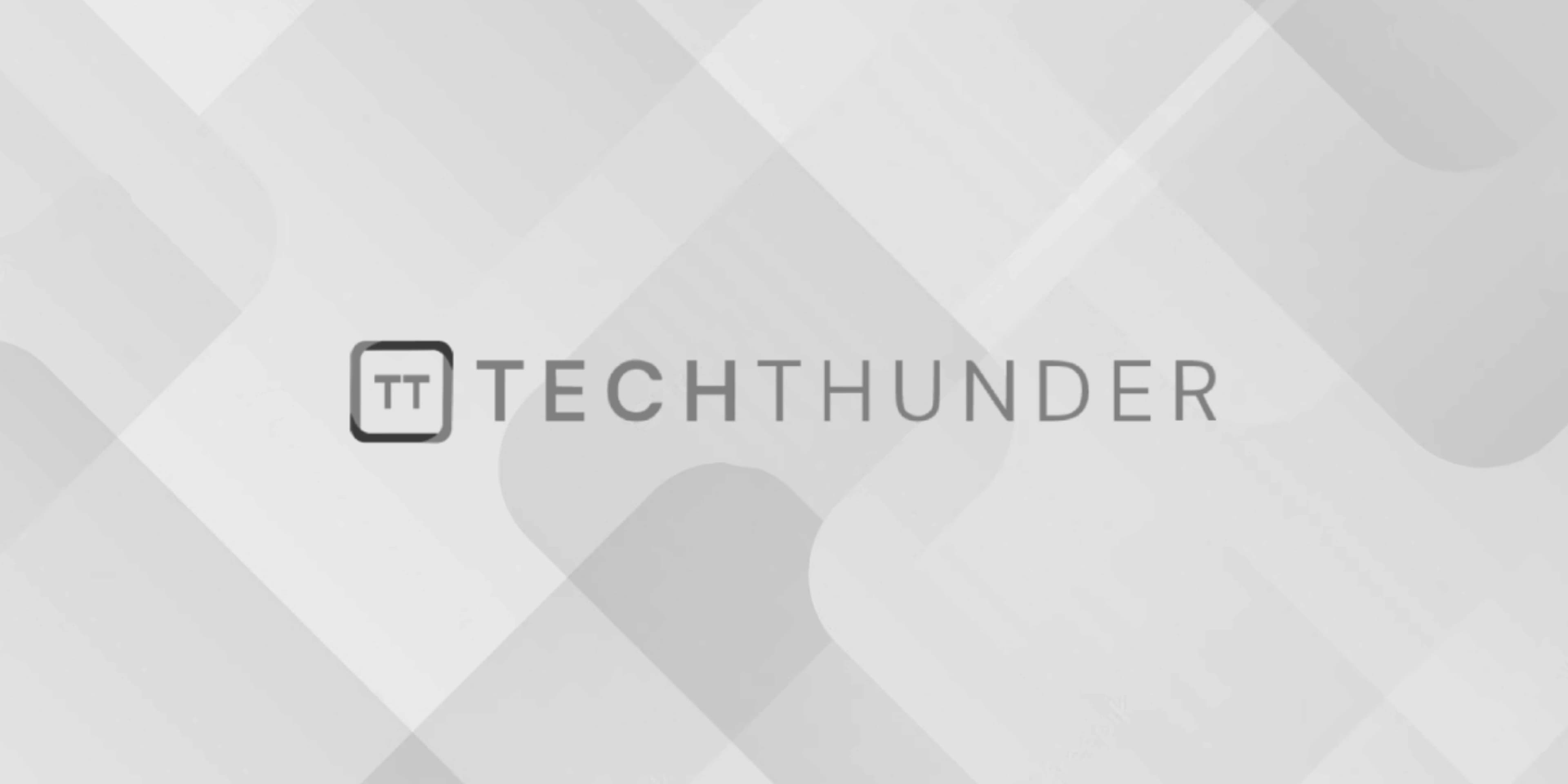
334 views
Prime Number in C
A prime number is a positive integer greater than 1 that has no positive integer divisors other than 1 and itself. To check if a number is prime in C, you can write a program that iterates through all the numbers from 2 to the square root of the number you want to check and checks for divisibility. If the number is divisible by any other number in that range, it’s not prime; otherwise, it’s prime. Here’s a C program to determine if a number is prime:
C
#include <stdio.h>
#include <math.h>
int isPrime(int num) {
if (num <= 1) {
return 0; // 0 and 1 are not prime
}
if (num == 2) {
return 1; // 2 is prime
}
if (num % 2 == 0) {
return 0; // Even numbers other than 2 are not prime
}
// Check for divisibility from 3 to the square root of num
for (int i = 3; i <= sqrt(num); i += 2) {
if (num % i == 0) {
return 0; // Not prime
}
}
return 1; // Prime
}
int main() {
int num;
printf("Enter a positive integer: ");
scanf("%d", &num);
if (isPrime(num)) {
printf("%d is a prime number.\n", num);
} else {
printf("%d is not a prime number.\n", num);
}
return 0;
}
In this program:
- The
isPrime
function takes an integernum
as input and returns 1 ifnum
is prime and 0 if it’s not prime. - We handle some special cases first: 0 and 1 are not prime, 2 is prime, and even numbers greater than 2 are not prime.
- We then iterate through odd numbers from 3 to the square root of
num
. We only check odd numbers because even numbers greater than 2 are already excluded as mentioned earlier. Ifnum
is divisible by any of these numbers, it’s not prime. - Finally, we call the
isPrime
function in themain
function to check if the entered number is prime and display the result.
Compile and run the program, and it will determine if the entered integer is prime or not.