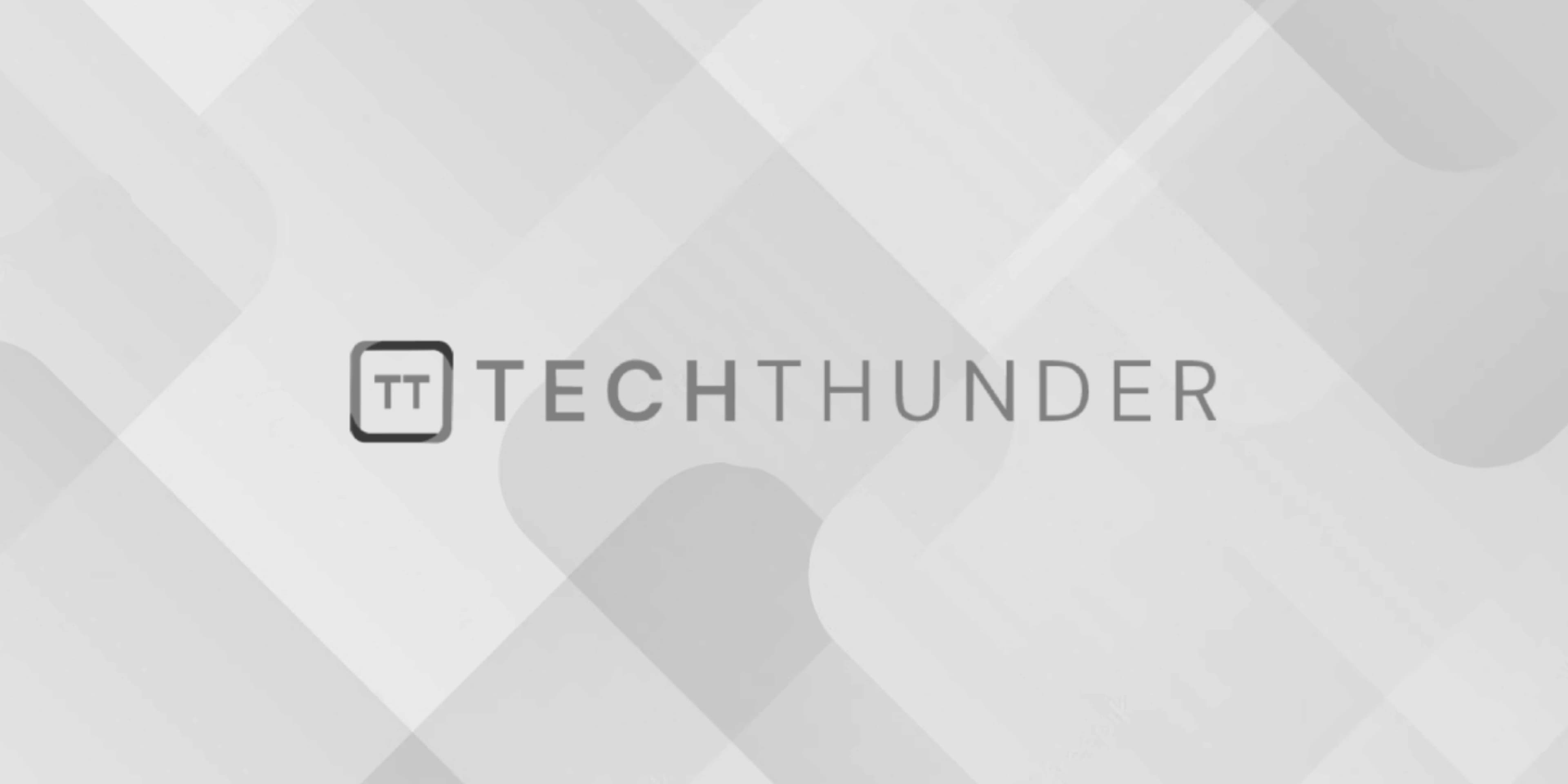
311 views
C if-else vs switch
The choice between using if-else
statements and the switch
statement in C depends on the specific requirements of your program and your coding preferences. Both constructs are used for making decisions and branching in your code, but they have different use cases and advantages.
Use if-else
When:
- Multiple Conditions with Different Expressions: If you have multiple conditions, each requiring different expressions or actions,
if-else
is more flexible. You can specify different expressions for eachif
andelse if
condition.
C
if (condition1) {
// Code for condition1
} else if (condition2) {
// Code for condition2
} else {
// Default code
}
- Non-Constant Conditions:
if-else
allows you to evaluate non-constant conditions, making it suitable when conditions are based on runtime values or user input.
C
if (user_choice == 1) {
// Code for choice 1
} else if (user_choice == 2) {
// Code for choice 2
} else {
// Default code
}
- Range Checking:
if-else
is effective for range checking or when conditions involve complex expressions.
C
if (x > 10 && x < 20) {
// Code for x in the range (10, 20)
} else if (x >= 20 && x < 30) {
// Code for x in the range [20, 30)
} else {
// Default code
}
Use switch
When:
- Single Value Testing:
switch
is ideal for cases where you need to test a single value against multiple constant values.
C
switch (choice) {
case 1:
// Code for choice 1
break;
case 2:
// Code for choice 2
break;
default:
// Default code
}
- Falling Through:
switch
allows for cases to “fall through” to execute the same code for multiple values without explicitly repeating it.
C
switch (day) {
case 1:
case 2:
case 3:
case 4:
case 5:
// Code for weekdays
break;
case 6:
case 7:
// Code for weekends
break;
default:
// Default code
}
- Enums or Integral Types:
switch
is often used with integral types (int, char) or enums, where the values being tested are discrete and limited.
C
enum Days { MON, TUE, WED, THU, FRI, SAT, SUN };
switch (day) {
case MON:
case TUE:
case WED:
case THU:
case FRI:
// Code for weekdays
break;
case SAT:
case SUN:
// Code for weekends
break;
default:
// Default code
}
In summary, both if-else
and switch
are essential tools in C for making decisions in your code. The choice between them depends on the specific conditions, values, and requirements of your program. Use if-else
when you have complex conditions or need to handle non-constant values, and use switch
when you have a single value to test against multiple constants, especially when some cases should fall through to execute the same code.