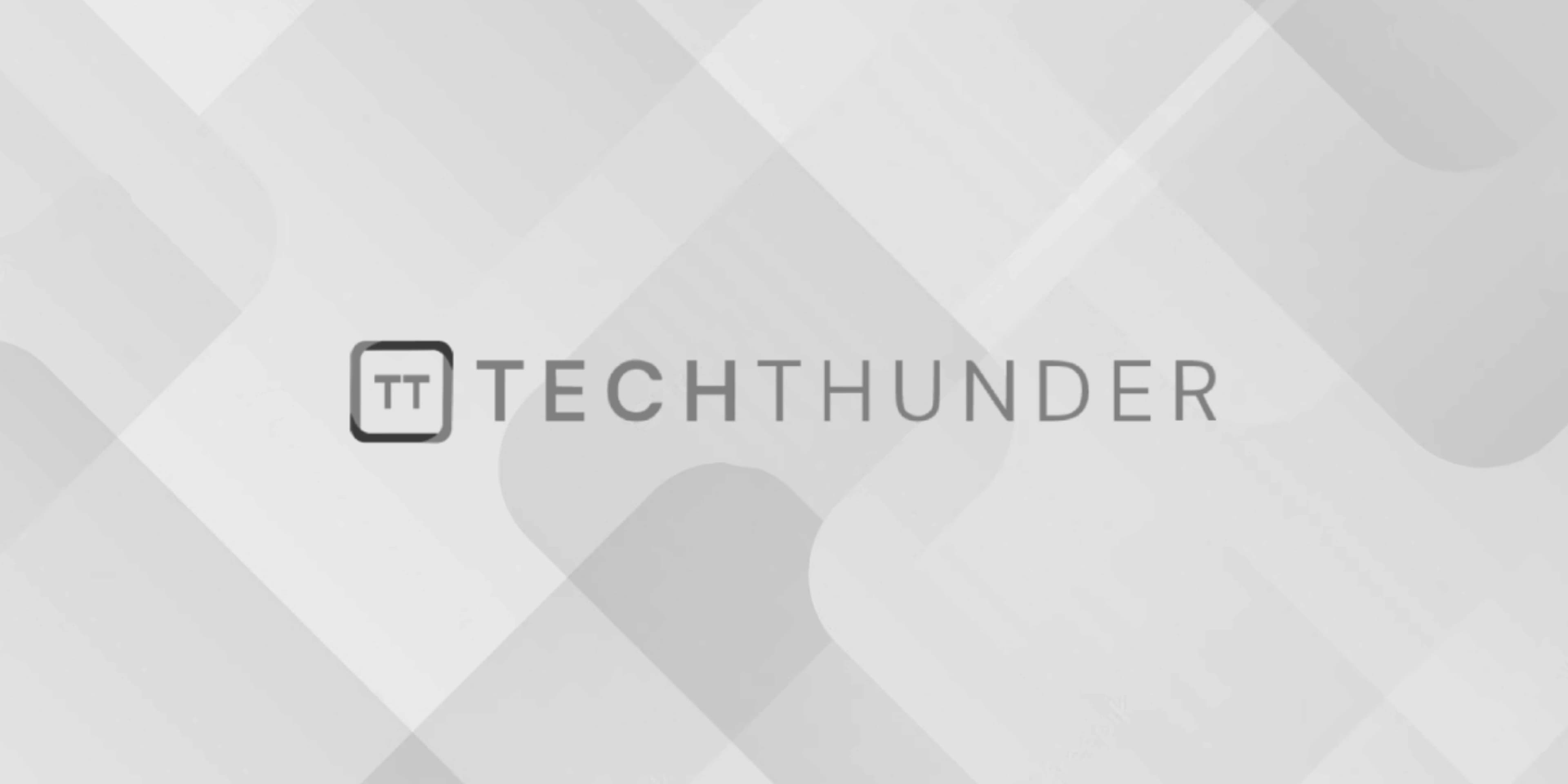
C strcat()
The strcat()
is a standard library function used to concatenate (append) one string to the end of another string. It’s part of the <string.h>
header and is commonly used for string manipulation in C programs. strcat()
appends the characters of the source string to the end of the destination string and then adds a null character (‘\0’) to terminate the concatenated string.
Here’s the syntax of strcat()
:
char *strcat(char *destination, const char *source);
destination
: A pointer to the destination string, which will receive the appended characters.source
: A pointer to the source string, which will be appended to the destination string.
The strcat()
function appends the characters from the source
string to the end of the destination
string until it reaches the null character in the source
string. It then adds a null character at the end of the concatenated string, ensuring it is null-terminated.
Here’s an example of using strcat()
:
#include <stdio.h>
#include <string.h>
int main() {
char destination[20] = "Hello, ";
const char source[] = "World!";
strcat(destination, source);
printf("Concatenated string: %s\n", destination);
return 0;
}
In this example, we declare a destination
string and a source
string. We use strcat()
to concatenate (append) the source
string to the end of the destination
string, resulting in a single string: “Hello, World!”
It’s important to ensure that the destination
string has enough space to accommodate the appended characters from the source
string, including the null character. Failing to allocate enough space can lead to buffer overflow issues.
Additionally, like strcpy()
, you should be cautious when working with user-input or untrusted data, as strcat()
does not perform bounds checking and can result in buffer overflows if the destination buffer is not large enough to hold the concatenated string. Consider using safer functions like strncat()
or strcat_s()
(if available) when working with strings of unknown or potentially large lengths to prevent buffer overflows.