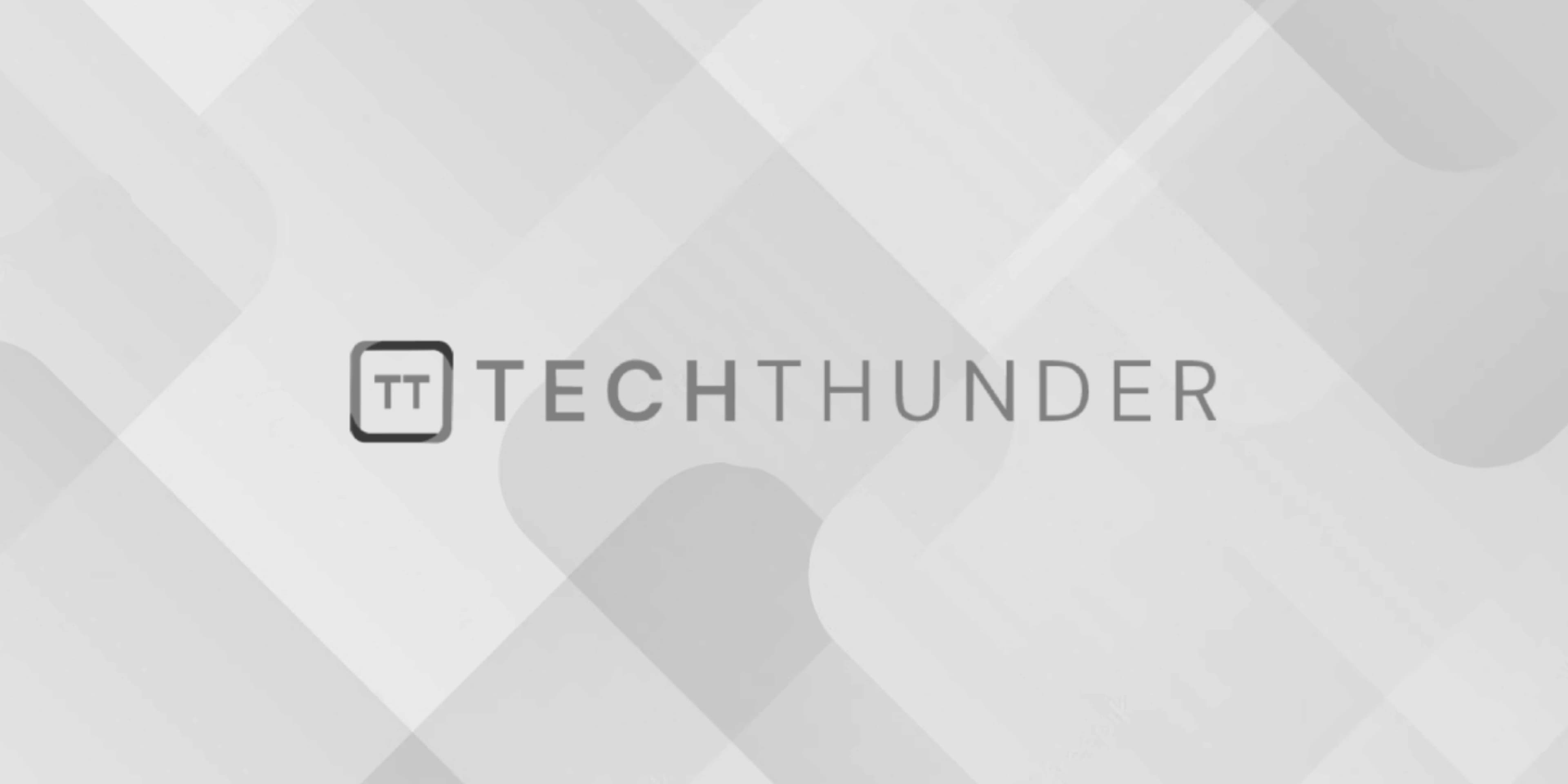
Logical AND Operator in C
The logical AND operator, denoted by &&
, is a binary operator that combines two boolean expressions and returns a result based on their logical conjunction. It’s used to determine whether both of its operands are true (non-zero) or not.
Here’s the syntax for the logical AND operator:
expression1 && expression2
expression1
andexpression2
are the boolean expressions you want to combine.
The logical AND operator works as follows:
- If both
expression1
andexpression2
are true (non-zero), the result is true (1). - If either
expression1
orexpression2
is false (zero), the result is false (0).
Here’s an example of how the logical AND operator is used in C:
#include <stdio.h>
int main() {
int a = 5;
int b = 10;
if (a > 0 && b > 0) {
printf("Both 'a' and 'b' are greater than 0.\n");
} else {
printf("At least one of 'a' and 'b' is not greater than 0.\n");
}
return 0;
}
In this example, the logical AND operator is used to check if both a
and b
are greater than 0. If both conditions are true, it prints a message indicating that both variables are greater than 0; otherwise, it prints a message indicating that at least one of them is not greater than 0.
Output (in this case):
Both 'a' and 'b' are greater than 0.
The logical AND operator is often used in conditional statements and boolean expressions to make decisions based on multiple conditions. It’s an essential part of C’s control flow and decision-making capabilities.