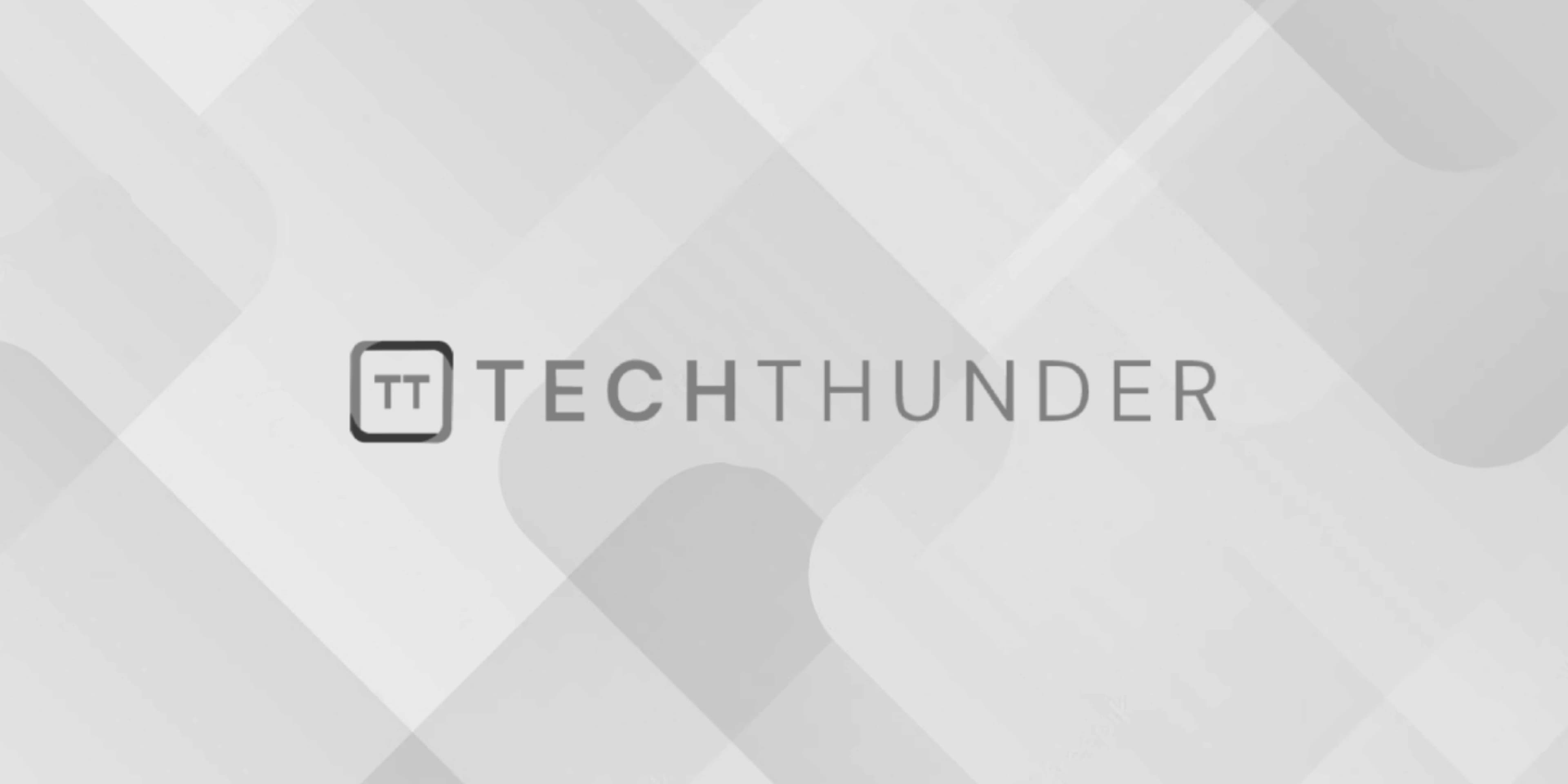
Const Qualifier in C
The const
qualifier is used to declare variables as constants, meaning that their values cannot be modified after initialization. The const
qualifier is part of the type of the variable and can be applied to various types, including variables, pointers, and function parameters.
Here are some common use cases of the const
qualifier:
- Constant Variables:
const int x = 10;
In this example, x
is a constant integer, and you cannot change its value after initialization. Attempting to modify it will result in a compilation error.
- Constant Pointers:
int y = 20;
const int *ptr = &y;
Here, ptr
is a pointer to a constant integer. This means that you can change the pointer itself (i.e., make it point to a different integer), but you cannot modify the value it points to (i.e., you cannot do *ptr = 30;
).
- Pointer to a Constant:
int z = 30;
int *const ptr = &z;
In this case, ptr
is a constant pointer to an integer. You can modify the value it points to (e.g., *ptr = 40;
), but you cannot change the pointer itself to point to a different integer.
- Constant Function Parameters:
void foo(const int param) {
// Cannot modify param within the function
}
Here, the param
parameter is declared as a constant integer, indicating that the function foo
cannot modify the value of param
.
The use of const
provides several benefits, including code clarity, prevention of unintended modifications, and potential optimizations by the compiler. It’s particularly useful for defining constants, making function interfaces more explicit, and indicating your intention not to modify certain values.
Keep in mind that the const
qualifier is a compile-time constraint. It does not necessarily guarantee that the underlying memory is read-only; it merely prevents modification through the declared variable or pointer. For truly read-only memory, you may need platform-specific or compiler-specific mechanisms like read-only data sections.