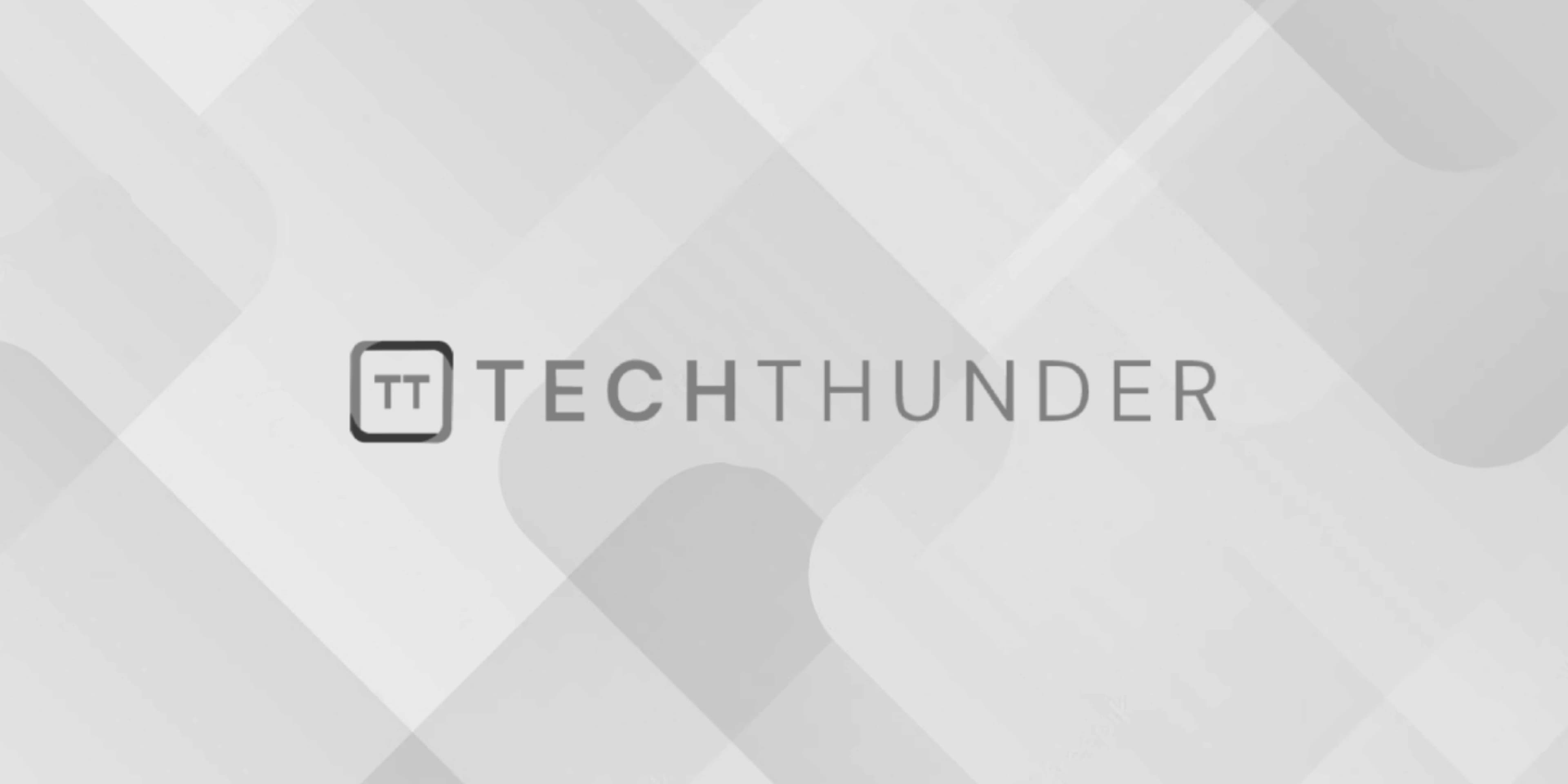
226 views
Library Management System in C
Creating a complete library management system in C is a complex task that involves multiple components, including managing books, library members, borrowing and returning books, and maintaining records. Here, I’ll provide you with a simplified outline and code structure to get you started on a basic library management system. This example does not include extensive error handling or data storage (e.g., using files or databases) for simplicity.
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// Structure to represent a book
struct Book {
int id;
char title[100];
char author[100];
int available;
};
// Structure to represent a library member
struct Member {
int id;
char name[100];
};
// Function to add a book to the library
void addBook(struct Book books[], int *bookCount) {
if (*bookCount >= MAX_BOOKS) {
printf("Library is full. Cannot add more books.\n");
return;
}
struct Book newBook;
newBook.id = *bookCount + 1;
printf("Enter Book Title: ");
scanf("%s", newBook.title);
printf("Enter Author: ");
scanf("%s", newBook.author);
newBook.available = 1; // Initially, the book is available
books[*bookCount] = newBook;
(*bookCount)++;
printf("Book added successfully.\n");
}
// Function to display all books in the library
void displayBooks(struct Book books[], int bookCount) {
if (bookCount == 0) {
printf("No books available in the library.\n");
return;
}
printf("ID\tTitle\tAuthor\tAvailable\n");
for (int i = 0; i < bookCount; i++) {
printf("%d\t%s\t%s\t%s\n", books[i].id, books[i].title, books[i].author,
books[i].available ? "Yes" : "No");
}
}
// Function to lend a book to a library member
void lendBook(struct Book books[], int bookCount, struct Member members[], int memberCount) {
// Implement lending logic here
}
// Function to return a book to the library
void returnBook(struct Book books[], int bookCount) {
// Implement return logic here
}
int main() {
struct Book books[MAX_BOOKS];
struct Member members[MAX_MEMBERS];
int bookCount = 0;
int memberCount = 0;
int choice;
while (1) {
printf("\nLibrary Management System Menu:\n");
printf("1. Add Book\n");
printf("2. Display Books\n");
printf("3. Lend Book\n");
printf("4. Return Book\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addBook(books, &bookCount);
break;
case 2:
displayBooks(books, bookCount);
break;
case 3:
lendBook(books, bookCount, members, memberCount);
break;
case 4:
returnBook(books, bookCount);
break;
case 5:
exit(0);
default:
printf("Invalid choice. Please try again.\n");
}
}
return 0;
}
This program allows you to input two matrices, perform addition, subtraction, multiplication, transposition, and display the result. You can expand this program by adding error handling, dynamic memory allocation, and more matrix operations as needed.