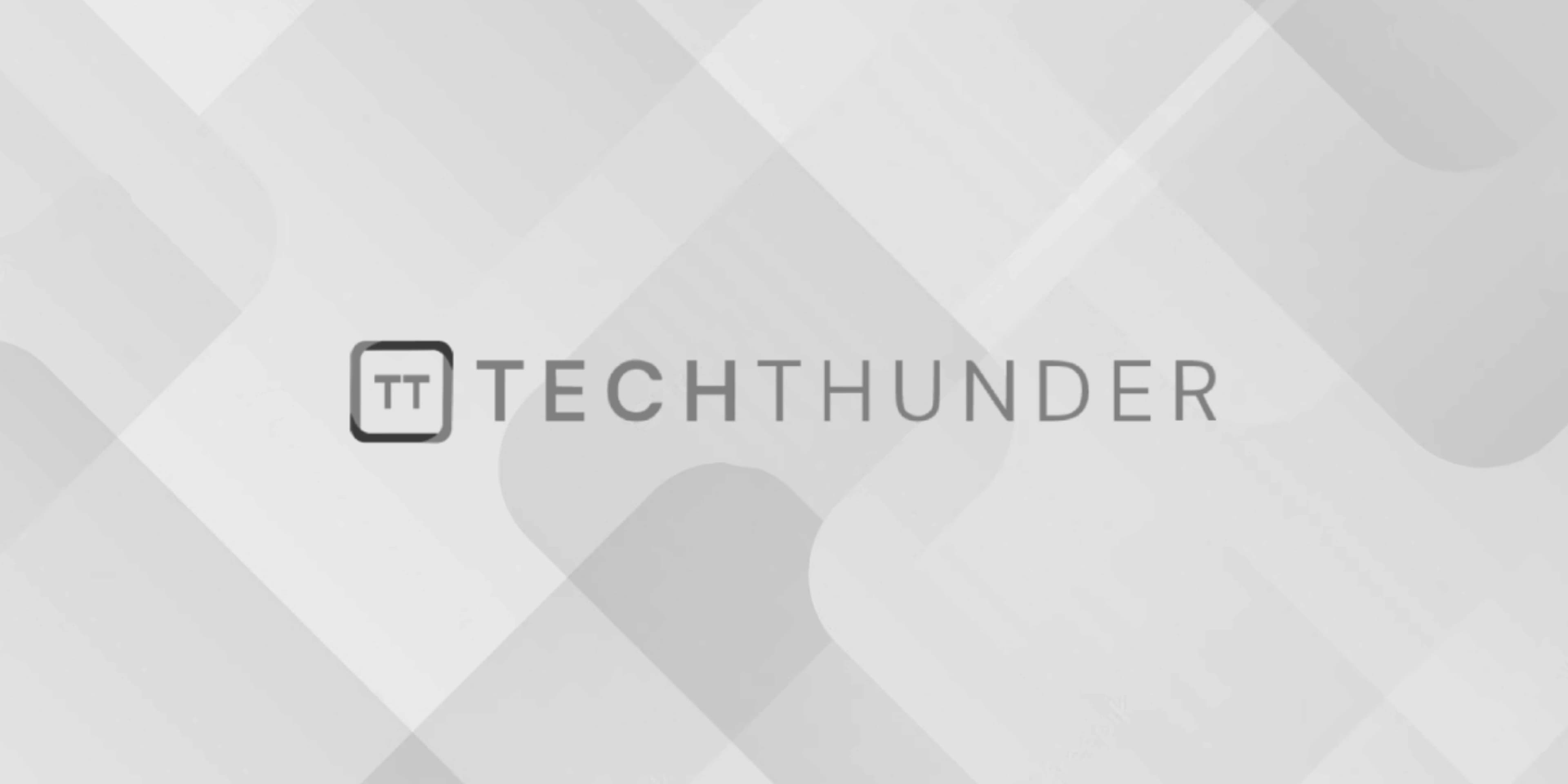
393 views
itoa Function in C
The itoa
function is not a standard C library function. However, it is available in some C libraries as a non-standard extension. The itoa
function is used to convert an integer to a string. If your C library does not provide itoa
, you can use the standard sprintf
function to achieve the same result.
Here’s an example of how to use sprintf
to convert an integer to a string in C:
C
#include <stdio.h>
int main() {
int num = 12345;
char str[20]; // Make sure the array is large enough to hold the string representation
sprintf(str, "%d", num);
printf("Integer: %d\nString: %s\n", num, str);
return 0;
}
In this example:
- We have an integer
num
with the value 12345. - We declare a character array
str
to hold the string representation of the integer. Make sure the array is large enough to accommodate the string representation. - We use the
sprintf
function to format the integer as a string and store it in thestr
array. The%d
format specifier is used to convert the integer to a string. - Finally, we print both the original integer and the string representation.
Keep in mind that sprintf
can be used for various formatting tasks, including converting integers to strings, but it should be used with caution to avoid buffer overflows. Ensure that the destination buffer (str
in this case) is large enough to hold the resulting string to prevent undefined behavior.