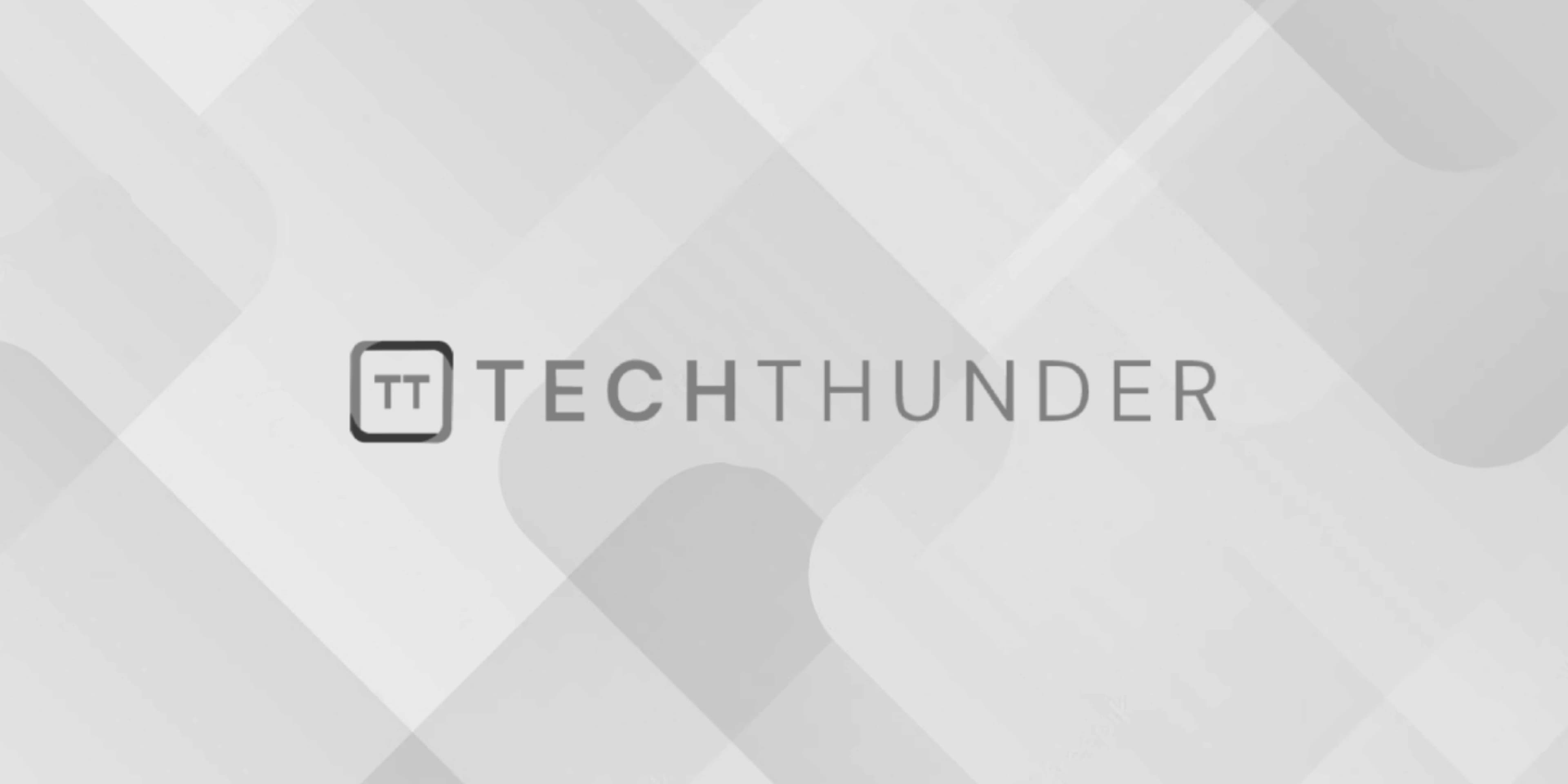
117 views
Number in Characters in C
To convert a number into its corresponding characters (digits) in C, you can use several methods, including using integer arithmetic and loops to extract individual digits. Here’s a simple C program that demonstrates how to convert an integer into a string of characters:
C
#include <stdio.h>
#include <stdlib.h>
int main() {
int num;
// Input: Enter an integer
printf("Enter an integer: ");
scanf("%d", &num);
// Determine the length of the number
int length = snprintf(NULL, 0, "%d", num);
// Allocate memory for the character array
char *numString = (char *)malloc(length + 1);
// Convert the integer to a string
snprintf(numString, length + 1, "%d", num);
// Print the result
printf("Number as characters: %s\n", numString);
// Free allocated memory
free(numString);
return 0;
}
In this program:
- We use
snprintf
twice to determine the length of the integer when converted to a string (length
) and to convert the integer into a string (numString
). - We allocate memory for the
numString
character array to store the characters of the number as a string. Thelength + 1
accounts for the null terminator. - Finally, we print the result and free the dynamically allocated memory to prevent memory leaks.
Compile and run the program. It will prompt you to enter an integer, and then it will convert the integer into characters and display them as a string.
Keep in mind that this program assumes that the input is a valid integer. Additional error checking may be necessary in a real-world application to handle invalid input.