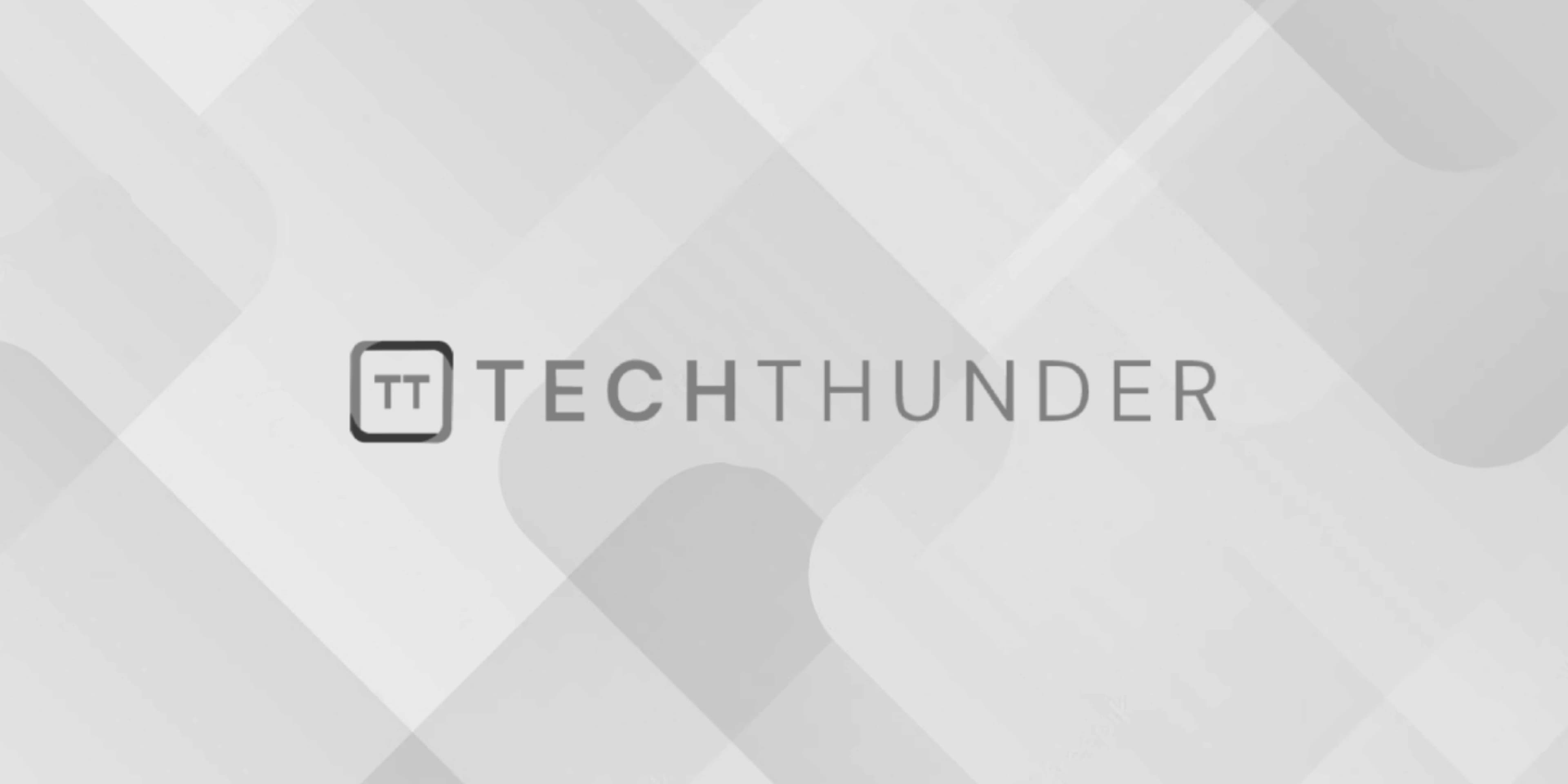
354 views
Employee Record System in C
Creating an employee record system in C involves managing employee data, including details such as name, ID, salary, and other relevant information. Here’s a basic implementation of an employee record system in C:
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_EMPLOYEES 100
// Structure to represent an employee
struct Employee {
int id;
char name[100];
double salary;
};
// Function to add a new employee
void addEmployee(struct Employee employees[], int *employeeCount) {
if (*employeeCount >= MAX_EMPLOYEES) {
printf("Employee database is full. Cannot add more employees.\n");
return;
}
struct Employee newEmployee;
printf("Enter Employee ID: ");
scanf("%d", &newEmployee.id);
printf("Enter Employee Name: ");
scanf("%s", newEmployee.name);
printf("Enter Employee Salary: ");
scanf("%lf", &newEmployee.salary);
employees[*employeeCount] = newEmployee;
(*employeeCount)++;
printf("Employee added successfully.\n");
}
// Function to display all employees
void displayEmployees(struct Employee employees[], int employeeCount) {
if (employeeCount == 0) {
printf("No employees to display.\n");
return;
}
printf("Employee ID\tName\tSalary\n");
for (int i = 0; i < employeeCount; i++) {
printf("%d\t\t%s\t%.2lf\n", employees[i].id, employees[i].name, employees[i].salary);
}
}
int main() {
struct Employee employees[MAX_EMPLOYEES];
int employeeCount = 0;
int choice;
while (1) {
printf("\nEmployee Record System Menu:\n");
printf("1. Add Employee\n");
printf("2. Display Employees\n");
printf("3. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addEmployee(employees, &employeeCount);
break;
case 2:
displayEmployees(employees, employeeCount);
break;
case 3:
exit(0);
default:
printf("Invalid choice. Please try again.\n");
}
}
return 0;
}
In this code, we’ve created a simple employee record system with the following features:
- You can add new employees, including their ID, name, and salary.
- You can display the details of all employees in the system.
- The system uses an array of structures (
struct Employee
) to store employee data. - The maximum number of employees is defined by
MAX_EMPLOYEES
. - The program provides a menu to interact with the system, allowing you to add employees, display employee records, and exit the program.
This is a basic implementation for educational purposes. In a real-world scenario, you may want to enhance the system with error handling, data validation, the ability to edit or delete employee records, and data persistence (e.g., saving and loading employee data from files).