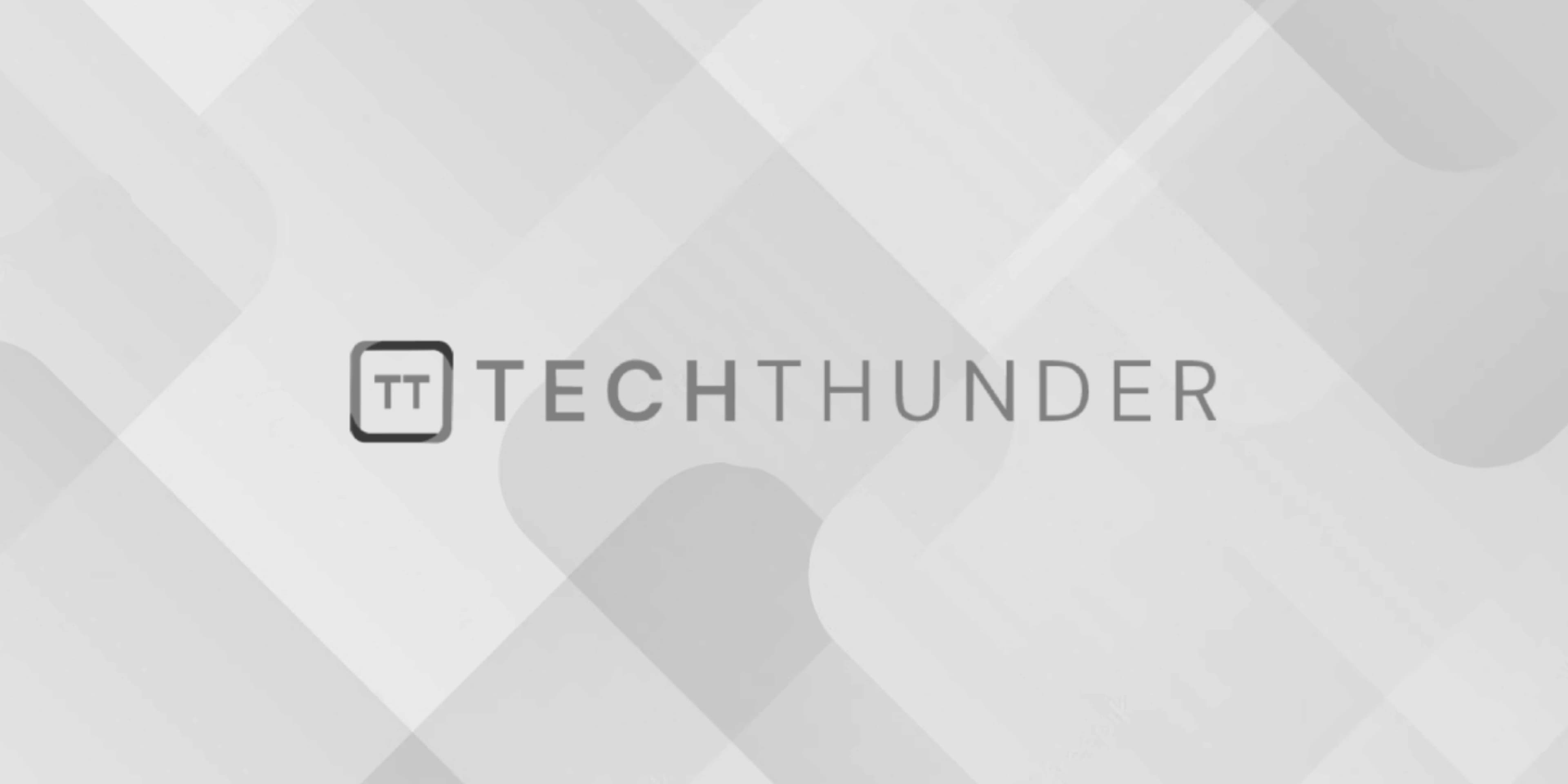
234 views
Calculator Program in C
Creating a basic calculator program in C is a common beginner’s exercise and a good way to practice arithmetic operations and user input handling. Below is a simple example of a calculator program that can perform addition, subtraction, multiplication, and division operations based on user input. It uses a switch
statement to select the operation.
C
#include <stdio.h>
int main() {
char operator;
double num1, num2, result;
// Input from the user
printf("Enter an operator (+, -, *, /): ");
scanf(" %c", &operator);
printf("Enter two numbers: ");
scanf("%lf %lf", &num1, &num2);
// Perform the requested operation
switch (operator) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
if (num2 != 0) {
result = num1 / num2;
} else {
printf("Error: Division by zero is not allowed.\n");
return 1; // Exit with an error code
}
break;
default:
printf("Error: Invalid operator.\n");
return 1; // Exit with an error code
}
// Display the result
printf("Result: %lf\n", result);
return 0;
}
In this program:
- We declare variables to store the operator, two numbers, and the result.
- We prompt the user to enter an operator (+, -, *, /) and two numbers.
- We use a
switch
statement to determine which operation to perform based on the entered operator. - We perform the calculation and handle division by zero errors if necessary.
- Finally, we display the result.
Compile and run this program, and it will allow you to perform basic arithmetic calculations.