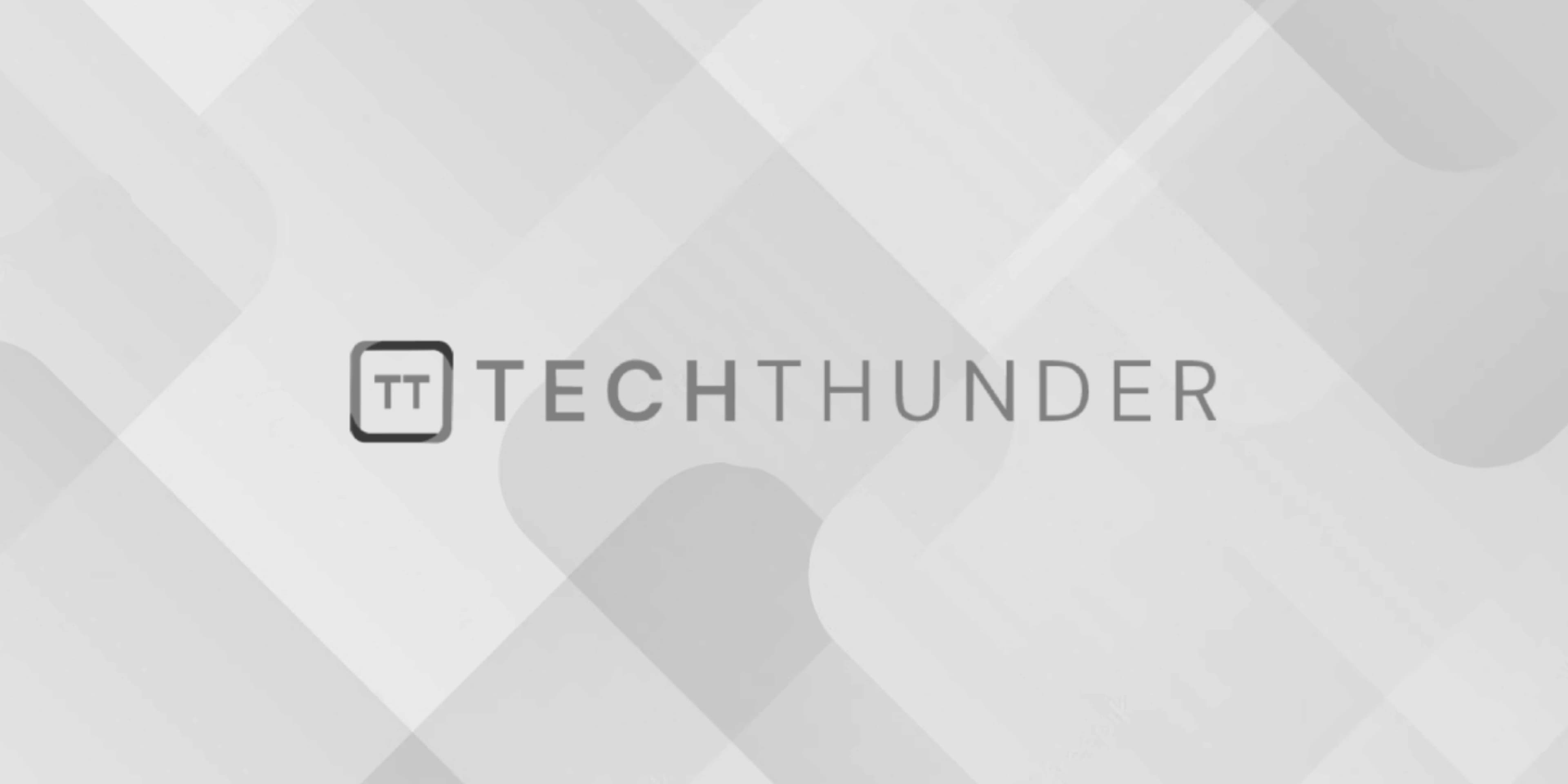
182 views
What is the main in C
The main
function is a special function that serves as the entry point for a C program. When a C program is executed, the operating system starts by calling the main
function, which is the starting point of program execution. The main
function is defined as follows:
C
int main() {
// Program code goes here
return 0; // Optionally, return an integer value to indicate program status
}
Here’s what you need to know about the main
function in C:
- Function Signature: The
main
function is defined with the return typeint
. This return type indicates the exit status of the program. By convention, a return value of0
usually indicates that the program ran successfully, while non-zero values can be used to indicate errors or other status conditions. - Arguments: The
main
function can also accept command-line arguments, although they are not required. The arguments can be accessed using theargc
(argument count) andargv
(argument vector) parameters, as follows:
C
int main(int argc, char *argv[]) {
// Program code that uses argc and argv
return 0;
}
argc
: An integer that holds the number of command-line arguments passed to the program, including the program name itself.argv
: An array of strings (character pointers) containing the command-line arguments.
- Execution: The code inside the
main
function is executed sequentially, starting from the opening curly brace{
and ending with the closing curly brace}
. This is where you write the logic for your program. - Return Value: The
main
function can return an integer value using thereturn
statement. This value is typically used to indicate the success or failure of the program to the calling environment. A return value of0
often indicates successful execution, while non-zero values can represent errors or specific status conditions. - Execution Flow: When the
main
function reaches thereturn
statement, or when the closing brace}
of themain
function is reached, the program terminates, and control is returned to the operating system. The return value ofmain
is used as the exit status code of the program.
Here’s a simple example of a C program with the main
function:
C
#include <stdio.h>
int main() {
printf("Hello, world!\n");
return 0; // Indicates successful execution
}
In this example, the main
function is responsible for printing “Hello, world!” to the console and returning 0
to indicate successful execution. The program starts by calling main
, executes the code within it, and then exits with a status code of 0
.