.\n");
}
} while (number <= 0);
printf("You entered a valid positive number: %d\n", number);
return 0;
}
In this example:
- The program first asks the user to enter a positive number.
- The input is stored in the
number
variable. - The
do-while
loop checks if thenumber
is less than or equal to 0. - If the condition is true (i.e., the input is not positive), it displays an error message and repeats the loop.
- The loop continues until the user enters a valid positive number.
The do-while
loop ensures that the user is prompted at least once, even if the initial input is not valid. This makes it useful for situations where you want to guarantee the execution of a block of code before checking the condition.