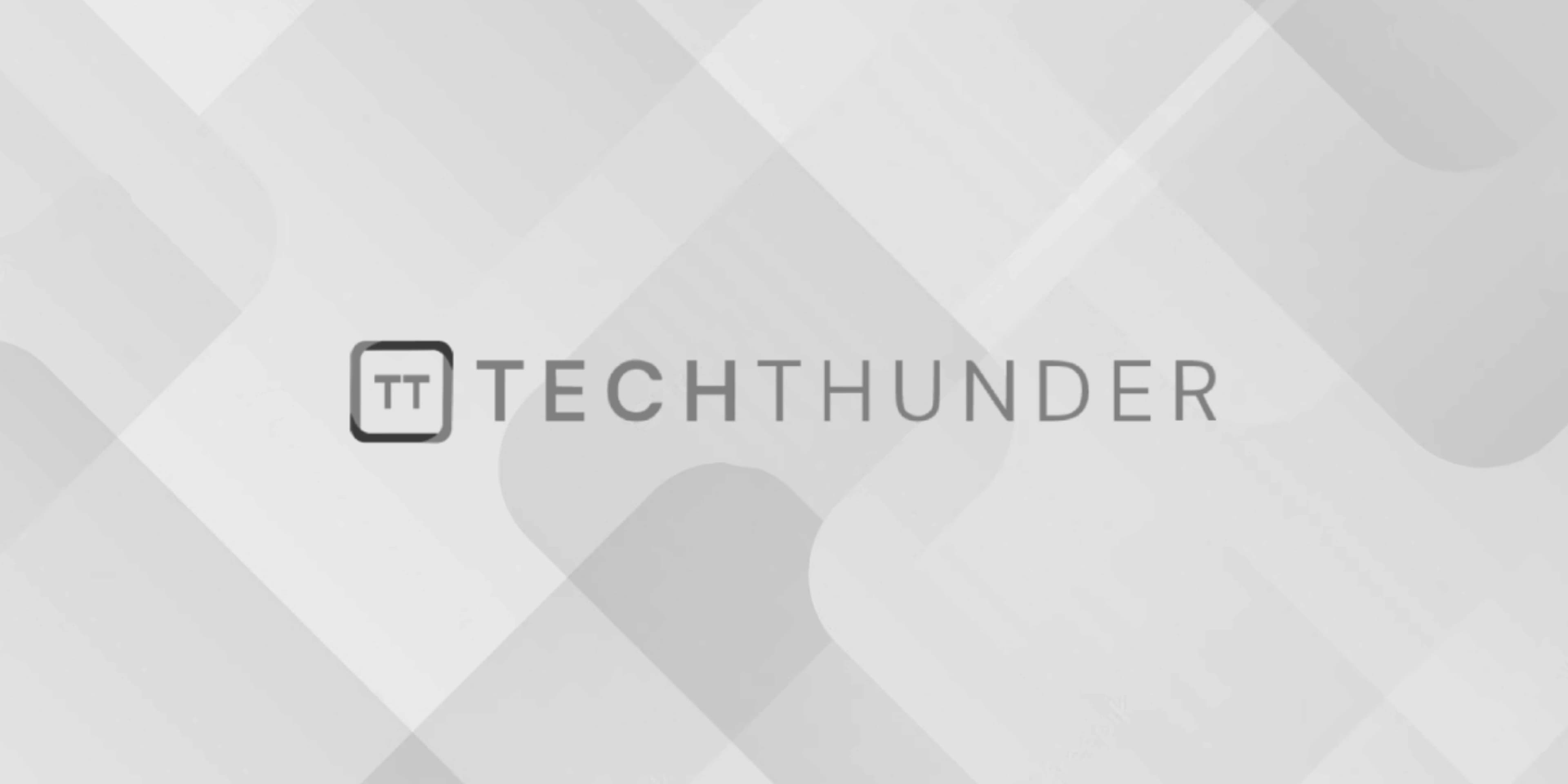
330 views
printf scanf
The printf
and scanf
are two important functions for input and output operations. They are part of the standard I/O library (stdio.h
) and are commonly used for displaying output and reading input, respectively.
printf:
printf
is a function used for formatted output in C.- It allows you to display text, numbers, and other data on the console or other output devices.
- The function takes a format string as its first argument, followed by one or more additional arguments that provide values to be formatted and inserted into the format string.
- Format specifiers, such as
%d
,%f
,%s
, etc., are used in the format string to specify the type and position of the values to be displayed. - Here’s a simple example:
C
#include <stdio.h>
int main() {
int num = 42;
printf("The value of num is %d\n", num);
return 0;
}
Output:
Plaintext
The value of num is 42
scanf:
scanf
is a function used for formatted input in C.- It allows you to read data from the standard input (usually the keyboard) and store it in variables.
- Like
printf
,scanf
also uses format specifiers to specify the expected type of input data. - It takes a format string as its first argument, followed by pointers to variables where the input data will be stored.
- Here’s an example of using
scanf
to read an integer:
C
#include <stdio.h>
int main() {
int num;
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered: %d\n", num);
return 0;
}
In this example, %d
in the scanf
format string indicates that an integer should be read from the input. &num
is used to store the input value in the num
variable.
Both printf
and scanf
are powerful tools for handling input and output in C programs, and they are commonly used in a wide range of applications. Properly using format specifiers is crucial to ensure that the data is read or displayed correctly. Additionally, error handling should be considered when using scanf
to handle unexpected input situations.